First commit
This commit is contained in:
commit
c560b33468
|
@ -0,0 +1,157 @@
|
|||
let selectedMenuItemId = null
|
||||
let selectedText = null
|
||||
let settings = {
|
||||
model: "text-davinci-003",
|
||||
temperature: 0.7,
|
||||
max_tokens: 256,
|
||||
top_p: 1,
|
||||
frequency_penalty: 0,
|
||||
presence_penalty: 0,
|
||||
api_key: "apikeyhere"
|
||||
}
|
||||
|
||||
const createContextMenu = (title) => {
|
||||
chrome.contextMenus.create({
|
||||
id: title.toLowerCase().split(" ").join("-"),
|
||||
title: title,
|
||||
contexts: ["browser_action", "selection"]
|
||||
})
|
||||
}
|
||||
|
||||
const generatePrompt = () => {
|
||||
let prompt
|
||||
switch (selectedMenuItemId) {
|
||||
case "explain-selection":
|
||||
prompt = "Explain: '" + selectedText + "'"
|
||||
break
|
||||
case "complete-selection":
|
||||
prompt = selectedText
|
||||
break
|
||||
case "respond-to-selection":
|
||||
prompt = "Respond to this message: '" + selectedText + "'"
|
||||
break
|
||||
case "summerize-selection":
|
||||
prompt = "Summerize: '" + selectedText + "'"
|
||||
break
|
||||
case "translate-selection":
|
||||
// TODO: Add language selection to the settings on the popup.js file.
|
||||
prompt = "Translate into english: '" + selectedText + "'"
|
||||
break
|
||||
default:
|
||||
prompt = selectedText
|
||||
break
|
||||
}
|
||||
return prompt
|
||||
}
|
||||
|
||||
const sendTabMessage = (message) => {
|
||||
chrome.tabs.query({ active: true, currentWindow: true }, function (tabs) {
|
||||
if (tabs[0]) {
|
||||
chrome.tabs.sendMessage(tabs[0].id, message)
|
||||
}
|
||||
})
|
||||
}
|
||||
|
||||
const generateRequestBody = (prompt) => {
|
||||
return JSON.stringify({ ...Object.fromEntries(Object.entries(settings).filter(([key]) => key != "api_key")), ...{ prompt: prompt } })
|
||||
}
|
||||
|
||||
const handleAPIResponse = (json) => {
|
||||
let apiResult = json.choices[0].text
|
||||
|
||||
if (selectedMenuItemId == null) {
|
||||
// send to popup.js
|
||||
chrome.runtime.sendMessage({
|
||||
type: 'prompt-response',
|
||||
data: apiResult
|
||||
})
|
||||
} else {
|
||||
// send to content.js
|
||||
sendTabMessage({ apiResult: apiResult })
|
||||
}
|
||||
}
|
||||
|
||||
const handleAPIError = (error) => {
|
||||
sendTabMessage({ apiResult: "Error. Please retry." })
|
||||
}
|
||||
|
||||
const executeAPICall = (prompt) => {
|
||||
fetch("https://api.openai.com/v1/completions", {
|
||||
method: "POST",
|
||||
headers: {
|
||||
"Content-Type": "application/json",
|
||||
"Authorization": "Bearer " + settings.api_key
|
||||
},
|
||||
body: generateRequestBody(prompt)
|
||||
})
|
||||
.then(response => response.json())
|
||||
.then(handleAPIResponse).catch(handleAPIError)
|
||||
}
|
||||
|
||||
const setLoading = () => {
|
||||
if (selectedMenuItemId) {
|
||||
sendTabMessage({ apiResult: "loading" })
|
||||
}
|
||||
}
|
||||
|
||||
const requestAPIResponse = () => {
|
||||
if (!selectedText) {
|
||||
return
|
||||
}
|
||||
|
||||
setLoading()
|
||||
executeAPICall(generatePrompt())
|
||||
}
|
||||
|
||||
const handleContextMenuClick = (info, tab) => {
|
||||
selectedMenuItemId = info.menuItemId
|
||||
requestAPIResponse()
|
||||
}
|
||||
|
||||
const handleSettingsLoad = (storageResult) => {
|
||||
if (storageResult.settings) {
|
||||
setSettings(storageResult.settings)
|
||||
}
|
||||
}
|
||||
|
||||
const setSettings = (newSettings) => {
|
||||
settings = {
|
||||
model: newSettings.model,
|
||||
temperature: parseFloat(newSettings.temperature),
|
||||
max_tokens: parseInt(newSettings.max_tokens),
|
||||
top_p: parseFloat(newSettings.top_p),
|
||||
frequency_penalty: parseFloat(newSettings.frequency_penalty),
|
||||
presence_penalty: parseFloat(newSettings.presence_penalty),
|
||||
api_key: newSettings.api_key
|
||||
}
|
||||
}
|
||||
|
||||
const handleMessage = (message, sender, sendResponse) => {
|
||||
// TODO: Use a message type
|
||||
if (message.hasOwnProperty("selectedText")) {
|
||||
selectedText = message.selectedText
|
||||
if (!selectedText || selectedText === "") {
|
||||
sendTabMessage({ removeTooltip: true })
|
||||
}
|
||||
} else if (message.hasOwnProperty("model")) {
|
||||
setSettings(message)
|
||||
} else if (message.hasOwnProperty("retry")) {
|
||||
requestAPIResponse()
|
||||
} else if (message.hasOwnProperty("prompt")) {
|
||||
selectedText = message.prompt
|
||||
selectedMenuItemId = null
|
||||
requestAPIResponse()
|
||||
}
|
||||
}
|
||||
|
||||
createContextMenu("Explain Selection")
|
||||
createContextMenu("Complete Selection")
|
||||
createContextMenu("Respond to Selection")
|
||||
createContextMenu("Summerize Selection")
|
||||
createContextMenu("Translate Selection")
|
||||
|
||||
chrome.contextMenus.onClicked.addListener(handleContextMenuClick)
|
||||
|
||||
chrome.storage.sync.get("settings", handleSettingsLoad)
|
||||
|
||||
chrome.runtime.onMessage.addListener(handleMessage)
|
|
@ -0,0 +1,116 @@
|
|||
// Keep track of the current tooltip element
|
||||
let currentTooltip = null
|
||||
|
||||
const removeTooltip = () => {
|
||||
// Remove the current tooltip element, if there is one
|
||||
if (currentTooltip) {
|
||||
document.body.removeChild(currentTooltip)
|
||||
currentTooltip = null
|
||||
}
|
||||
}
|
||||
|
||||
const addLoadingIcon = (tooltip) => {
|
||||
// Create a loading element
|
||||
let loadingElement = document.createElement("div")
|
||||
loadingElement.classList.add("loading-ai-tooltip")
|
||||
|
||||
// Add a spinner animation to the loading element
|
||||
let spinner = document.createElement("div")
|
||||
spinner.classList.add("spinner-ai-tooltip")
|
||||
loadingElement.appendChild(spinner)
|
||||
|
||||
// Add the loading element to the tooltip
|
||||
tooltip.appendChild(loadingElement)
|
||||
}
|
||||
|
||||
const handleRetryButtonClick = () => {
|
||||
chrome.runtime.sendMessage({ retry: true })
|
||||
}
|
||||
|
||||
|
||||
const createButton = (text, callback) => {
|
||||
let button = document.createElement("button")
|
||||
button.innerText = text
|
||||
button.onclick = callback
|
||||
return button
|
||||
}
|
||||
|
||||
const addButtons = (tooltip, apiResult) => {
|
||||
let retryButton = createButton("Retry", handleRetryButtonClick)
|
||||
let copyButton = createButton("Copy", () => { navigator.clipboard.writeText(apiResult) })
|
||||
|
||||
tooltip.appendChild(copyButton)
|
||||
tooltip.appendChild(retryButton)
|
||||
}
|
||||
|
||||
const addTooltip = (apiResult) => {
|
||||
let tooltip = document.createElement("div")
|
||||
tooltip.classList.add("ai-tooltip")
|
||||
|
||||
if (apiResult == 'loading') {
|
||||
addLoadingIcon(tooltip)
|
||||
} else {
|
||||
tooltip.innerText = apiResult
|
||||
addButtons(tooltip, apiResult)
|
||||
}
|
||||
|
||||
document.body.appendChild(tooltip)
|
||||
currentTooltip = tooltip
|
||||
adjustTooltipPosition()
|
||||
}
|
||||
|
||||
const adjustTooltipPosition = () => {
|
||||
if (!currentTooltip) {
|
||||
return
|
||||
}
|
||||
|
||||
let rect = window.getSelection().getRangeAt(0).getBoundingClientRect()
|
||||
currentTooltip.style.cssText = "top: " + rect.bottom + "px !important; left: " + rect.left + "px !important;"
|
||||
}
|
||||
|
||||
const handleAPIResult = (apiResult) => {
|
||||
removeTooltip()
|
||||
addTooltip(apiResult)
|
||||
}
|
||||
|
||||
const messageHandler = (message) => {
|
||||
if (message.hasOwnProperty("removeTooltip")) {
|
||||
removeTooltip()
|
||||
} else {
|
||||
console.log(message)
|
||||
handleAPIResult(message.apiResult.trim())
|
||||
}
|
||||
}
|
||||
|
||||
const sendRuntimeMessage = () => {
|
||||
let selectedText = window.getSelection().toString()
|
||||
chrome.runtime.sendMessage({ selectedText: selectedText })
|
||||
}
|
||||
|
||||
const debounce = (func, wait) => {
|
||||
let timeout
|
||||
return (...args) => {
|
||||
const later = () => {
|
||||
timeout = null
|
||||
func(...args)
|
||||
}
|
||||
clearTimeout(timeout)
|
||||
timeout = setTimeout(later, wait)
|
||||
}
|
||||
}
|
||||
|
||||
const handleScroll = () => {
|
||||
setTimeout(adjustTooltipPosition, 300)
|
||||
}
|
||||
|
||||
// Listen for messages from the background script
|
||||
chrome.runtime.onMessage.addListener(messageHandler)
|
||||
|
||||
// Create a debounced version of the event listener
|
||||
let debouncedSendRuntimeMessage = debounce(sendRuntimeMessage, 250)
|
||||
|
||||
// Add the debounced event listener
|
||||
document.addEventListener("selectionchange", debouncedSendRuntimeMessage)
|
||||
|
||||
// Fix the position of the tooltip when the page is scrolled
|
||||
window.addEventListener("wheel", handleScroll)
|
Binary file not shown.
After Width: | Height: | Size: 823 B |
Binary file not shown.
After Width: | Height: | Size: 1.8 KiB |
|
@ -0,0 +1,37 @@
|
|||
.loading-ai-tooltip {
|
||||
display: flex;
|
||||
align-items: center;
|
||||
justify-content: center;
|
||||
}
|
||||
|
||||
.spinner-ai-tooltip {
|
||||
width: 20px;
|
||||
height: 20px;
|
||||
border: 3px solid #ccc;
|
||||
border-radius: 50%;
|
||||
border-top-color: #333;
|
||||
animation: spinner-ai-tooltip 0.6s linear infinite;
|
||||
}
|
||||
|
||||
@keyframes spinner-ai-tooltip {
|
||||
100% {
|
||||
transform: rotate(360deg);
|
||||
}
|
||||
}
|
||||
|
||||
.ai-tooltip {
|
||||
all: initial;
|
||||
color: black !important;
|
||||
z-index: 99999;
|
||||
font-size: 14px !important;
|
||||
max-width: 500px !important;
|
||||
min-height: 30px;
|
||||
min-width: 50px;
|
||||
max-height: 200px !important;
|
||||
overflow-y: auto !important;
|
||||
position: fixed !important;
|
||||
background-color: lightyellow !important;
|
||||
border: 1px solid gray !important;
|
||||
padding: 5px !important;
|
||||
border-radius: 15px !important;
|
||||
}
|
File diff suppressed because it is too large
Load Diff
File diff suppressed because one or more lines are too long
File diff suppressed because one or more lines are too long
File diff suppressed because it is too large
Load Diff
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,49 @@
|
|||
{
|
||||
"manifest_version": 3,
|
||||
"name": "AI Browser Assistant",
|
||||
"description": "OpenAI powered Assistant",
|
||||
"version": "0.0.1",
|
||||
"permissions": [
|
||||
"storage",
|
||||
"contextMenus",
|
||||
"activeTab"
|
||||
],
|
||||
"host_permissions": [
|
||||
"https://api.openai.com/*",
|
||||
"<all_urls>"
|
||||
],
|
||||
"web_accessible_resources": [
|
||||
{
|
||||
"resources": [
|
||||
"fix.css"
|
||||
],
|
||||
"matches": [
|
||||
"<all_urls>"
|
||||
]
|
||||
}
|
||||
],
|
||||
"background": {
|
||||
"service_worker": "background.js"
|
||||
},
|
||||
"content_scripts": [
|
||||
{
|
||||
"matches": [
|
||||
"<all_urls>"
|
||||
],
|
||||
"js": [
|
||||
"content.js"
|
||||
],
|
||||
"css": [
|
||||
"fix.css"
|
||||
]
|
||||
}
|
||||
],
|
||||
"action": {
|
||||
"default_icon": "icon.png",
|
||||
"default_popup": "popup.html"
|
||||
},
|
||||
"icons": {
|
||||
"16": "favicon-16x16.png",
|
||||
"32": "favicon-32x32.png"
|
||||
}
|
||||
}
|
|
@ -0,0 +1 @@
|
|||
../mocha/bin/_mocha
|
|
@ -0,0 +1 @@
|
|||
../he/bin/he
|
|
@ -0,0 +1 @@
|
|||
../mkdirp/bin/cmd.js
|
|
@ -0,0 +1 @@
|
|||
../mocha/bin/mocha
|
|
@ -0,0 +1 @@
|
|||
../semver/bin/semver
|
|
@ -0,0 +1 @@
|
|||
../vscode/bin/install
|
|
@ -0,0 +1,475 @@
|
|||
{
|
||||
"name": "assistant",
|
||||
"version": "1.0.0",
|
||||
"lockfileVersion": 2,
|
||||
"requires": true,
|
||||
"packages": {
|
||||
"node_modules/@tootallnate/once": {
|
||||
"version": "1.1.2",
|
||||
"resolved": "https://registry.npmjs.org/@tootallnate/once/-/once-1.1.2.tgz",
|
||||
"integrity": "sha512-RbzJvlNzmRq5c3O09UipeuXno4tA1FE6ikOjxZK0tuxVv3412l64l5t1W5pj4+rJq9vpkm/kwiR07aZXnsKPxw==",
|
||||
"dev": true,
|
||||
"engines": {
|
||||
"node": ">= 6"
|
||||
}
|
||||
},
|
||||
"node_modules/agent-base": {
|
||||
"version": "6.0.2",
|
||||
"resolved": "https://registry.npmjs.org/agent-base/-/agent-base-6.0.2.tgz",
|
||||
"integrity": "sha512-RZNwNclF7+MS/8bDg70amg32dyeZGZxiDuQmZxKLAlQjr3jGyLx+4Kkk58UO7D2QdgFIQCovuSuZESne6RG6XQ==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"debug": "4"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 6.0.0"
|
||||
}
|
||||
},
|
||||
"node_modules/balanced-match": {
|
||||
"version": "1.0.2",
|
||||
"resolved": "https://registry.npmjs.org/balanced-match/-/balanced-match-1.0.2.tgz",
|
||||
"integrity": "sha512-3oSeUO0TMV67hN1AmbXsK4yaqU7tjiHlbxRDZOpH0KW9+CeX4bRAaX0Anxt0tx2MrpRpWwQaPwIlISEJhYU5Pw==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/brace-expansion": {
|
||||
"version": "1.1.11",
|
||||
"resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-1.1.11.tgz",
|
||||
"integrity": "sha512-iCuPHDFgrHX7H2vEI/5xpz07zSHB00TpugqhmYtVmMO6518mCuRMoOYFldEBl0g187ufozdaHgWKcYFb61qGiA==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"balanced-match": "^1.0.0",
|
||||
"concat-map": "0.0.1"
|
||||
}
|
||||
},
|
||||
"node_modules/browser-stdout": {
|
||||
"version": "1.3.1",
|
||||
"resolved": "https://registry.npmjs.org/browser-stdout/-/browser-stdout-1.3.1.tgz",
|
||||
"integrity": "sha512-qhAVI1+Av2X7qelOfAIYwXONood6XlZE/fXaBSmW/T5SzLAmCgzi+eiWE7fUvbHaeNBQH13UftjpXxsfLkMpgw==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/buffer-from": {
|
||||
"version": "1.1.2",
|
||||
"resolved": "https://registry.npmjs.org/buffer-from/-/buffer-from-1.1.2.tgz",
|
||||
"integrity": "sha512-E+XQCRwSbaaiChtv6k6Dwgc+bx+Bs6vuKJHHl5kox/BaKbhiXzqQOwK4cO22yElGp2OCmjwVhT3HmxgyPGnJfQ==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/commander": {
|
||||
"version": "2.15.1",
|
||||
"resolved": "https://registry.npmjs.org/commander/-/commander-2.15.1.tgz",
|
||||
"integrity": "sha512-VlfT9F3V0v+jr4yxPc5gg9s62/fIVWsd2Bk2iD435um1NlGMYdVCq+MjcXnhYq2icNOizHr1kK+5TI6H0Hy0ag==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/concat-map": {
|
||||
"version": "0.0.1",
|
||||
"resolved": "https://registry.npmjs.org/concat-map/-/concat-map-0.0.1.tgz",
|
||||
"integrity": "sha512-/Srv4dswyQNBfohGpz9o6Yb3Gz3SrUDqBH5rTuhGR7ahtlbYKnVxw2bCFMRljaA7EXHaXZ8wsHdodFvbkhKmqg==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/debug": {
|
||||
"version": "4.3.4",
|
||||
"resolved": "https://registry.npmjs.org/debug/-/debug-4.3.4.tgz",
|
||||
"integrity": "sha512-PRWFHuSU3eDtQJPvnNY7Jcket1j0t5OuOsFzPPzsekD52Zl8qUfFIPEiswXqIvHWGVHOgX+7G/vCNNhehwxfkQ==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"ms": "2.1.2"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=6.0"
|
||||
},
|
||||
"peerDependenciesMeta": {
|
||||
"supports-color": {
|
||||
"optional": true
|
||||
}
|
||||
}
|
||||
},
|
||||
"node_modules/diff": {
|
||||
"version": "3.5.0",
|
||||
"resolved": "https://registry.npmjs.org/diff/-/diff-3.5.0.tgz",
|
||||
"integrity": "sha512-A46qtFgd+g7pDZinpnwiRJtxbC1hpgf0uzP3iG89scHk0AUC7A1TGxf5OiiOUv/JMZR8GOt8hL900hV0bOy5xA==",
|
||||
"dev": true,
|
||||
"engines": {
|
||||
"node": ">=0.3.1"
|
||||
}
|
||||
},
|
||||
"node_modules/es6-promise": {
|
||||
"version": "4.2.8",
|
||||
"resolved": "https://registry.npmjs.org/es6-promise/-/es6-promise-4.2.8.tgz",
|
||||
"integrity": "sha512-HJDGx5daxeIvxdBxvG2cb9g4tEvwIk3i8+nhX0yGrYmZUzbkdg8QbDevheDB8gd0//uPj4c1EQua8Q+MViT0/w==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/es6-promisify": {
|
||||
"version": "5.0.0",
|
||||
"resolved": "https://registry.npmjs.org/es6-promisify/-/es6-promisify-5.0.0.tgz",
|
||||
"integrity": "sha512-C+d6UdsYDk0lMebHNR4S2NybQMMngAOnOwYBQjTOiv0MkoJMP0Myw2mgpDLBcpfCmRLxyFqYhS/CfOENq4SJhQ==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"es6-promise": "^4.0.3"
|
||||
}
|
||||
},
|
||||
"node_modules/escape-string-regexp": {
|
||||
"version": "1.0.5",
|
||||
"resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-1.0.5.tgz",
|
||||
"integrity": "sha512-vbRorB5FUQWvla16U8R/qgaFIya2qGzwDrNmCZuYKrbdSUMG6I1ZCGQRefkRVhuOkIGVne7BQ35DSfo1qvJqFg==",
|
||||
"dev": true,
|
||||
"engines": {
|
||||
"node": ">=0.8.0"
|
||||
}
|
||||
},
|
||||
"node_modules/fs.realpath": {
|
||||
"version": "1.0.0",
|
||||
"resolved": "https://registry.npmjs.org/fs.realpath/-/fs.realpath-1.0.0.tgz",
|
||||
"integrity": "sha512-OO0pH2lK6a0hZnAdau5ItzHPI6pUlvI7jMVnxUQRtw4owF2wk8lOSabtGDCTP4Ggrg2MbGnWO9X8K1t4+fGMDw==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/glob": {
|
||||
"version": "7.2.3",
|
||||
"resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
|
||||
"integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"fs.realpath": "^1.0.0",
|
||||
"inflight": "^1.0.4",
|
||||
"inherits": "2",
|
||||
"minimatch": "^3.1.1",
|
||||
"once": "^1.3.0",
|
||||
"path-is-absolute": "^1.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": "*"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/isaacs"
|
||||
}
|
||||
},
|
||||
"node_modules/growl": {
|
||||
"version": "1.10.5",
|
||||
"resolved": "https://registry.npmjs.org/growl/-/growl-1.10.5.tgz",
|
||||
"integrity": "sha512-qBr4OuELkhPenW6goKVXiv47US3clb3/IbuWF9KNKEijAy9oeHxU9IgzjvJhHkUzhaj7rOUD7+YGWqUjLp5oSA==",
|
||||
"dev": true,
|
||||
"engines": {
|
||||
"node": ">=4.x"
|
||||
}
|
||||
},
|
||||
"node_modules/has-flag": {
|
||||
"version": "3.0.0",
|
||||
"resolved": "https://registry.npmjs.org/has-flag/-/has-flag-3.0.0.tgz",
|
||||
"integrity": "sha512-sKJf1+ceQBr4SMkvQnBDNDtf4TXpVhVGateu0t918bl30FnbE2m4vNLX+VWe/dpjlb+HugGYzW7uQXH98HPEYw==",
|
||||
"dev": true,
|
||||
"engines": {
|
||||
"node": ">=4"
|
||||
}
|
||||
},
|
||||
"node_modules/he": {
|
||||
"version": "1.1.1",
|
||||
"resolved": "https://registry.npmjs.org/he/-/he-1.1.1.tgz",
|
||||
"integrity": "sha512-z/GDPjlRMNOa2XJiB4em8wJpuuBfrFOlYKTZxtpkdr1uPdibHI8rYA3MY0KDObpVyaes0e/aunid/t88ZI2EKA==",
|
||||
"dev": true,
|
||||
"bin": {
|
||||
"he": "bin/he"
|
||||
}
|
||||
},
|
||||
"node_modules/http-proxy-agent": {
|
||||
"version": "4.0.1",
|
||||
"resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-4.0.1.tgz",
|
||||
"integrity": "sha512-k0zdNgqWTGA6aeIRVpvfVob4fL52dTfaehylg0Y4UvSySvOq/Y+BOyPrgpUrA7HylqvU8vIZGsRuXmspskV0Tg==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"@tootallnate/once": "1",
|
||||
"agent-base": "6",
|
||||
"debug": "4"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 6"
|
||||
}
|
||||
},
|
||||
"node_modules/https-proxy-agent": {
|
||||
"version": "5.0.1",
|
||||
"resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-5.0.1.tgz",
|
||||
"integrity": "sha512-dFcAjpTQFgoLMzC2VwU+C/CbS7uRL0lWmxDITmqm7C+7F0Odmj6s9l6alZc6AELXhrnggM2CeWSXHGOdX2YtwA==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"agent-base": "6",
|
||||
"debug": "4"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 6"
|
||||
}
|
||||
},
|
||||
"node_modules/inflight": {
|
||||
"version": "1.0.6",
|
||||
"resolved": "https://registry.npmjs.org/inflight/-/inflight-1.0.6.tgz",
|
||||
"integrity": "sha512-k92I/b08q4wvFscXCLvqfsHCrjrF7yiXsQuIVvVE7N82W3+aqpzuUdBbfhWcy/FZR3/4IgflMgKLOsvPDrGCJA==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"once": "^1.3.0",
|
||||
"wrappy": "1"
|
||||
}
|
||||
},
|
||||
"node_modules/inherits": {
|
||||
"version": "2.0.4",
|
||||
"resolved": "https://registry.npmjs.org/inherits/-/inherits-2.0.4.tgz",
|
||||
"integrity": "sha512-k/vGaX4/Yla3WzyMCvTQOXYeIHvqOKtnqBduzTHpzpQZzAskKMhZ2K+EnBiSM9zGSoIFeMpXKxa4dYeZIQqewQ==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/minimatch": {
|
||||
"version": "3.1.2",
|
||||
"resolved": "https://registry.npmjs.org/minimatch/-/minimatch-3.1.2.tgz",
|
||||
"integrity": "sha512-J7p63hRiAjw1NDEww1W7i37+ByIrOWO5XQQAzZ3VOcL0PNybwpfmV/N05zFAzwQ9USyEcX6t3UO+K5aqBQOIHw==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"brace-expansion": "^1.1.7"
|
||||
},
|
||||
"engines": {
|
||||
"node": "*"
|
||||
}
|
||||
},
|
||||
"node_modules/minimist": {
|
||||
"version": "0.0.8",
|
||||
"resolved": "https://registry.npmjs.org/minimist/-/minimist-0.0.8.tgz",
|
||||
"integrity": "sha512-miQKw5Hv4NS1Psg2517mV4e4dYNaO3++hjAvLOAzKqZ61rH8NS1SK+vbfBWZ5PY/Me/bEWhUwqMghEW5Fb9T7Q==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/mkdirp": {
|
||||
"version": "0.5.1",
|
||||
"resolved": "https://registry.npmjs.org/mkdirp/-/mkdirp-0.5.1.tgz",
|
||||
"integrity": "sha512-SknJC52obPfGQPnjIkXbmA6+5H15E+fR+E4iR2oQ3zzCLbd7/ONua69R/Gw7AgkTLsRG+r5fzksYwWe1AgTyWA==",
|
||||
"deprecated": "Legacy versions of mkdirp are no longer supported. Please update to mkdirp 1.x. (Note that the API surface has changed to use Promises in 1.x.)",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"minimist": "0.0.8"
|
||||
},
|
||||
"bin": {
|
||||
"mkdirp": "bin/cmd.js"
|
||||
}
|
||||
},
|
||||
"node_modules/mocha": {
|
||||
"version": "5.2.0",
|
||||
"resolved": "https://registry.npmjs.org/mocha/-/mocha-5.2.0.tgz",
|
||||
"integrity": "sha512-2IUgKDhc3J7Uug+FxMXuqIyYzH7gJjXECKe/w43IGgQHTSj3InJi+yAA7T24L9bQMRKiUEHxEX37G5JpVUGLcQ==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"browser-stdout": "1.3.1",
|
||||
"commander": "2.15.1",
|
||||
"debug": "3.1.0",
|
||||
"diff": "3.5.0",
|
||||
"escape-string-regexp": "1.0.5",
|
||||
"glob": "7.1.2",
|
||||
"growl": "1.10.5",
|
||||
"he": "1.1.1",
|
||||
"minimatch": "3.0.4",
|
||||
"mkdirp": "0.5.1",
|
||||
"supports-color": "5.4.0"
|
||||
},
|
||||
"bin": {
|
||||
"_mocha": "bin/_mocha",
|
||||
"mocha": "bin/mocha"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 4.0.0"
|
||||
}
|
||||
},
|
||||
"node_modules/mocha/node_modules/debug": {
|
||||
"version": "3.1.0",
|
||||
"resolved": "https://registry.npmjs.org/debug/-/debug-3.1.0.tgz",
|
||||
"integrity": "sha512-OX8XqP7/1a9cqkxYw2yXss15f26NKWBpDXQd0/uK/KPqdQhxbPa994hnzjcE2VqQpDslf55723cKPUOGSmMY3g==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"ms": "2.0.0"
|
||||
}
|
||||
},
|
||||
"node_modules/mocha/node_modules/glob": {
|
||||
"version": "7.1.2",
|
||||
"resolved": "https://registry.npmjs.org/glob/-/glob-7.1.2.tgz",
|
||||
"integrity": "sha512-MJTUg1kjuLeQCJ+ccE4Vpa6kKVXkPYJ2mOCQyUuKLcLQsdrMCpBPUi8qVE6+YuaJkozeA9NusTAw3hLr8Xe5EQ==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"fs.realpath": "^1.0.0",
|
||||
"inflight": "^1.0.4",
|
||||
"inherits": "2",
|
||||
"minimatch": "^3.0.4",
|
||||
"once": "^1.3.0",
|
||||
"path-is-absolute": "^1.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": "*"
|
||||
}
|
||||
},
|
||||
"node_modules/mocha/node_modules/minimatch": {
|
||||
"version": "3.0.4",
|
||||
"resolved": "https://registry.npmjs.org/minimatch/-/minimatch-3.0.4.tgz",
|
||||
"integrity": "sha512-yJHVQEhyqPLUTgt9B83PXu6W3rx4MvvHvSUvToogpwoGDOUQ+yDrR0HRot+yOCdCO7u4hX3pWft6kWBBcqh0UA==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"brace-expansion": "^1.1.7"
|
||||
},
|
||||
"engines": {
|
||||
"node": "*"
|
||||
}
|
||||
},
|
||||
"node_modules/mocha/node_modules/ms": {
|
||||
"version": "2.0.0",
|
||||
"resolved": "https://registry.npmjs.org/ms/-/ms-2.0.0.tgz",
|
||||
"integrity": "sha512-Tpp60P6IUJDTuOq/5Z8cdskzJujfwqfOTkrwIwj7IRISpnkJnT6SyJ4PCPnGMoFjC9ddhal5KVIYtAt97ix05A==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/ms": {
|
||||
"version": "2.1.2",
|
||||
"resolved": "https://registry.npmjs.org/ms/-/ms-2.1.2.tgz",
|
||||
"integrity": "sha512-sGkPx+VjMtmA6MX27oA4FBFELFCZZ4S4XqeGOXCv68tT+jb3vk/RyaKWP0PTKyWtmLSM0b+adUTEvbs1PEaH2w==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/once": {
|
||||
"version": "1.4.0",
|
||||
"resolved": "https://registry.npmjs.org/once/-/once-1.4.0.tgz",
|
||||
"integrity": "sha512-lNaJgI+2Q5URQBkccEKHTQOPaXdUxnZZElQTZY0MFUAuaEqe1E+Nyvgdz/aIyNi6Z9MzO5dv1H8n58/GELp3+w==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"wrappy": "1"
|
||||
}
|
||||
},
|
||||
"node_modules/path-is-absolute": {
|
||||
"version": "1.0.1",
|
||||
"resolved": "https://registry.npmjs.org/path-is-absolute/-/path-is-absolute-1.0.1.tgz",
|
||||
"integrity": "sha512-AVbw3UJ2e9bq64vSaS9Am0fje1Pa8pbGqTTsmXfaIiMpnr5DlDhfJOuLj9Sf95ZPVDAUerDfEk88MPmPe7UCQg==",
|
||||
"dev": true,
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
}
|
||||
},
|
||||
"node_modules/semver": {
|
||||
"version": "5.7.1",
|
||||
"resolved": "https://registry.npmjs.org/semver/-/semver-5.7.1.tgz",
|
||||
"integrity": "sha512-sauaDf/PZdVgrLTNYHRtpXa1iRiKcaebiKQ1BJdpQlWH2lCvexQdX55snPFyK7QzpudqbCI0qXFfOasHdyNDGQ==",
|
||||
"dev": true,
|
||||
"bin": {
|
||||
"semver": "bin/semver"
|
||||
}
|
||||
},
|
||||
"node_modules/source-map": {
|
||||
"version": "0.6.1",
|
||||
"resolved": "https://registry.npmjs.org/source-map/-/source-map-0.6.1.tgz",
|
||||
"integrity": "sha512-UjgapumWlbMhkBgzT7Ykc5YXUT46F0iKu8SGXq0bcwP5dz/h0Plj6enJqjz1Zbq2l5WaqYnrVbwWOWMyF3F47g==",
|
||||
"dev": true,
|
||||
"engines": {
|
||||
"node": ">=0.10.0"
|
||||
}
|
||||
},
|
||||
"node_modules/source-map-support": {
|
||||
"version": "0.5.21",
|
||||
"resolved": "https://registry.npmjs.org/source-map-support/-/source-map-support-0.5.21.tgz",
|
||||
"integrity": "sha512-uBHU3L3czsIyYXKX88fdrGovxdSCoTGDRZ6SYXtSRxLZUzHg5P/66Ht6uoUlHu9EZod+inXhKo3qQgwXUT/y1w==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"buffer-from": "^1.0.0",
|
||||
"source-map": "^0.6.0"
|
||||
}
|
||||
},
|
||||
"node_modules/supports-color": {
|
||||
"version": "5.4.0",
|
||||
"resolved": "https://registry.npmjs.org/supports-color/-/supports-color-5.4.0.tgz",
|
||||
"integrity": "sha512-zjaXglF5nnWpsq470jSv6P9DwPvgLkuapYmfDm3JWOm0vkNTVF2tI4UrN2r6jH1qM/uc/WtxYY1hYoA2dOKj5w==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"has-flag": "^3.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=4"
|
||||
}
|
||||
},
|
||||
"node_modules/vscode": {
|
||||
"version": "1.1.37",
|
||||
"resolved": "https://registry.npmjs.org/vscode/-/vscode-1.1.37.tgz",
|
||||
"integrity": "sha512-vJNj6IlN7IJPdMavlQa1KoFB3Ihn06q1AiN3ZFI/HfzPNzbKZWPPuiU+XkpNOfGU5k15m4r80nxNPlM7wcc0wg==",
|
||||
"deprecated": "This package is deprecated in favor of @types/vscode and vscode-test. For more information please read: https://code.visualstudio.com/updates/v1_36#_splitting-vscode-package-into-typesvscode-and-vscodetest",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"glob": "^7.1.2",
|
||||
"http-proxy-agent": "^4.0.1",
|
||||
"https-proxy-agent": "^5.0.0",
|
||||
"mocha": "^5.2.0",
|
||||
"semver": "^5.4.1",
|
||||
"source-map-support": "^0.5.0",
|
||||
"vscode-test": "^0.4.1"
|
||||
},
|
||||
"bin": {
|
||||
"vscode-install": "bin/install"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=8.9.3"
|
||||
}
|
||||
},
|
||||
"node_modules/vscode-test": {
|
||||
"version": "0.4.3",
|
||||
"resolved": "https://registry.npmjs.org/vscode-test/-/vscode-test-0.4.3.tgz",
|
||||
"integrity": "sha512-EkMGqBSefZH2MgW65nY05rdRSko15uvzq4VAPM5jVmwYuFQKE7eikKXNJDRxL+OITXHB6pI+a3XqqD32Y3KC5w==",
|
||||
"deprecated": "This package has been renamed to @vscode/test-electron, please update to the new name",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"http-proxy-agent": "^2.1.0",
|
||||
"https-proxy-agent": "^2.2.1"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=8.9.3"
|
||||
}
|
||||
},
|
||||
"node_modules/vscode-test/node_modules/agent-base": {
|
||||
"version": "4.3.0",
|
||||
"resolved": "https://registry.npmjs.org/agent-base/-/agent-base-4.3.0.tgz",
|
||||
"integrity": "sha512-salcGninV0nPrwpGNn4VTXBb1SOuXQBiqbrNXoeizJsHrsL6ERFM2Ne3JUSBWRE6aeNJI2ROP/WEEIDUiDe3cg==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"es6-promisify": "^5.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 4.0.0"
|
||||
}
|
||||
},
|
||||
"node_modules/vscode-test/node_modules/debug": {
|
||||
"version": "3.1.0",
|
||||
"resolved": "https://registry.npmjs.org/debug/-/debug-3.1.0.tgz",
|
||||
"integrity": "sha512-OX8XqP7/1a9cqkxYw2yXss15f26NKWBpDXQd0/uK/KPqdQhxbPa994hnzjcE2VqQpDslf55723cKPUOGSmMY3g==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"ms": "2.0.0"
|
||||
}
|
||||
},
|
||||
"node_modules/vscode-test/node_modules/http-proxy-agent": {
|
||||
"version": "2.1.0",
|
||||
"resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-2.1.0.tgz",
|
||||
"integrity": "sha512-qwHbBLV7WviBl0rQsOzH6o5lwyOIvwp/BdFnvVxXORldu5TmjFfjzBcWUWS5kWAZhmv+JtiDhSuQCp4sBfbIgg==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"agent-base": "4",
|
||||
"debug": "3.1.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 4.5.0"
|
||||
}
|
||||
},
|
||||
"node_modules/vscode-test/node_modules/https-proxy-agent": {
|
||||
"version": "2.2.4",
|
||||
"resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-2.2.4.tgz",
|
||||
"integrity": "sha512-OmvfoQ53WLjtA9HeYP9RNrWMJzzAz1JGaSFr1nijg0PVR1JaD/xbJq1mdEIIlxGpXp9eSe/O2LgU9DJmTPd0Eg==",
|
||||
"dev": true,
|
||||
"dependencies": {
|
||||
"agent-base": "^4.3.0",
|
||||
"debug": "^3.1.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 4.5.0"
|
||||
}
|
||||
},
|
||||
"node_modules/vscode-test/node_modules/ms": {
|
||||
"version": "2.0.0",
|
||||
"resolved": "https://registry.npmjs.org/ms/-/ms-2.0.0.tgz",
|
||||
"integrity": "sha512-Tpp60P6IUJDTuOq/5Z8cdskzJujfwqfOTkrwIwj7IRISpnkJnT6SyJ4PCPnGMoFjC9ddhal5KVIYtAt97ix05A==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/wrappy": {
|
||||
"version": "1.0.2",
|
||||
"resolved": "https://registry.npmjs.org/wrappy/-/wrappy-1.0.2.tgz",
|
||||
"integrity": "sha512-l4Sp/DRseor9wL6EvV2+TuQn63dMkPjZ/sp9XkghTEbV9KlPS1xUsZ3u7/IQO4wxtcFB4bgpQPRcR3QCvezPcQ==",
|
||||
"dev": true
|
||||
}
|
||||
}
|
||||
}
|
|
@ -0,0 +1,14 @@
|
|||
/// <reference types="node" />
|
||||
import { EventEmitter } from 'events';
|
||||
declare function once<T>(emitter: EventEmitter, name: string): once.CancelablePromise<T>;
|
||||
declare namespace once {
|
||||
interface CancelFunction {
|
||||
(): void;
|
||||
}
|
||||
interface CancelablePromise<T> extends Promise<T> {
|
||||
cancel: CancelFunction;
|
||||
}
|
||||
type CancellablePromise<T> = CancelablePromise<T>;
|
||||
function spread<T extends any[]>(emitter: EventEmitter, name: string): once.CancelablePromise<T>;
|
||||
}
|
||||
export = once;
|
|
@ -0,0 +1,39 @@
|
|||
"use strict";
|
||||
function noop() { }
|
||||
function once(emitter, name) {
|
||||
const o = once.spread(emitter, name);
|
||||
const r = o.then((args) => args[0]);
|
||||
r.cancel = o.cancel;
|
||||
return r;
|
||||
}
|
||||
(function (once) {
|
||||
function spread(emitter, name) {
|
||||
let c = null;
|
||||
const p = new Promise((resolve, reject) => {
|
||||
function cancel() {
|
||||
emitter.removeListener(name, onEvent);
|
||||
emitter.removeListener('error', onError);
|
||||
p.cancel = noop;
|
||||
}
|
||||
function onEvent(...args) {
|
||||
cancel();
|
||||
resolve(args);
|
||||
}
|
||||
function onError(err) {
|
||||
cancel();
|
||||
reject(err);
|
||||
}
|
||||
c = cancel;
|
||||
emitter.on(name, onEvent);
|
||||
emitter.on('error', onError);
|
||||
});
|
||||
if (!c) {
|
||||
throw new TypeError('Could not get `cancel()` function');
|
||||
}
|
||||
p.cancel = c;
|
||||
return p;
|
||||
}
|
||||
once.spread = spread;
|
||||
})(once || (once = {}));
|
||||
module.exports = once;
|
||||
//# sourceMappingURL=index.js.map
|
|
@ -0,0 +1 @@
|
|||
{"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";AAEA,SAAS,IAAI,KAAI,CAAC;AAElB,SAAS,IAAI,CACZ,OAAqB,EACrB,IAAY;IAEZ,MAAM,CAAC,GAAG,IAAI,CAAC,MAAM,CAAM,OAAO,EAAE,IAAI,CAAC,CAAC;IAC1C,MAAM,CAAC,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC,IAAS,EAAE,EAAE,CAAC,IAAI,CAAC,CAAC,CAAC,CAA8B,CAAC;IACtE,CAAC,CAAC,MAAM,GAAG,CAAC,CAAC,MAAM,CAAC;IACpB,OAAO,CAAC,CAAC;AACV,CAAC;AAED,WAAU,IAAI;IAWb,SAAgB,MAAM,CACrB,OAAqB,EACrB,IAAY;QAEZ,IAAI,CAAC,GAA+B,IAAI,CAAC;QACzC,MAAM,CAAC,GAAG,IAAI,OAAO,CAAI,CAAC,OAAO,EAAE,MAAM,EAAE,EAAE;YAC5C,SAAS,MAAM;gBACd,OAAO,CAAC,cAAc,CAAC,IAAI,EAAE,OAAO,CAAC,CAAC;gBACtC,OAAO,CAAC,cAAc,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;gBACzC,CAAC,CAAC,MAAM,GAAG,IAAI,CAAC;YACjB,CAAC;YACD,SAAS,OAAO,CAAC,GAAG,IAAW;gBAC9B,MAAM,EAAE,CAAC;gBACT,OAAO,CAAC,IAAS,CAAC,CAAC;YACpB,CAAC;YACD,SAAS,OAAO,CAAC,GAAU;gBAC1B,MAAM,EAAE,CAAC;gBACT,MAAM,CAAC,GAAG,CAAC,CAAC;YACb,CAAC;YACD,CAAC,GAAG,MAAM,CAAC;YACX,OAAO,CAAC,EAAE,CAAC,IAAI,EAAE,OAAO,CAAC,CAAC;YAC1B,OAAO,CAAC,EAAE,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;QAC9B,CAAC,CAA8B,CAAC;QAChC,IAAI,CAAC,CAAC,EAAE;YACP,MAAM,IAAI,SAAS,CAAC,mCAAmC,CAAC,CAAC;SACzD;QACD,CAAC,CAAC,MAAM,GAAG,CAAC,CAAC;QACb,OAAO,CAAC,CAAC;IACV,CAAC;IA5Be,WAAM,SA4BrB,CAAA;AACF,CAAC,EAxCS,IAAI,KAAJ,IAAI,QAwCb;AAED,iBAAS,IAAI,CAAC"}
|
|
@ -0,0 +1,45 @@
|
|||
{
|
||||
"name": "@tootallnate/once",
|
||||
"version": "1.1.2",
|
||||
"description": "Creates a Promise that waits for a single event",
|
||||
"main": "./dist/index.js",
|
||||
"types": "./dist/index.d.ts",
|
||||
"files": [
|
||||
"dist"
|
||||
],
|
||||
"scripts": {
|
||||
"prebuild": "rimraf dist",
|
||||
"build": "tsc",
|
||||
"test": "mocha --reporter spec",
|
||||
"test-lint": "eslint src --ext .js,.ts",
|
||||
"prepublishOnly": "npm run build"
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/TooTallNate/once.git"
|
||||
},
|
||||
"keywords": [],
|
||||
"author": "Nathan Rajlich <nathan@tootallnate.net> (http://n8.io/)",
|
||||
"license": "MIT",
|
||||
"bugs": {
|
||||
"url": "https://github.com/TooTallNate/once/issues"
|
||||
},
|
||||
"devDependencies": {
|
||||
"@types/node": "^12.12.11",
|
||||
"@typescript-eslint/eslint-plugin": "1.6.0",
|
||||
"@typescript-eslint/parser": "1.1.0",
|
||||
"eslint": "5.16.0",
|
||||
"eslint-config-airbnb": "17.1.0",
|
||||
"eslint-config-prettier": "4.1.0",
|
||||
"eslint-import-resolver-typescript": "1.1.1",
|
||||
"eslint-plugin-import": "2.16.0",
|
||||
"eslint-plugin-jsx-a11y": "6.2.1",
|
||||
"eslint-plugin-react": "7.12.4",
|
||||
"mocha": "^6.2.2",
|
||||
"rimraf": "^3.0.0",
|
||||
"typescript": "^3.7.3"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 6"
|
||||
}
|
||||
}
|
|
@ -0,0 +1,145 @@
|
|||
agent-base
|
||||
==========
|
||||
### Turn a function into an [`http.Agent`][http.Agent] instance
|
||||
[](https://github.com/TooTallNate/node-agent-base/actions?workflow=Node+CI)
|
||||
|
||||
This module provides an `http.Agent` generator. That is, you pass it an async
|
||||
callback function, and it returns a new `http.Agent` instance that will invoke the
|
||||
given callback function when sending outbound HTTP requests.
|
||||
|
||||
#### Some subclasses:
|
||||
|
||||
Here's some more interesting uses of `agent-base`.
|
||||
Send a pull request to list yours!
|
||||
|
||||
* [`http-proxy-agent`][http-proxy-agent]: An HTTP(s) proxy `http.Agent` implementation for HTTP endpoints
|
||||
* [`https-proxy-agent`][https-proxy-agent]: An HTTP(s) proxy `http.Agent` implementation for HTTPS endpoints
|
||||
* [`pac-proxy-agent`][pac-proxy-agent]: A PAC file proxy `http.Agent` implementation for HTTP and HTTPS
|
||||
* [`socks-proxy-agent`][socks-proxy-agent]: A SOCKS proxy `http.Agent` implementation for HTTP and HTTPS
|
||||
|
||||
|
||||
Installation
|
||||
------------
|
||||
|
||||
Install with `npm`:
|
||||
|
||||
``` bash
|
||||
$ npm install agent-base
|
||||
```
|
||||
|
||||
|
||||
Example
|
||||
-------
|
||||
|
||||
Here's a minimal example that creates a new `net.Socket` connection to the server
|
||||
for every HTTP request (i.e. the equivalent of `agent: false` option):
|
||||
|
||||
```js
|
||||
var net = require('net');
|
||||
var tls = require('tls');
|
||||
var url = require('url');
|
||||
var http = require('http');
|
||||
var agent = require('agent-base');
|
||||
|
||||
var endpoint = 'http://nodejs.org/api/';
|
||||
var parsed = url.parse(endpoint);
|
||||
|
||||
// This is the important part!
|
||||
parsed.agent = agent(function (req, opts) {
|
||||
var socket;
|
||||
// `secureEndpoint` is true when using the https module
|
||||
if (opts.secureEndpoint) {
|
||||
socket = tls.connect(opts);
|
||||
} else {
|
||||
socket = net.connect(opts);
|
||||
}
|
||||
return socket;
|
||||
});
|
||||
|
||||
// Everything else works just like normal...
|
||||
http.get(parsed, function (res) {
|
||||
console.log('"response" event!', res.headers);
|
||||
res.pipe(process.stdout);
|
||||
});
|
||||
```
|
||||
|
||||
Returning a Promise or using an `async` function is also supported:
|
||||
|
||||
```js
|
||||
agent(async function (req, opts) {
|
||||
await sleep(1000);
|
||||
// etc…
|
||||
});
|
||||
```
|
||||
|
||||
Return another `http.Agent` instance to "pass through" the responsibility
|
||||
for that HTTP request to that agent:
|
||||
|
||||
```js
|
||||
agent(function (req, opts) {
|
||||
return opts.secureEndpoint ? https.globalAgent : http.globalAgent;
|
||||
});
|
||||
```
|
||||
|
||||
|
||||
API
|
||||
---
|
||||
|
||||
## Agent(Function callback[, Object options]) → [http.Agent][]
|
||||
|
||||
Creates a base `http.Agent` that will execute the callback function `callback`
|
||||
for every HTTP request that it is used as the `agent` for. The callback function
|
||||
is responsible for creating a `stream.Duplex` instance of some kind that will be
|
||||
used as the underlying socket in the HTTP request.
|
||||
|
||||
The `options` object accepts the following properties:
|
||||
|
||||
* `timeout` - Number - Timeout for the `callback()` function in milliseconds. Defaults to Infinity (optional).
|
||||
|
||||
The callback function should have the following signature:
|
||||
|
||||
### callback(http.ClientRequest req, Object options, Function cb) → undefined
|
||||
|
||||
The ClientRequest `req` can be accessed to read request headers and
|
||||
and the path, etc. The `options` object contains the options passed
|
||||
to the `http.request()`/`https.request()` function call, and is formatted
|
||||
to be directly passed to `net.connect()`/`tls.connect()`, or however
|
||||
else you want a Socket to be created. Pass the created socket to
|
||||
the callback function `cb` once created, and the HTTP request will
|
||||
continue to proceed.
|
||||
|
||||
If the `https` module is used to invoke the HTTP request, then the
|
||||
`secureEndpoint` property on `options` _will be set to `true`_.
|
||||
|
||||
|
||||
License
|
||||
-------
|
||||
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2013 Nathan Rajlich <nathan@tootallnate.net>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
||||
[http-proxy-agent]: https://github.com/TooTallNate/node-http-proxy-agent
|
||||
[https-proxy-agent]: https://github.com/TooTallNate/node-https-proxy-agent
|
||||
[pac-proxy-agent]: https://github.com/TooTallNate/node-pac-proxy-agent
|
||||
[socks-proxy-agent]: https://github.com/TooTallNate/node-socks-proxy-agent
|
||||
[http.Agent]: https://nodejs.org/api/http.html#http_class_http_agent
|
|
@ -0,0 +1,78 @@
|
|||
/// <reference types="node" />
|
||||
import net from 'net';
|
||||
import http from 'http';
|
||||
import https from 'https';
|
||||
import { Duplex } from 'stream';
|
||||
import { EventEmitter } from 'events';
|
||||
declare function createAgent(opts?: createAgent.AgentOptions): createAgent.Agent;
|
||||
declare function createAgent(callback: createAgent.AgentCallback, opts?: createAgent.AgentOptions): createAgent.Agent;
|
||||
declare namespace createAgent {
|
||||
interface ClientRequest extends http.ClientRequest {
|
||||
_last?: boolean;
|
||||
_hadError?: boolean;
|
||||
method: string;
|
||||
}
|
||||
interface AgentRequestOptions {
|
||||
host?: string;
|
||||
path?: string;
|
||||
port: number;
|
||||
}
|
||||
interface HttpRequestOptions extends AgentRequestOptions, Omit<http.RequestOptions, keyof AgentRequestOptions> {
|
||||
secureEndpoint: false;
|
||||
}
|
||||
interface HttpsRequestOptions extends AgentRequestOptions, Omit<https.RequestOptions, keyof AgentRequestOptions> {
|
||||
secureEndpoint: true;
|
||||
}
|
||||
type RequestOptions = HttpRequestOptions | HttpsRequestOptions;
|
||||
type AgentLike = Pick<createAgent.Agent, 'addRequest'> | http.Agent;
|
||||
type AgentCallbackReturn = Duplex | AgentLike;
|
||||
type AgentCallbackCallback = (err?: Error | null, socket?: createAgent.AgentCallbackReturn) => void;
|
||||
type AgentCallbackPromise = (req: createAgent.ClientRequest, opts: createAgent.RequestOptions) => createAgent.AgentCallbackReturn | Promise<createAgent.AgentCallbackReturn>;
|
||||
type AgentCallback = typeof Agent.prototype.callback;
|
||||
type AgentOptions = {
|
||||
timeout?: number;
|
||||
};
|
||||
/**
|
||||
* Base `http.Agent` implementation.
|
||||
* No pooling/keep-alive is implemented by default.
|
||||
*
|
||||
* @param {Function} callback
|
||||
* @api public
|
||||
*/
|
||||
class Agent extends EventEmitter {
|
||||
timeout: number | null;
|
||||
maxFreeSockets: number;
|
||||
maxTotalSockets: number;
|
||||
maxSockets: number;
|
||||
sockets: {
|
||||
[key: string]: net.Socket[];
|
||||
};
|
||||
freeSockets: {
|
||||
[key: string]: net.Socket[];
|
||||
};
|
||||
requests: {
|
||||
[key: string]: http.IncomingMessage[];
|
||||
};
|
||||
options: https.AgentOptions;
|
||||
private promisifiedCallback?;
|
||||
private explicitDefaultPort?;
|
||||
private explicitProtocol?;
|
||||
constructor(callback?: createAgent.AgentCallback | createAgent.AgentOptions, _opts?: createAgent.AgentOptions);
|
||||
get defaultPort(): number;
|
||||
set defaultPort(v: number);
|
||||
get protocol(): string;
|
||||
set protocol(v: string);
|
||||
callback(req: createAgent.ClientRequest, opts: createAgent.RequestOptions, fn: createAgent.AgentCallbackCallback): void;
|
||||
callback(req: createAgent.ClientRequest, opts: createAgent.RequestOptions): createAgent.AgentCallbackReturn | Promise<createAgent.AgentCallbackReturn>;
|
||||
/**
|
||||
* Called by node-core's "_http_client.js" module when creating
|
||||
* a new HTTP request with this Agent instance.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
addRequest(req: ClientRequest, _opts: RequestOptions): void;
|
||||
freeSocket(socket: net.Socket, opts: AgentOptions): void;
|
||||
destroy(): void;
|
||||
}
|
||||
}
|
||||
export = createAgent;
|
|
@ -0,0 +1,203 @@
|
|||
"use strict";
|
||||
var __importDefault = (this && this.__importDefault) || function (mod) {
|
||||
return (mod && mod.__esModule) ? mod : { "default": mod };
|
||||
};
|
||||
const events_1 = require("events");
|
||||
const debug_1 = __importDefault(require("debug"));
|
||||
const promisify_1 = __importDefault(require("./promisify"));
|
||||
const debug = debug_1.default('agent-base');
|
||||
function isAgent(v) {
|
||||
return Boolean(v) && typeof v.addRequest === 'function';
|
||||
}
|
||||
function isSecureEndpoint() {
|
||||
const { stack } = new Error();
|
||||
if (typeof stack !== 'string')
|
||||
return false;
|
||||
return stack.split('\n').some(l => l.indexOf('(https.js:') !== -1 || l.indexOf('node:https:') !== -1);
|
||||
}
|
||||
function createAgent(callback, opts) {
|
||||
return new createAgent.Agent(callback, opts);
|
||||
}
|
||||
(function (createAgent) {
|
||||
/**
|
||||
* Base `http.Agent` implementation.
|
||||
* No pooling/keep-alive is implemented by default.
|
||||
*
|
||||
* @param {Function} callback
|
||||
* @api public
|
||||
*/
|
||||
class Agent extends events_1.EventEmitter {
|
||||
constructor(callback, _opts) {
|
||||
super();
|
||||
let opts = _opts;
|
||||
if (typeof callback === 'function') {
|
||||
this.callback = callback;
|
||||
}
|
||||
else if (callback) {
|
||||
opts = callback;
|
||||
}
|
||||
// Timeout for the socket to be returned from the callback
|
||||
this.timeout = null;
|
||||
if (opts && typeof opts.timeout === 'number') {
|
||||
this.timeout = opts.timeout;
|
||||
}
|
||||
// These aren't actually used by `agent-base`, but are required
|
||||
// for the TypeScript definition files in `@types/node` :/
|
||||
this.maxFreeSockets = 1;
|
||||
this.maxSockets = 1;
|
||||
this.maxTotalSockets = Infinity;
|
||||
this.sockets = {};
|
||||
this.freeSockets = {};
|
||||
this.requests = {};
|
||||
this.options = {};
|
||||
}
|
||||
get defaultPort() {
|
||||
if (typeof this.explicitDefaultPort === 'number') {
|
||||
return this.explicitDefaultPort;
|
||||
}
|
||||
return isSecureEndpoint() ? 443 : 80;
|
||||
}
|
||||
set defaultPort(v) {
|
||||
this.explicitDefaultPort = v;
|
||||
}
|
||||
get protocol() {
|
||||
if (typeof this.explicitProtocol === 'string') {
|
||||
return this.explicitProtocol;
|
||||
}
|
||||
return isSecureEndpoint() ? 'https:' : 'http:';
|
||||
}
|
||||
set protocol(v) {
|
||||
this.explicitProtocol = v;
|
||||
}
|
||||
callback(req, opts, fn) {
|
||||
throw new Error('"agent-base" has no default implementation, you must subclass and override `callback()`');
|
||||
}
|
||||
/**
|
||||
* Called by node-core's "_http_client.js" module when creating
|
||||
* a new HTTP request with this Agent instance.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
addRequest(req, _opts) {
|
||||
const opts = Object.assign({}, _opts);
|
||||
if (typeof opts.secureEndpoint !== 'boolean') {
|
||||
opts.secureEndpoint = isSecureEndpoint();
|
||||
}
|
||||
if (opts.host == null) {
|
||||
opts.host = 'localhost';
|
||||
}
|
||||
if (opts.port == null) {
|
||||
opts.port = opts.secureEndpoint ? 443 : 80;
|
||||
}
|
||||
if (opts.protocol == null) {
|
||||
opts.protocol = opts.secureEndpoint ? 'https:' : 'http:';
|
||||
}
|
||||
if (opts.host && opts.path) {
|
||||
// If both a `host` and `path` are specified then it's most
|
||||
// likely the result of a `url.parse()` call... we need to
|
||||
// remove the `path` portion so that `net.connect()` doesn't
|
||||
// attempt to open that as a unix socket file.
|
||||
delete opts.path;
|
||||
}
|
||||
delete opts.agent;
|
||||
delete opts.hostname;
|
||||
delete opts._defaultAgent;
|
||||
delete opts.defaultPort;
|
||||
delete opts.createConnection;
|
||||
// Hint to use "Connection: close"
|
||||
// XXX: non-documented `http` module API :(
|
||||
req._last = true;
|
||||
req.shouldKeepAlive = false;
|
||||
let timedOut = false;
|
||||
let timeoutId = null;
|
||||
const timeoutMs = opts.timeout || this.timeout;
|
||||
const onerror = (err) => {
|
||||
if (req._hadError)
|
||||
return;
|
||||
req.emit('error', err);
|
||||
// For Safety. Some additional errors might fire later on
|
||||
// and we need to make sure we don't double-fire the error event.
|
||||
req._hadError = true;
|
||||
};
|
||||
const ontimeout = () => {
|
||||
timeoutId = null;
|
||||
timedOut = true;
|
||||
const err = new Error(`A "socket" was not created for HTTP request before ${timeoutMs}ms`);
|
||||
err.code = 'ETIMEOUT';
|
||||
onerror(err);
|
||||
};
|
||||
const callbackError = (err) => {
|
||||
if (timedOut)
|
||||
return;
|
||||
if (timeoutId !== null) {
|
||||
clearTimeout(timeoutId);
|
||||
timeoutId = null;
|
||||
}
|
||||
onerror(err);
|
||||
};
|
||||
const onsocket = (socket) => {
|
||||
if (timedOut)
|
||||
return;
|
||||
if (timeoutId != null) {
|
||||
clearTimeout(timeoutId);
|
||||
timeoutId = null;
|
||||
}
|
||||
if (isAgent(socket)) {
|
||||
// `socket` is actually an `http.Agent` instance, so
|
||||
// relinquish responsibility for this `req` to the Agent
|
||||
// from here on
|
||||
debug('Callback returned another Agent instance %o', socket.constructor.name);
|
||||
socket.addRequest(req, opts);
|
||||
return;
|
||||
}
|
||||
if (socket) {
|
||||
socket.once('free', () => {
|
||||
this.freeSocket(socket, opts);
|
||||
});
|
||||
req.onSocket(socket);
|
||||
return;
|
||||
}
|
||||
const err = new Error(`no Duplex stream was returned to agent-base for \`${req.method} ${req.path}\``);
|
||||
onerror(err);
|
||||
};
|
||||
if (typeof this.callback !== 'function') {
|
||||
onerror(new Error('`callback` is not defined'));
|
||||
return;
|
||||
}
|
||||
if (!this.promisifiedCallback) {
|
||||
if (this.callback.length >= 3) {
|
||||
debug('Converting legacy callback function to promise');
|
||||
this.promisifiedCallback = promisify_1.default(this.callback);
|
||||
}
|
||||
else {
|
||||
this.promisifiedCallback = this.callback;
|
||||
}
|
||||
}
|
||||
if (typeof timeoutMs === 'number' && timeoutMs > 0) {
|
||||
timeoutId = setTimeout(ontimeout, timeoutMs);
|
||||
}
|
||||
if ('port' in opts && typeof opts.port !== 'number') {
|
||||
opts.port = Number(opts.port);
|
||||
}
|
||||
try {
|
||||
debug('Resolving socket for %o request: %o', opts.protocol, `${req.method} ${req.path}`);
|
||||
Promise.resolve(this.promisifiedCallback(req, opts)).then(onsocket, callbackError);
|
||||
}
|
||||
catch (err) {
|
||||
Promise.reject(err).catch(callbackError);
|
||||
}
|
||||
}
|
||||
freeSocket(socket, opts) {
|
||||
debug('Freeing socket %o %o', socket.constructor.name, opts);
|
||||
socket.destroy();
|
||||
}
|
||||
destroy() {
|
||||
debug('Destroying agent %o', this.constructor.name);
|
||||
}
|
||||
}
|
||||
createAgent.Agent = Agent;
|
||||
// So that `instanceof` works correctly
|
||||
createAgent.prototype = createAgent.Agent.prototype;
|
||||
})(createAgent || (createAgent = {}));
|
||||
module.exports = createAgent;
|
||||
//# sourceMappingURL=index.js.map
|
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,4 @@
|
|||
import { ClientRequest, RequestOptions, AgentCallbackCallback, AgentCallbackPromise } from './index';
|
||||
declare type LegacyCallback = (req: ClientRequest, opts: RequestOptions, fn: AgentCallbackCallback) => void;
|
||||
export default function promisify(fn: LegacyCallback): AgentCallbackPromise;
|
||||
export {};
|
|
@ -0,0 +1,18 @@
|
|||
"use strict";
|
||||
Object.defineProperty(exports, "__esModule", { value: true });
|
||||
function promisify(fn) {
|
||||
return function (req, opts) {
|
||||
return new Promise((resolve, reject) => {
|
||||
fn.call(this, req, opts, (err, rtn) => {
|
||||
if (err) {
|
||||
reject(err);
|
||||
}
|
||||
else {
|
||||
resolve(rtn);
|
||||
}
|
||||
});
|
||||
});
|
||||
};
|
||||
}
|
||||
exports.default = promisify;
|
||||
//# sourceMappingURL=promisify.js.map
|
|
@ -0,0 +1 @@
|
|||
{"version":3,"file":"promisify.js","sourceRoot":"","sources":["../../src/promisify.ts"],"names":[],"mappings":";;AAeA,SAAwB,SAAS,CAAC,EAAkB;IACnD,OAAO,UAAsB,GAAkB,EAAE,IAAoB;QACpE,OAAO,IAAI,OAAO,CAAC,CAAC,OAAO,EAAE,MAAM,EAAE,EAAE;YACtC,EAAE,CAAC,IAAI,CACN,IAAI,EACJ,GAAG,EACH,IAAI,EACJ,CAAC,GAA6B,EAAE,GAAyB,EAAE,EAAE;gBAC5D,IAAI,GAAG,EAAE;oBACR,MAAM,CAAC,GAAG,CAAC,CAAC;iBACZ;qBAAM;oBACN,OAAO,CAAC,GAAG,CAAC,CAAC;iBACb;YACF,CAAC,CACD,CAAC;QACH,CAAC,CAAC,CAAC;IACJ,CAAC,CAAC;AACH,CAAC;AAjBD,4BAiBC"}
|
|
@ -0,0 +1,64 @@
|
|||
{
|
||||
"name": "agent-base",
|
||||
"version": "6.0.2",
|
||||
"description": "Turn a function into an `http.Agent` instance",
|
||||
"main": "dist/src/index",
|
||||
"typings": "dist/src/index",
|
||||
"files": [
|
||||
"dist/src",
|
||||
"src"
|
||||
],
|
||||
"scripts": {
|
||||
"prebuild": "rimraf dist",
|
||||
"build": "tsc",
|
||||
"postbuild": "cpy --parents src test '!**/*.ts' dist",
|
||||
"test": "mocha --reporter spec dist/test/*.js",
|
||||
"test-lint": "eslint src --ext .js,.ts",
|
||||
"prepublishOnly": "npm run build"
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/TooTallNate/node-agent-base.git"
|
||||
},
|
||||
"keywords": [
|
||||
"http",
|
||||
"agent",
|
||||
"base",
|
||||
"barebones",
|
||||
"https"
|
||||
],
|
||||
"author": "Nathan Rajlich <nathan@tootallnate.net> (http://n8.io/)",
|
||||
"license": "MIT",
|
||||
"bugs": {
|
||||
"url": "https://github.com/TooTallNate/node-agent-base/issues"
|
||||
},
|
||||
"dependencies": {
|
||||
"debug": "4"
|
||||
},
|
||||
"devDependencies": {
|
||||
"@types/debug": "4",
|
||||
"@types/mocha": "^5.2.7",
|
||||
"@types/node": "^14.0.20",
|
||||
"@types/semver": "^7.1.0",
|
||||
"@types/ws": "^6.0.3",
|
||||
"@typescript-eslint/eslint-plugin": "1.6.0",
|
||||
"@typescript-eslint/parser": "1.1.0",
|
||||
"async-listen": "^1.2.0",
|
||||
"cpy-cli": "^2.0.0",
|
||||
"eslint": "5.16.0",
|
||||
"eslint-config-airbnb": "17.1.0",
|
||||
"eslint-config-prettier": "4.1.0",
|
||||
"eslint-import-resolver-typescript": "1.1.1",
|
||||
"eslint-plugin-import": "2.16.0",
|
||||
"eslint-plugin-jsx-a11y": "6.2.1",
|
||||
"eslint-plugin-react": "7.12.4",
|
||||
"mocha": "^6.2.0",
|
||||
"rimraf": "^3.0.0",
|
||||
"semver": "^7.1.2",
|
||||
"typescript": "^3.5.3",
|
||||
"ws": "^3.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 6.0.0"
|
||||
}
|
||||
}
|
|
@ -0,0 +1,345 @@
|
|||
import net from 'net';
|
||||
import http from 'http';
|
||||
import https from 'https';
|
||||
import { Duplex } from 'stream';
|
||||
import { EventEmitter } from 'events';
|
||||
import createDebug from 'debug';
|
||||
import promisify from './promisify';
|
||||
|
||||
const debug = createDebug('agent-base');
|
||||
|
||||
function isAgent(v: any): v is createAgent.AgentLike {
|
||||
return Boolean(v) && typeof v.addRequest === 'function';
|
||||
}
|
||||
|
||||
function isSecureEndpoint(): boolean {
|
||||
const { stack } = new Error();
|
||||
if (typeof stack !== 'string') return false;
|
||||
return stack.split('\n').some(l => l.indexOf('(https.js:') !== -1 || l.indexOf('node:https:') !== -1);
|
||||
}
|
||||
|
||||
function createAgent(opts?: createAgent.AgentOptions): createAgent.Agent;
|
||||
function createAgent(
|
||||
callback: createAgent.AgentCallback,
|
||||
opts?: createAgent.AgentOptions
|
||||
): createAgent.Agent;
|
||||
function createAgent(
|
||||
callback?: createAgent.AgentCallback | createAgent.AgentOptions,
|
||||
opts?: createAgent.AgentOptions
|
||||
) {
|
||||
return new createAgent.Agent(callback, opts);
|
||||
}
|
||||
|
||||
namespace createAgent {
|
||||
export interface ClientRequest extends http.ClientRequest {
|
||||
_last?: boolean;
|
||||
_hadError?: boolean;
|
||||
method: string;
|
||||
}
|
||||
|
||||
export interface AgentRequestOptions {
|
||||
host?: string;
|
||||
path?: string;
|
||||
// `port` on `http.RequestOptions` can be a string or undefined,
|
||||
// but `net.TcpNetConnectOpts` expects only a number
|
||||
port: number;
|
||||
}
|
||||
|
||||
export interface HttpRequestOptions
|
||||
extends AgentRequestOptions,
|
||||
Omit<http.RequestOptions, keyof AgentRequestOptions> {
|
||||
secureEndpoint: false;
|
||||
}
|
||||
|
||||
export interface HttpsRequestOptions
|
||||
extends AgentRequestOptions,
|
||||
Omit<https.RequestOptions, keyof AgentRequestOptions> {
|
||||
secureEndpoint: true;
|
||||
}
|
||||
|
||||
export type RequestOptions = HttpRequestOptions | HttpsRequestOptions;
|
||||
|
||||
export type AgentLike = Pick<createAgent.Agent, 'addRequest'> | http.Agent;
|
||||
|
||||
export type AgentCallbackReturn = Duplex | AgentLike;
|
||||
|
||||
export type AgentCallbackCallback = (
|
||||
err?: Error | null,
|
||||
socket?: createAgent.AgentCallbackReturn
|
||||
) => void;
|
||||
|
||||
export type AgentCallbackPromise = (
|
||||
req: createAgent.ClientRequest,
|
||||
opts: createAgent.RequestOptions
|
||||
) =>
|
||||
| createAgent.AgentCallbackReturn
|
||||
| Promise<createAgent.AgentCallbackReturn>;
|
||||
|
||||
export type AgentCallback = typeof Agent.prototype.callback;
|
||||
|
||||
export type AgentOptions = {
|
||||
timeout?: number;
|
||||
};
|
||||
|
||||
/**
|
||||
* Base `http.Agent` implementation.
|
||||
* No pooling/keep-alive is implemented by default.
|
||||
*
|
||||
* @param {Function} callback
|
||||
* @api public
|
||||
*/
|
||||
export class Agent extends EventEmitter {
|
||||
public timeout: number | null;
|
||||
public maxFreeSockets: number;
|
||||
public maxTotalSockets: number;
|
||||
public maxSockets: number;
|
||||
public sockets: {
|
||||
[key: string]: net.Socket[];
|
||||
};
|
||||
public freeSockets: {
|
||||
[key: string]: net.Socket[];
|
||||
};
|
||||
public requests: {
|
||||
[key: string]: http.IncomingMessage[];
|
||||
};
|
||||
public options: https.AgentOptions;
|
||||
private promisifiedCallback?: createAgent.AgentCallbackPromise;
|
||||
private explicitDefaultPort?: number;
|
||||
private explicitProtocol?: string;
|
||||
|
||||
constructor(
|
||||
callback?: createAgent.AgentCallback | createAgent.AgentOptions,
|
||||
_opts?: createAgent.AgentOptions
|
||||
) {
|
||||
super();
|
||||
|
||||
let opts = _opts;
|
||||
if (typeof callback === 'function') {
|
||||
this.callback = callback;
|
||||
} else if (callback) {
|
||||
opts = callback;
|
||||
}
|
||||
|
||||
// Timeout for the socket to be returned from the callback
|
||||
this.timeout = null;
|
||||
if (opts && typeof opts.timeout === 'number') {
|
||||
this.timeout = opts.timeout;
|
||||
}
|
||||
|
||||
// These aren't actually used by `agent-base`, but are required
|
||||
// for the TypeScript definition files in `@types/node` :/
|
||||
this.maxFreeSockets = 1;
|
||||
this.maxSockets = 1;
|
||||
this.maxTotalSockets = Infinity;
|
||||
this.sockets = {};
|
||||
this.freeSockets = {};
|
||||
this.requests = {};
|
||||
this.options = {};
|
||||
}
|
||||
|
||||
get defaultPort(): number {
|
||||
if (typeof this.explicitDefaultPort === 'number') {
|
||||
return this.explicitDefaultPort;
|
||||
}
|
||||
return isSecureEndpoint() ? 443 : 80;
|
||||
}
|
||||
|
||||
set defaultPort(v: number) {
|
||||
this.explicitDefaultPort = v;
|
||||
}
|
||||
|
||||
get protocol(): string {
|
||||
if (typeof this.explicitProtocol === 'string') {
|
||||
return this.explicitProtocol;
|
||||
}
|
||||
return isSecureEndpoint() ? 'https:' : 'http:';
|
||||
}
|
||||
|
||||
set protocol(v: string) {
|
||||
this.explicitProtocol = v;
|
||||
}
|
||||
|
||||
callback(
|
||||
req: createAgent.ClientRequest,
|
||||
opts: createAgent.RequestOptions,
|
||||
fn: createAgent.AgentCallbackCallback
|
||||
): void;
|
||||
callback(
|
||||
req: createAgent.ClientRequest,
|
||||
opts: createAgent.RequestOptions
|
||||
):
|
||||
| createAgent.AgentCallbackReturn
|
||||
| Promise<createAgent.AgentCallbackReturn>;
|
||||
callback(
|
||||
req: createAgent.ClientRequest,
|
||||
opts: createAgent.AgentOptions,
|
||||
fn?: createAgent.AgentCallbackCallback
|
||||
):
|
||||
| createAgent.AgentCallbackReturn
|
||||
| Promise<createAgent.AgentCallbackReturn>
|
||||
| void {
|
||||
throw new Error(
|
||||
'"agent-base" has no default implementation, you must subclass and override `callback()`'
|
||||
);
|
||||
}
|
||||
|
||||
/**
|
||||
* Called by node-core's "_http_client.js" module when creating
|
||||
* a new HTTP request with this Agent instance.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
addRequest(req: ClientRequest, _opts: RequestOptions): void {
|
||||
const opts: RequestOptions = { ..._opts };
|
||||
|
||||
if (typeof opts.secureEndpoint !== 'boolean') {
|
||||
opts.secureEndpoint = isSecureEndpoint();
|
||||
}
|
||||
|
||||
if (opts.host == null) {
|
||||
opts.host = 'localhost';
|
||||
}
|
||||
|
||||
if (opts.port == null) {
|
||||
opts.port = opts.secureEndpoint ? 443 : 80;
|
||||
}
|
||||
|
||||
if (opts.protocol == null) {
|
||||
opts.protocol = opts.secureEndpoint ? 'https:' : 'http:';
|
||||
}
|
||||
|
||||
if (opts.host && opts.path) {
|
||||
// If both a `host` and `path` are specified then it's most
|
||||
// likely the result of a `url.parse()` call... we need to
|
||||
// remove the `path` portion so that `net.connect()` doesn't
|
||||
// attempt to open that as a unix socket file.
|
||||
delete opts.path;
|
||||
}
|
||||
|
||||
delete opts.agent;
|
||||
delete opts.hostname;
|
||||
delete opts._defaultAgent;
|
||||
delete opts.defaultPort;
|
||||
delete opts.createConnection;
|
||||
|
||||
// Hint to use "Connection: close"
|
||||
// XXX: non-documented `http` module API :(
|
||||
req._last = true;
|
||||
req.shouldKeepAlive = false;
|
||||
|
||||
let timedOut = false;
|
||||
let timeoutId: ReturnType<typeof setTimeout> | null = null;
|
||||
const timeoutMs = opts.timeout || this.timeout;
|
||||
|
||||
const onerror = (err: NodeJS.ErrnoException) => {
|
||||
if (req._hadError) return;
|
||||
req.emit('error', err);
|
||||
// For Safety. Some additional errors might fire later on
|
||||
// and we need to make sure we don't double-fire the error event.
|
||||
req._hadError = true;
|
||||
};
|
||||
|
||||
const ontimeout = () => {
|
||||
timeoutId = null;
|
||||
timedOut = true;
|
||||
const err: NodeJS.ErrnoException = new Error(
|
||||
`A "socket" was not created for HTTP request before ${timeoutMs}ms`
|
||||
);
|
||||
err.code = 'ETIMEOUT';
|
||||
onerror(err);
|
||||
};
|
||||
|
||||
const callbackError = (err: NodeJS.ErrnoException) => {
|
||||
if (timedOut) return;
|
||||
if (timeoutId !== null) {
|
||||
clearTimeout(timeoutId);
|
||||
timeoutId = null;
|
||||
}
|
||||
onerror(err);
|
||||
};
|
||||
|
||||
const onsocket = (socket: AgentCallbackReturn) => {
|
||||
if (timedOut) return;
|
||||
if (timeoutId != null) {
|
||||
clearTimeout(timeoutId);
|
||||
timeoutId = null;
|
||||
}
|
||||
|
||||
if (isAgent(socket)) {
|
||||
// `socket` is actually an `http.Agent` instance, so
|
||||
// relinquish responsibility for this `req` to the Agent
|
||||
// from here on
|
||||
debug(
|
||||
'Callback returned another Agent instance %o',
|
||||
socket.constructor.name
|
||||
);
|
||||
(socket as createAgent.Agent).addRequest(req, opts);
|
||||
return;
|
||||
}
|
||||
|
||||
if (socket) {
|
||||
socket.once('free', () => {
|
||||
this.freeSocket(socket as net.Socket, opts);
|
||||
});
|
||||
req.onSocket(socket as net.Socket);
|
||||
return;
|
||||
}
|
||||
|
||||
const err = new Error(
|
||||
`no Duplex stream was returned to agent-base for \`${req.method} ${req.path}\``
|
||||
);
|
||||
onerror(err);
|
||||
};
|
||||
|
||||
if (typeof this.callback !== 'function') {
|
||||
onerror(new Error('`callback` is not defined'));
|
||||
return;
|
||||
}
|
||||
|
||||
if (!this.promisifiedCallback) {
|
||||
if (this.callback.length >= 3) {
|
||||
debug('Converting legacy callback function to promise');
|
||||
this.promisifiedCallback = promisify(this.callback);
|
||||
} else {
|
||||
this.promisifiedCallback = this.callback;
|
||||
}
|
||||
}
|
||||
|
||||
if (typeof timeoutMs === 'number' && timeoutMs > 0) {
|
||||
timeoutId = setTimeout(ontimeout, timeoutMs);
|
||||
}
|
||||
|
||||
if ('port' in opts && typeof opts.port !== 'number') {
|
||||
opts.port = Number(opts.port);
|
||||
}
|
||||
|
||||
try {
|
||||
debug(
|
||||
'Resolving socket for %o request: %o',
|
||||
opts.protocol,
|
||||
`${req.method} ${req.path}`
|
||||
);
|
||||
Promise.resolve(this.promisifiedCallback(req, opts)).then(
|
||||
onsocket,
|
||||
callbackError
|
||||
);
|
||||
} catch (err) {
|
||||
Promise.reject(err).catch(callbackError);
|
||||
}
|
||||
}
|
||||
|
||||
freeSocket(socket: net.Socket, opts: AgentOptions) {
|
||||
debug('Freeing socket %o %o', socket.constructor.name, opts);
|
||||
socket.destroy();
|
||||
}
|
||||
|
||||
destroy() {
|
||||
debug('Destroying agent %o', this.constructor.name);
|
||||
}
|
||||
}
|
||||
|
||||
// So that `instanceof` works correctly
|
||||
createAgent.prototype = createAgent.Agent.prototype;
|
||||
}
|
||||
|
||||
export = createAgent;
|
|
@ -0,0 +1,33 @@
|
|||
import {
|
||||
Agent,
|
||||
ClientRequest,
|
||||
RequestOptions,
|
||||
AgentCallbackCallback,
|
||||
AgentCallbackPromise,
|
||||
AgentCallbackReturn
|
||||
} from './index';
|
||||
|
||||
type LegacyCallback = (
|
||||
req: ClientRequest,
|
||||
opts: RequestOptions,
|
||||
fn: AgentCallbackCallback
|
||||
) => void;
|
||||
|
||||
export default function promisify(fn: LegacyCallback): AgentCallbackPromise {
|
||||
return function(this: Agent, req: ClientRequest, opts: RequestOptions) {
|
||||
return new Promise((resolve, reject) => {
|
||||
fn.call(
|
||||
this,
|
||||
req,
|
||||
opts,
|
||||
(err: Error | null | undefined, rtn?: AgentCallbackReturn) => {
|
||||
if (err) {
|
||||
reject(err);
|
||||
} else {
|
||||
resolve(rtn);
|
||||
}
|
||||
}
|
||||
);
|
||||
});
|
||||
};
|
||||
}
|
|
@ -0,0 +1,2 @@
|
|||
tidelift: "npm/balanced-match"
|
||||
patreon: juliangruber
|
|
@ -0,0 +1,21 @@
|
|||
(MIT)
|
||||
|
||||
Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
this software and associated documentation files (the "Software"), to deal in
|
||||
the Software without restriction, including without limitation the rights to
|
||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
|
||||
of the Software, and to permit persons to whom the Software is furnished to do
|
||||
so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,97 @@
|
|||
# balanced-match
|
||||
|
||||
Match balanced string pairs, like `{` and `}` or `<b>` and `</b>`. Supports regular expressions as well!
|
||||
|
||||
[](http://travis-ci.org/juliangruber/balanced-match)
|
||||
[](https://www.npmjs.org/package/balanced-match)
|
||||
|
||||
[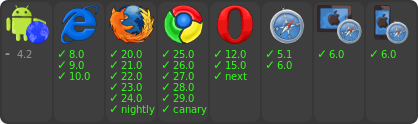](https://ci.testling.com/juliangruber/balanced-match)
|
||||
|
||||
## Example
|
||||
|
||||
Get the first matching pair of braces:
|
||||
|
||||
```js
|
||||
var balanced = require('balanced-match');
|
||||
|
||||
console.log(balanced('{', '}', 'pre{in{nested}}post'));
|
||||
console.log(balanced('{', '}', 'pre{first}between{second}post'));
|
||||
console.log(balanced(/\s+\{\s+/, /\s+\}\s+/, 'pre { in{nest} } post'));
|
||||
```
|
||||
|
||||
The matches are:
|
||||
|
||||
```bash
|
||||
$ node example.js
|
||||
{ start: 3, end: 14, pre: 'pre', body: 'in{nested}', post: 'post' }
|
||||
{ start: 3,
|
||||
end: 9,
|
||||
pre: 'pre',
|
||||
body: 'first',
|
||||
post: 'between{second}post' }
|
||||
{ start: 3, end: 17, pre: 'pre', body: 'in{nest}', post: 'post' }
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
### var m = balanced(a, b, str)
|
||||
|
||||
For the first non-nested matching pair of `a` and `b` in `str`, return an
|
||||
object with those keys:
|
||||
|
||||
* **start** the index of the first match of `a`
|
||||
* **end** the index of the matching `b`
|
||||
* **pre** the preamble, `a` and `b` not included
|
||||
* **body** the match, `a` and `b` not included
|
||||
* **post** the postscript, `a` and `b` not included
|
||||
|
||||
If there's no match, `undefined` will be returned.
|
||||
|
||||
If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `['{', 'a', '']` and `{a}}` will match `['', 'a', '}']`.
|
||||
|
||||
### var r = balanced.range(a, b, str)
|
||||
|
||||
For the first non-nested matching pair of `a` and `b` in `str`, return an
|
||||
array with indexes: `[ <a index>, <b index> ]`.
|
||||
|
||||
If there's no match, `undefined` will be returned.
|
||||
|
||||
If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `[ 1, 3 ]` and `{a}}` will match `[0, 2]`.
|
||||
|
||||
## Installation
|
||||
|
||||
With [npm](https://npmjs.org) do:
|
||||
|
||||
```bash
|
||||
npm install balanced-match
|
||||
```
|
||||
|
||||
## Security contact information
|
||||
|
||||
To report a security vulnerability, please use the
|
||||
[Tidelift security contact](https://tidelift.com/security).
|
||||
Tidelift will coordinate the fix and disclosure.
|
||||
|
||||
## License
|
||||
|
||||
(MIT)
|
||||
|
||||
Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
this software and associated documentation files (the "Software"), to deal in
|
||||
the Software without restriction, including without limitation the rights to
|
||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
|
||||
of the Software, and to permit persons to whom the Software is furnished to do
|
||||
so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,62 @@
|
|||
'use strict';
|
||||
module.exports = balanced;
|
||||
function balanced(a, b, str) {
|
||||
if (a instanceof RegExp) a = maybeMatch(a, str);
|
||||
if (b instanceof RegExp) b = maybeMatch(b, str);
|
||||
|
||||
var r = range(a, b, str);
|
||||
|
||||
return r && {
|
||||
start: r[0],
|
||||
end: r[1],
|
||||
pre: str.slice(0, r[0]),
|
||||
body: str.slice(r[0] + a.length, r[1]),
|
||||
post: str.slice(r[1] + b.length)
|
||||
};
|
||||
}
|
||||
|
||||
function maybeMatch(reg, str) {
|
||||
var m = str.match(reg);
|
||||
return m ? m[0] : null;
|
||||
}
|
||||
|
||||
balanced.range = range;
|
||||
function range(a, b, str) {
|
||||
var begs, beg, left, right, result;
|
||||
var ai = str.indexOf(a);
|
||||
var bi = str.indexOf(b, ai + 1);
|
||||
var i = ai;
|
||||
|
||||
if (ai >= 0 && bi > 0) {
|
||||
if(a===b) {
|
||||
return [ai, bi];
|
||||
}
|
||||
begs = [];
|
||||
left = str.length;
|
||||
|
||||
while (i >= 0 && !result) {
|
||||
if (i == ai) {
|
||||
begs.push(i);
|
||||
ai = str.indexOf(a, i + 1);
|
||||
} else if (begs.length == 1) {
|
||||
result = [ begs.pop(), bi ];
|
||||
} else {
|
||||
beg = begs.pop();
|
||||
if (beg < left) {
|
||||
left = beg;
|
||||
right = bi;
|
||||
}
|
||||
|
||||
bi = str.indexOf(b, i + 1);
|
||||
}
|
||||
|
||||
i = ai < bi && ai >= 0 ? ai : bi;
|
||||
}
|
||||
|
||||
if (begs.length) {
|
||||
result = [ left, right ];
|
||||
}
|
||||
}
|
||||
|
||||
return result;
|
||||
}
|
|
@ -0,0 +1,48 @@
|
|||
{
|
||||
"name": "balanced-match",
|
||||
"description": "Match balanced character pairs, like \"{\" and \"}\"",
|
||||
"version": "1.0.2",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/juliangruber/balanced-match.git"
|
||||
},
|
||||
"homepage": "https://github.com/juliangruber/balanced-match",
|
||||
"main": "index.js",
|
||||
"scripts": {
|
||||
"test": "tape test/test.js",
|
||||
"bench": "matcha test/bench.js"
|
||||
},
|
||||
"devDependencies": {
|
||||
"matcha": "^0.7.0",
|
||||
"tape": "^4.6.0"
|
||||
},
|
||||
"keywords": [
|
||||
"match",
|
||||
"regexp",
|
||||
"test",
|
||||
"balanced",
|
||||
"parse"
|
||||
],
|
||||
"author": {
|
||||
"name": "Julian Gruber",
|
||||
"email": "mail@juliangruber.com",
|
||||
"url": "http://juliangruber.com"
|
||||
},
|
||||
"license": "MIT",
|
||||
"testling": {
|
||||
"files": "test/*.js",
|
||||
"browsers": [
|
||||
"ie/8..latest",
|
||||
"firefox/20..latest",
|
||||
"firefox/nightly",
|
||||
"chrome/25..latest",
|
||||
"chrome/canary",
|
||||
"opera/12..latest",
|
||||
"opera/next",
|
||||
"safari/5.1..latest",
|
||||
"ipad/6.0..latest",
|
||||
"iphone/6.0..latest",
|
||||
"android-browser/4.2..latest"
|
||||
]
|
||||
}
|
||||
}
|
|
@ -0,0 +1,21 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,129 @@
|
|||
# brace-expansion
|
||||
|
||||
[Brace expansion](https://www.gnu.org/software/bash/manual/html_node/Brace-Expansion.html),
|
||||
as known from sh/bash, in JavaScript.
|
||||
|
||||
[](http://travis-ci.org/juliangruber/brace-expansion)
|
||||
[](https://www.npmjs.org/package/brace-expansion)
|
||||
[](https://greenkeeper.io/)
|
||||
|
||||
[](https://ci.testling.com/juliangruber/brace-expansion)
|
||||
|
||||
## Example
|
||||
|
||||
```js
|
||||
var expand = require('brace-expansion');
|
||||
|
||||
expand('file-{a,b,c}.jpg')
|
||||
// => ['file-a.jpg', 'file-b.jpg', 'file-c.jpg']
|
||||
|
||||
expand('-v{,,}')
|
||||
// => ['-v', '-v', '-v']
|
||||
|
||||
expand('file{0..2}.jpg')
|
||||
// => ['file0.jpg', 'file1.jpg', 'file2.jpg']
|
||||
|
||||
expand('file-{a..c}.jpg')
|
||||
// => ['file-a.jpg', 'file-b.jpg', 'file-c.jpg']
|
||||
|
||||
expand('file{2..0}.jpg')
|
||||
// => ['file2.jpg', 'file1.jpg', 'file0.jpg']
|
||||
|
||||
expand('file{0..4..2}.jpg')
|
||||
// => ['file0.jpg', 'file2.jpg', 'file4.jpg']
|
||||
|
||||
expand('file-{a..e..2}.jpg')
|
||||
// => ['file-a.jpg', 'file-c.jpg', 'file-e.jpg']
|
||||
|
||||
expand('file{00..10..5}.jpg')
|
||||
// => ['file00.jpg', 'file05.jpg', 'file10.jpg']
|
||||
|
||||
expand('{{A..C},{a..c}}')
|
||||
// => ['A', 'B', 'C', 'a', 'b', 'c']
|
||||
|
||||
expand('ppp{,config,oe{,conf}}')
|
||||
// => ['ppp', 'pppconfig', 'pppoe', 'pppoeconf']
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
```js
|
||||
var expand = require('brace-expansion');
|
||||
```
|
||||
|
||||
### var expanded = expand(str)
|
||||
|
||||
Return an array of all possible and valid expansions of `str`. If none are
|
||||
found, `[str]` is returned.
|
||||
|
||||
Valid expansions are:
|
||||
|
||||
```js
|
||||
/^(.*,)+(.+)?$/
|
||||
// {a,b,...}
|
||||
```
|
||||
|
||||
A comma separated list of options, like `{a,b}` or `{a,{b,c}}` or `{,a,}`.
|
||||
|
||||
```js
|
||||
/^-?\d+\.\.-?\d+(\.\.-?\d+)?$/
|
||||
// {x..y[..incr]}
|
||||
```
|
||||
|
||||
A numeric sequence from `x` to `y` inclusive, with optional increment.
|
||||
If `x` or `y` start with a leading `0`, all the numbers will be padded
|
||||
to have equal length. Negative numbers and backwards iteration work too.
|
||||
|
||||
```js
|
||||
/^-?\d+\.\.-?\d+(\.\.-?\d+)?$/
|
||||
// {x..y[..incr]}
|
||||
```
|
||||
|
||||
An alphabetic sequence from `x` to `y` inclusive, with optional increment.
|
||||
`x` and `y` must be exactly one character, and if given, `incr` must be a
|
||||
number.
|
||||
|
||||
For compatibility reasons, the string `${` is not eligible for brace expansion.
|
||||
|
||||
## Installation
|
||||
|
||||
With [npm](https://npmjs.org) do:
|
||||
|
||||
```bash
|
||||
npm install brace-expansion
|
||||
```
|
||||
|
||||
## Contributors
|
||||
|
||||
- [Julian Gruber](https://github.com/juliangruber)
|
||||
- [Isaac Z. Schlueter](https://github.com/isaacs)
|
||||
|
||||
## Sponsors
|
||||
|
||||
This module is proudly supported by my [Sponsors](https://github.com/juliangruber/sponsors)!
|
||||
|
||||
Do you want to support modules like this to improve their quality, stability and weigh in on new features? Then please consider donating to my [Patreon](https://www.patreon.com/juliangruber). Not sure how much of my modules you're using? Try [feross/thanks](https://github.com/feross/thanks)!
|
||||
|
||||
## License
|
||||
|
||||
(MIT)
|
||||
|
||||
Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
this software and associated documentation files (the "Software"), to deal in
|
||||
the Software without restriction, including without limitation the rights to
|
||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
|
||||
of the Software, and to permit persons to whom the Software is furnished to do
|
||||
so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,201 @@
|
|||
var concatMap = require('concat-map');
|
||||
var balanced = require('balanced-match');
|
||||
|
||||
module.exports = expandTop;
|
||||
|
||||
var escSlash = '\0SLASH'+Math.random()+'\0';
|
||||
var escOpen = '\0OPEN'+Math.random()+'\0';
|
||||
var escClose = '\0CLOSE'+Math.random()+'\0';
|
||||
var escComma = '\0COMMA'+Math.random()+'\0';
|
||||
var escPeriod = '\0PERIOD'+Math.random()+'\0';
|
||||
|
||||
function numeric(str) {
|
||||
return parseInt(str, 10) == str
|
||||
? parseInt(str, 10)
|
||||
: str.charCodeAt(0);
|
||||
}
|
||||
|
||||
function escapeBraces(str) {
|
||||
return str.split('\\\\').join(escSlash)
|
||||
.split('\\{').join(escOpen)
|
||||
.split('\\}').join(escClose)
|
||||
.split('\\,').join(escComma)
|
||||
.split('\\.').join(escPeriod);
|
||||
}
|
||||
|
||||
function unescapeBraces(str) {
|
||||
return str.split(escSlash).join('\\')
|
||||
.split(escOpen).join('{')
|
||||
.split(escClose).join('}')
|
||||
.split(escComma).join(',')
|
||||
.split(escPeriod).join('.');
|
||||
}
|
||||
|
||||
|
||||
// Basically just str.split(","), but handling cases
|
||||
// where we have nested braced sections, which should be
|
||||
// treated as individual members, like {a,{b,c},d}
|
||||
function parseCommaParts(str) {
|
||||
if (!str)
|
||||
return [''];
|
||||
|
||||
var parts = [];
|
||||
var m = balanced('{', '}', str);
|
||||
|
||||
if (!m)
|
||||
return str.split(',');
|
||||
|
||||
var pre = m.pre;
|
||||
var body = m.body;
|
||||
var post = m.post;
|
||||
var p = pre.split(',');
|
||||
|
||||
p[p.length-1] += '{' + body + '}';
|
||||
var postParts = parseCommaParts(post);
|
||||
if (post.length) {
|
||||
p[p.length-1] += postParts.shift();
|
||||
p.push.apply(p, postParts);
|
||||
}
|
||||
|
||||
parts.push.apply(parts, p);
|
||||
|
||||
return parts;
|
||||
}
|
||||
|
||||
function expandTop(str) {
|
||||
if (!str)
|
||||
return [];
|
||||
|
||||
// I don't know why Bash 4.3 does this, but it does.
|
||||
// Anything starting with {} will have the first two bytes preserved
|
||||
// but *only* at the top level, so {},a}b will not expand to anything,
|
||||
// but a{},b}c will be expanded to [a}c,abc].
|
||||
// One could argue that this is a bug in Bash, but since the goal of
|
||||
// this module is to match Bash's rules, we escape a leading {}
|
||||
if (str.substr(0, 2) === '{}') {
|
||||
str = '\\{\\}' + str.substr(2);
|
||||
}
|
||||
|
||||
return expand(escapeBraces(str), true).map(unescapeBraces);
|
||||
}
|
||||
|
||||
function identity(e) {
|
||||
return e;
|
||||
}
|
||||
|
||||
function embrace(str) {
|
||||
return '{' + str + '}';
|
||||
}
|
||||
function isPadded(el) {
|
||||
return /^-?0\d/.test(el);
|
||||
}
|
||||
|
||||
function lte(i, y) {
|
||||
return i <= y;
|
||||
}
|
||||
function gte(i, y) {
|
||||
return i >= y;
|
||||
}
|
||||
|
||||
function expand(str, isTop) {
|
||||
var expansions = [];
|
||||
|
||||
var m = balanced('{', '}', str);
|
||||
if (!m || /\$$/.test(m.pre)) return [str];
|
||||
|
||||
var isNumericSequence = /^-?\d+\.\.-?\d+(?:\.\.-?\d+)?$/.test(m.body);
|
||||
var isAlphaSequence = /^[a-zA-Z]\.\.[a-zA-Z](?:\.\.-?\d+)?$/.test(m.body);
|
||||
var isSequence = isNumericSequence || isAlphaSequence;
|
||||
var isOptions = m.body.indexOf(',') >= 0;
|
||||
if (!isSequence && !isOptions) {
|
||||
// {a},b}
|
||||
if (m.post.match(/,.*\}/)) {
|
||||
str = m.pre + '{' + m.body + escClose + m.post;
|
||||
return expand(str);
|
||||
}
|
||||
return [str];
|
||||
}
|
||||
|
||||
var n;
|
||||
if (isSequence) {
|
||||
n = m.body.split(/\.\./);
|
||||
} else {
|
||||
n = parseCommaParts(m.body);
|
||||
if (n.length === 1) {
|
||||
// x{{a,b}}y ==> x{a}y x{b}y
|
||||
n = expand(n[0], false).map(embrace);
|
||||
if (n.length === 1) {
|
||||
var post = m.post.length
|
||||
? expand(m.post, false)
|
||||
: [''];
|
||||
return post.map(function(p) {
|
||||
return m.pre + n[0] + p;
|
||||
});
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
// at this point, n is the parts, and we know it's not a comma set
|
||||
// with a single entry.
|
||||
|
||||
// no need to expand pre, since it is guaranteed to be free of brace-sets
|
||||
var pre = m.pre;
|
||||
var post = m.post.length
|
||||
? expand(m.post, false)
|
||||
: [''];
|
||||
|
||||
var N;
|
||||
|
||||
if (isSequence) {
|
||||
var x = numeric(n[0]);
|
||||
var y = numeric(n[1]);
|
||||
var width = Math.max(n[0].length, n[1].length)
|
||||
var incr = n.length == 3
|
||||
? Math.abs(numeric(n[2]))
|
||||
: 1;
|
||||
var test = lte;
|
||||
var reverse = y < x;
|
||||
if (reverse) {
|
||||
incr *= -1;
|
||||
test = gte;
|
||||
}
|
||||
var pad = n.some(isPadded);
|
||||
|
||||
N = [];
|
||||
|
||||
for (var i = x; test(i, y); i += incr) {
|
||||
var c;
|
||||
if (isAlphaSequence) {
|
||||
c = String.fromCharCode(i);
|
||||
if (c === '\\')
|
||||
c = '';
|
||||
} else {
|
||||
c = String(i);
|
||||
if (pad) {
|
||||
var need = width - c.length;
|
||||
if (need > 0) {
|
||||
var z = new Array(need + 1).join('0');
|
||||
if (i < 0)
|
||||
c = '-' + z + c.slice(1);
|
||||
else
|
||||
c = z + c;
|
||||
}
|
||||
}
|
||||
}
|
||||
N.push(c);
|
||||
}
|
||||
} else {
|
||||
N = concatMap(n, function(el) { return expand(el, false) });
|
||||
}
|
||||
|
||||
for (var j = 0; j < N.length; j++) {
|
||||
for (var k = 0; k < post.length; k++) {
|
||||
var expansion = pre + N[j] + post[k];
|
||||
if (!isTop || isSequence || expansion)
|
||||
expansions.push(expansion);
|
||||
}
|
||||
}
|
||||
|
||||
return expansions;
|
||||
}
|
||||
|
|
@ -0,0 +1,47 @@
|
|||
{
|
||||
"name": "brace-expansion",
|
||||
"description": "Brace expansion as known from sh/bash",
|
||||
"version": "1.1.11",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/juliangruber/brace-expansion.git"
|
||||
},
|
||||
"homepage": "https://github.com/juliangruber/brace-expansion",
|
||||
"main": "index.js",
|
||||
"scripts": {
|
||||
"test": "tape test/*.js",
|
||||
"gentest": "bash test/generate.sh",
|
||||
"bench": "matcha test/perf/bench.js"
|
||||
},
|
||||
"dependencies": {
|
||||
"balanced-match": "^1.0.0",
|
||||
"concat-map": "0.0.1"
|
||||
},
|
||||
"devDependencies": {
|
||||
"matcha": "^0.7.0",
|
||||
"tape": "^4.6.0"
|
||||
},
|
||||
"keywords": [],
|
||||
"author": {
|
||||
"name": "Julian Gruber",
|
||||
"email": "mail@juliangruber.com",
|
||||
"url": "http://juliangruber.com"
|
||||
},
|
||||
"license": "MIT",
|
||||
"testling": {
|
||||
"files": "test/*.js",
|
||||
"browsers": [
|
||||
"ie/8..latest",
|
||||
"firefox/20..latest",
|
||||
"firefox/nightly",
|
||||
"chrome/25..latest",
|
||||
"chrome/canary",
|
||||
"opera/12..latest",
|
||||
"opera/next",
|
||||
"safari/5.1..latest",
|
||||
"ipad/6.0..latest",
|
||||
"iphone/6.0..latest",
|
||||
"android-browser/4.2..latest"
|
||||
]
|
||||
}
|
||||
}
|
|
@ -0,0 +1,5 @@
|
|||
Copyright 2018 kumavis
|
||||
|
||||
Permission to use, copy, modify, and/or distribute this software for any purpose with or without fee is hereby granted, provided that the above copyright notice and this permission notice appear in all copies.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
|
|
@ -0,0 +1,40 @@
|
|||
### wat?
|
||||
|
||||
`process.stdout` in your browser.
|
||||
|
||||
### wai?
|
||||
|
||||
iono. cuz hakz.
|
||||
|
||||
### hau?
|
||||
|
||||
```js
|
||||
var BrowserStdout = require('browser-stdout')
|
||||
|
||||
myStream.pipe(BrowserStdout())
|
||||
```
|
||||
|
||||
### monkey
|
||||
|
||||
You can monkey-patch `process.stdout` for your dependency graph like this:
|
||||
|
||||
```
|
||||
process.stdout = require('browser-stdout')()
|
||||
var coolTool = require('module-that-uses-stdout-somewhere-in-its-depths')
|
||||
```
|
||||
|
||||
### opts
|
||||
|
||||
opts are passed directly to `stream.Writable`.
|
||||
additionally, a label arg can be used to label console output.
|
||||
|
||||
```js
|
||||
BrowserStdout({
|
||||
objectMode: true,
|
||||
label: 'dataz',
|
||||
})
|
||||
```
|
||||
|
||||
### ur doin it rong
|
||||
|
||||
i accept pr's.
|
|
@ -0,0 +1,25 @@
|
|||
var WritableStream = require('stream').Writable
|
||||
var inherits = require('util').inherits
|
||||
|
||||
module.exports = BrowserStdout
|
||||
|
||||
|
||||
inherits(BrowserStdout, WritableStream)
|
||||
|
||||
function BrowserStdout(opts) {
|
||||
if (!(this instanceof BrowserStdout)) return new BrowserStdout(opts)
|
||||
|
||||
opts = opts || {}
|
||||
WritableStream.call(this, opts)
|
||||
this.label = (opts.label !== undefined) ? opts.label : 'stdout'
|
||||
}
|
||||
|
||||
BrowserStdout.prototype._write = function(chunks, encoding, cb) {
|
||||
var output = chunks.toString ? chunks.toString() : chunks
|
||||
if (this.label === false) {
|
||||
console.log(output)
|
||||
} else {
|
||||
console.log(this.label+':', output)
|
||||
}
|
||||
process.nextTick(cb)
|
||||
}
|
|
@ -0,0 +1,15 @@
|
|||
{
|
||||
"name": "browser-stdout",
|
||||
"version": "1.3.1",
|
||||
"description": "",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "http://github.com/kumavis/browser-stdout.git"
|
||||
},
|
||||
"main": "index.js",
|
||||
"scripts": {
|
||||
"test": "echo \"Error: no test specified\" && exit 1"
|
||||
},
|
||||
"author": "kumavis",
|
||||
"license": "ISC"
|
||||
}
|
|
@ -0,0 +1,21 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2016, 2018 Linus Unnebäck
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,72 @@
|
|||
/* eslint-disable node/no-deprecated-api */
|
||||
|
||||
var toString = Object.prototype.toString
|
||||
|
||||
var isModern = (
|
||||
typeof Buffer !== 'undefined' &&
|
||||
typeof Buffer.alloc === 'function' &&
|
||||
typeof Buffer.allocUnsafe === 'function' &&
|
||||
typeof Buffer.from === 'function'
|
||||
)
|
||||
|
||||
function isArrayBuffer (input) {
|
||||
return toString.call(input).slice(8, -1) === 'ArrayBuffer'
|
||||
}
|
||||
|
||||
function fromArrayBuffer (obj, byteOffset, length) {
|
||||
byteOffset >>>= 0
|
||||
|
||||
var maxLength = obj.byteLength - byteOffset
|
||||
|
||||
if (maxLength < 0) {
|
||||
throw new RangeError("'offset' is out of bounds")
|
||||
}
|
||||
|
||||
if (length === undefined) {
|
||||
length = maxLength
|
||||
} else {
|
||||
length >>>= 0
|
||||
|
||||
if (length > maxLength) {
|
||||
throw new RangeError("'length' is out of bounds")
|
||||
}
|
||||
}
|
||||
|
||||
return isModern
|
||||
? Buffer.from(obj.slice(byteOffset, byteOffset + length))
|
||||
: new Buffer(new Uint8Array(obj.slice(byteOffset, byteOffset + length)))
|
||||
}
|
||||
|
||||
function fromString (string, encoding) {
|
||||
if (typeof encoding !== 'string' || encoding === '') {
|
||||
encoding = 'utf8'
|
||||
}
|
||||
|
||||
if (!Buffer.isEncoding(encoding)) {
|
||||
throw new TypeError('"encoding" must be a valid string encoding')
|
||||
}
|
||||
|
||||
return isModern
|
||||
? Buffer.from(string, encoding)
|
||||
: new Buffer(string, encoding)
|
||||
}
|
||||
|
||||
function bufferFrom (value, encodingOrOffset, length) {
|
||||
if (typeof value === 'number') {
|
||||
throw new TypeError('"value" argument must not be a number')
|
||||
}
|
||||
|
||||
if (isArrayBuffer(value)) {
|
||||
return fromArrayBuffer(value, encodingOrOffset, length)
|
||||
}
|
||||
|
||||
if (typeof value === 'string') {
|
||||
return fromString(value, encodingOrOffset)
|
||||
}
|
||||
|
||||
return isModern
|
||||
? Buffer.from(value)
|
||||
: new Buffer(value)
|
||||
}
|
||||
|
||||
module.exports = bufferFrom
|
|
@ -0,0 +1,19 @@
|
|||
{
|
||||
"name": "buffer-from",
|
||||
"version": "1.1.2",
|
||||
"license": "MIT",
|
||||
"repository": "LinusU/buffer-from",
|
||||
"files": [
|
||||
"index.js"
|
||||
],
|
||||
"scripts": {
|
||||
"test": "standard && node test"
|
||||
},
|
||||
"devDependencies": {
|
||||
"standard": "^12.0.1"
|
||||
},
|
||||
"keywords": [
|
||||
"buffer",
|
||||
"buffer from"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,69 @@
|
|||
# Buffer From
|
||||
|
||||
A [ponyfill](https://ponyfill.com) for `Buffer.from`, uses native implementation if available.
|
||||
|
||||
## Installation
|
||||
|
||||
```sh
|
||||
npm install --save buffer-from
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
const bufferFrom = require('buffer-from')
|
||||
|
||||
console.log(bufferFrom([1, 2, 3, 4]))
|
||||
//=> <Buffer 01 02 03 04>
|
||||
|
||||
const arr = new Uint8Array([1, 2, 3, 4])
|
||||
console.log(bufferFrom(arr.buffer, 1, 2))
|
||||
//=> <Buffer 02 03>
|
||||
|
||||
console.log(bufferFrom('test', 'utf8'))
|
||||
//=> <Buffer 74 65 73 74>
|
||||
|
||||
const buf = bufferFrom('test')
|
||||
console.log(bufferFrom(buf))
|
||||
//=> <Buffer 74 65 73 74>
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
### bufferFrom(array)
|
||||
|
||||
- `array` <Array>
|
||||
|
||||
Allocates a new `Buffer` using an `array` of octets.
|
||||
|
||||
### bufferFrom(arrayBuffer[, byteOffset[, length]])
|
||||
|
||||
- `arrayBuffer` <ArrayBuffer> The `.buffer` property of a TypedArray or ArrayBuffer
|
||||
- `byteOffset` <Integer> Where to start copying from `arrayBuffer`. **Default:** `0`
|
||||
- `length` <Integer> How many bytes to copy from `arrayBuffer`. **Default:** `arrayBuffer.length - byteOffset`
|
||||
|
||||
When passed a reference to the `.buffer` property of a TypedArray instance, the
|
||||
newly created `Buffer` will share the same allocated memory as the TypedArray.
|
||||
|
||||
The optional `byteOffset` and `length` arguments specify a memory range within
|
||||
the `arrayBuffer` that will be shared by the `Buffer`.
|
||||
|
||||
### bufferFrom(buffer)
|
||||
|
||||
- `buffer` <Buffer> An existing `Buffer` to copy data from
|
||||
|
||||
Copies the passed `buffer` data onto a new `Buffer` instance.
|
||||
|
||||
### bufferFrom(string[, encoding])
|
||||
|
||||
- `string` <String> A string to encode.
|
||||
- `encoding` <String> The encoding of `string`. **Default:** `'utf8'`
|
||||
|
||||
Creates a new `Buffer` containing the given JavaScript string `string`. If
|
||||
provided, the `encoding` parameter identifies the character encoding of
|
||||
`string`.
|
||||
|
||||
## See also
|
||||
|
||||
- [buffer-alloc](https://github.com/LinusU/buffer-alloc) A ponyfill for `Buffer.alloc`
|
||||
- [buffer-alloc-unsafe](https://github.com/LinusU/buffer-alloc-unsafe) A ponyfill for `Buffer.allocUnsafe`
|
|
@ -0,0 +1,356 @@
|
|||
|
||||
2.15.0 / 2018-03-07
|
||||
==================
|
||||
|
||||
* Update downloads badge to point to graph of downloads over time instead of duplicating link to npm
|
||||
* Arguments description
|
||||
|
||||
2.14.1 / 2018-02-07
|
||||
==================
|
||||
|
||||
* Fix typing of help function
|
||||
|
||||
2.14.0 / 2018-02-05
|
||||
==================
|
||||
|
||||
* only register the option:version event once
|
||||
* Fixes issue #727: Passing empty string for option on command is set to undefined
|
||||
* enable eqeqeq rule
|
||||
* resolves #754 add linter configuration to project
|
||||
* resolves #560 respect custom name for version option
|
||||
* document how to override the version flag
|
||||
* document using options per command
|
||||
|
||||
2.13.0 / 2018-01-09
|
||||
==================
|
||||
|
||||
* Do not print default for --no-
|
||||
* remove trailing spaces in command help
|
||||
* Update CI's Node.js to LTS and latest version
|
||||
* typedefs: Command and Option types added to commander namespace
|
||||
|
||||
2.12.2 / 2017-11-28
|
||||
==================
|
||||
|
||||
* fix: typings are not shipped
|
||||
|
||||
2.12.1 / 2017-11-23
|
||||
==================
|
||||
|
||||
* Move @types/node to dev dependency
|
||||
|
||||
2.12.0 / 2017-11-22
|
||||
==================
|
||||
|
||||
* add attributeName() method to Option objects
|
||||
* Documentation updated for options with --no prefix
|
||||
* typings: `outputHelp` takes a string as the first parameter
|
||||
* typings: use overloads
|
||||
* feat(typings): update to match js api
|
||||
* Print default value in option help
|
||||
* Fix translation error
|
||||
* Fail when using same command and alias (#491)
|
||||
* feat(typings): add help callback
|
||||
* fix bug when description is add after command with options (#662)
|
||||
* Format js code
|
||||
* Rename History.md to CHANGELOG.md (#668)
|
||||
* feat(typings): add typings to support TypeScript (#646)
|
||||
* use current node
|
||||
|
||||
2.11.0 / 2017-07-03
|
||||
==================
|
||||
|
||||
* Fix help section order and padding (#652)
|
||||
* feature: support for signals to subcommands (#632)
|
||||
* Fixed #37, --help should not display first (#447)
|
||||
* Fix translation errors. (#570)
|
||||
* Add package-lock.json
|
||||
* Remove engines
|
||||
* Upgrade package version
|
||||
* Prefix events to prevent conflicts between commands and options (#494)
|
||||
* Removing dependency on graceful-readlink
|
||||
* Support setting name in #name function and make it chainable
|
||||
* Add .vscode directory to .gitignore (Visual Studio Code metadata)
|
||||
* Updated link to ruby commander in readme files
|
||||
|
||||
2.10.0 / 2017-06-19
|
||||
==================
|
||||
|
||||
* Update .travis.yml. drop support for older node.js versions.
|
||||
* Fix require arguments in README.md
|
||||
* On SemVer you do not start from 0.0.1
|
||||
* Add missing semi colon in readme
|
||||
* Add save param to npm install
|
||||
* node v6 travis test
|
||||
* Update Readme_zh-CN.md
|
||||
* Allow literal '--' to be passed-through as an argument
|
||||
* Test subcommand alias help
|
||||
* link build badge to master branch
|
||||
* Support the alias of Git style sub-command
|
||||
* added keyword commander for better search result on npm
|
||||
* Fix Sub-Subcommands
|
||||
* test node.js stable
|
||||
* Fixes TypeError when a command has an option called `--description`
|
||||
* Update README.md to make it beginner friendly and elaborate on the difference between angled and square brackets.
|
||||
* Add chinese Readme file
|
||||
|
||||
2.9.0 / 2015-10-13
|
||||
==================
|
||||
|
||||
* Add option `isDefault` to set default subcommand #415 @Qix-
|
||||
* Add callback to allow filtering or post-processing of help text #434 @djulien
|
||||
* Fix `undefined` text in help information close #414 #416 @zhiyelee
|
||||
|
||||
2.8.1 / 2015-04-22
|
||||
==================
|
||||
|
||||
* Back out `support multiline description` Close #396 #397
|
||||
|
||||
2.8.0 / 2015-04-07
|
||||
==================
|
||||
|
||||
* Add `process.execArg` support, execution args like `--harmony` will be passed to sub-commands #387 @DigitalIO @zhiyelee
|
||||
* Fix bug in Git-style sub-commands #372 @zhiyelee
|
||||
* Allow commands to be hidden from help #383 @tonylukasavage
|
||||
* When git-style sub-commands are in use, yet none are called, display help #382 @claylo
|
||||
* Add ability to specify arguments syntax for top-level command #258 @rrthomas
|
||||
* Support multiline descriptions #208 @zxqfox
|
||||
|
||||
2.7.1 / 2015-03-11
|
||||
==================
|
||||
|
||||
* Revert #347 (fix collisions when option and first arg have same name) which causes a bug in #367.
|
||||
|
||||
2.7.0 / 2015-03-09
|
||||
==================
|
||||
|
||||
* Fix git-style bug when installed globally. Close #335 #349 @zhiyelee
|
||||
* Fix collisions when option and first arg have same name. Close #346 #347 @tonylukasavage
|
||||
* Add support for camelCase on `opts()`. Close #353 @nkzawa
|
||||
* Add node.js 0.12 and io.js to travis.yml
|
||||
* Allow RegEx options. #337 @palanik
|
||||
* Fixes exit code when sub-command failing. Close #260 #332 @pirelenito
|
||||
* git-style `bin` files in $PATH make sense. Close #196 #327 @zhiyelee
|
||||
|
||||
2.6.0 / 2014-12-30
|
||||
==================
|
||||
|
||||
* added `Command#allowUnknownOption` method. Close #138 #318 @doozr @zhiyelee
|
||||
* Add application description to the help msg. Close #112 @dalssoft
|
||||
|
||||
2.5.1 / 2014-12-15
|
||||
==================
|
||||
|
||||
* fixed two bugs incurred by variadic arguments. Close #291 @Quentin01 #302 @zhiyelee
|
||||
|
||||
2.5.0 / 2014-10-24
|
||||
==================
|
||||
|
||||
* add support for variadic arguments. Closes #277 @whitlockjc
|
||||
|
||||
2.4.0 / 2014-10-17
|
||||
==================
|
||||
|
||||
* fixed a bug on executing the coercion function of subcommands option. Closes #270
|
||||
* added `Command.prototype.name` to retrieve command name. Closes #264 #266 @tonylukasavage
|
||||
* added `Command.prototype.opts` to retrieve all the options as a simple object of key-value pairs. Closes #262 @tonylukasavage
|
||||
* fixed a bug on subcommand name. Closes #248 @jonathandelgado
|
||||
* fixed function normalize doesn’t honor option terminator. Closes #216 @abbr
|
||||
|
||||
2.3.0 / 2014-07-16
|
||||
==================
|
||||
|
||||
* add command alias'. Closes PR #210
|
||||
* fix: Typos. Closes #99
|
||||
* fix: Unused fs module. Closes #217
|
||||
|
||||
2.2.0 / 2014-03-29
|
||||
==================
|
||||
|
||||
* add passing of previous option value
|
||||
* fix: support subcommands on windows. Closes #142
|
||||
* Now the defaultValue passed as the second argument of the coercion function.
|
||||
|
||||
2.1.0 / 2013-11-21
|
||||
==================
|
||||
|
||||
* add: allow cflag style option params, unit test, fixes #174
|
||||
|
||||
2.0.0 / 2013-07-18
|
||||
==================
|
||||
|
||||
* remove input methods (.prompt, .confirm, etc)
|
||||
|
||||
1.3.2 / 2013-07-18
|
||||
==================
|
||||
|
||||
* add support for sub-commands to co-exist with the original command
|
||||
|
||||
1.3.1 / 2013-07-18
|
||||
==================
|
||||
|
||||
* add quick .runningCommand hack so you can opt-out of other logic when running a sub command
|
||||
|
||||
1.3.0 / 2013-07-09
|
||||
==================
|
||||
|
||||
* add EACCES error handling
|
||||
* fix sub-command --help
|
||||
|
||||
1.2.0 / 2013-06-13
|
||||
==================
|
||||
|
||||
* allow "-" hyphen as an option argument
|
||||
* support for RegExp coercion
|
||||
|
||||
1.1.1 / 2012-11-20
|
||||
==================
|
||||
|
||||
* add more sub-command padding
|
||||
* fix .usage() when args are present. Closes #106
|
||||
|
||||
1.1.0 / 2012-11-16
|
||||
==================
|
||||
|
||||
* add git-style executable subcommand support. Closes #94
|
||||
|
||||
1.0.5 / 2012-10-09
|
||||
==================
|
||||
|
||||
* fix `--name` clobbering. Closes #92
|
||||
* fix examples/help. Closes #89
|
||||
|
||||
1.0.4 / 2012-09-03
|
||||
==================
|
||||
|
||||
* add `outputHelp()` method.
|
||||
|
||||
1.0.3 / 2012-08-30
|
||||
==================
|
||||
|
||||
* remove invalid .version() defaulting
|
||||
|
||||
1.0.2 / 2012-08-24
|
||||
==================
|
||||
|
||||
* add `--foo=bar` support [arv]
|
||||
* fix password on node 0.8.8. Make backward compatible with 0.6 [focusaurus]
|
||||
|
||||
1.0.1 / 2012-08-03
|
||||
==================
|
||||
|
||||
* fix issue #56
|
||||
* fix tty.setRawMode(mode) was moved to tty.ReadStream#setRawMode() (i.e. process.stdin.setRawMode())
|
||||
|
||||
1.0.0 / 2012-07-05
|
||||
==================
|
||||
|
||||
* add support for optional option descriptions
|
||||
* add defaulting of `.version()` to package.json's version
|
||||
|
||||
0.6.1 / 2012-06-01
|
||||
==================
|
||||
|
||||
* Added: append (yes or no) on confirmation
|
||||
* Added: allow node.js v0.7.x
|
||||
|
||||
0.6.0 / 2012-04-10
|
||||
==================
|
||||
|
||||
* Added `.prompt(obj, callback)` support. Closes #49
|
||||
* Added default support to .choose(). Closes #41
|
||||
* Fixed the choice example
|
||||
|
||||
0.5.1 / 2011-12-20
|
||||
==================
|
||||
|
||||
* Fixed `password()` for recent nodes. Closes #36
|
||||
|
||||
0.5.0 / 2011-12-04
|
||||
==================
|
||||
|
||||
* Added sub-command option support [itay]
|
||||
|
||||
0.4.3 / 2011-12-04
|
||||
==================
|
||||
|
||||
* Fixed custom help ordering. Closes #32
|
||||
|
||||
0.4.2 / 2011-11-24
|
||||
==================
|
||||
|
||||
* Added travis support
|
||||
* Fixed: line-buffered input automatically trimmed. Closes #31
|
||||
|
||||
0.4.1 / 2011-11-18
|
||||
==================
|
||||
|
||||
* Removed listening for "close" on --help
|
||||
|
||||
0.4.0 / 2011-11-15
|
||||
==================
|
||||
|
||||
* Added support for `--`. Closes #24
|
||||
|
||||
0.3.3 / 2011-11-14
|
||||
==================
|
||||
|
||||
* Fixed: wait for close event when writing help info [Jerry Hamlet]
|
||||
|
||||
0.3.2 / 2011-11-01
|
||||
==================
|
||||
|
||||
* Fixed long flag definitions with values [felixge]
|
||||
|
||||
0.3.1 / 2011-10-31
|
||||
==================
|
||||
|
||||
* Changed `--version` short flag to `-V` from `-v`
|
||||
* Changed `.version()` so it's configurable [felixge]
|
||||
|
||||
0.3.0 / 2011-10-31
|
||||
==================
|
||||
|
||||
* Added support for long flags only. Closes #18
|
||||
|
||||
0.2.1 / 2011-10-24
|
||||
==================
|
||||
|
||||
* "node": ">= 0.4.x < 0.7.0". Closes #20
|
||||
|
||||
0.2.0 / 2011-09-26
|
||||
==================
|
||||
|
||||
* Allow for defaults that are not just boolean. Default peassignment only occurs for --no-*, optional, and required arguments. [Jim Isaacs]
|
||||
|
||||
0.1.0 / 2011-08-24
|
||||
==================
|
||||
|
||||
* Added support for custom `--help` output
|
||||
|
||||
0.0.5 / 2011-08-18
|
||||
==================
|
||||
|
||||
* Changed: when the user enters nothing prompt for password again
|
||||
* Fixed issue with passwords beginning with numbers [NuckChorris]
|
||||
|
||||
0.0.4 / 2011-08-15
|
||||
==================
|
||||
|
||||
* Fixed `Commander#args`
|
||||
|
||||
0.0.3 / 2011-08-15
|
||||
==================
|
||||
|
||||
* Added default option value support
|
||||
|
||||
0.0.2 / 2011-08-15
|
||||
==================
|
||||
|
||||
* Added mask support to `Command#password(str[, mask], fn)`
|
||||
* Added `Command#password(str, fn)`
|
||||
|
||||
0.0.1 / 2010-01-03
|
||||
==================
|
||||
|
||||
* Initial release
|
|
@ -0,0 +1,22 @@
|
|||
(The MIT License)
|
||||
|
||||
Copyright (c) 2011 TJ Holowaychuk <tj@vision-media.ca>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
@ -0,0 +1,408 @@
|
|||
# Commander.js
|
||||
|
||||
|
||||
[](http://travis-ci.org/tj/commander.js)
|
||||
[](https://www.npmjs.org/package/commander)
|
||||
[](https://npmcharts.com/compare/commander?minimal=true)
|
||||
[](https://gitter.im/tj/commander.js?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
|
||||
|
||||
The complete solution for [node.js](http://nodejs.org) command-line interfaces, inspired by Ruby's [commander](https://github.com/commander-rb/commander).
|
||||
[API documentation](http://tj.github.com/commander.js/)
|
||||
|
||||
|
||||
## Installation
|
||||
|
||||
$ npm install commander --save
|
||||
|
||||
## Option parsing
|
||||
|
||||
Options with commander are defined with the `.option()` method, also serving as documentation for the options. The example below parses args and options from `process.argv`, leaving remaining args as the `program.args` array which were not consumed by options.
|
||||
|
||||
```js
|
||||
#!/usr/bin/env node
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var program = require('commander');
|
||||
|
||||
program
|
||||
.version('0.1.0')
|
||||
.option('-p, --peppers', 'Add peppers')
|
||||
.option('-P, --pineapple', 'Add pineapple')
|
||||
.option('-b, --bbq-sauce', 'Add bbq sauce')
|
||||
.option('-c, --cheese [type]', 'Add the specified type of cheese [marble]', 'marble')
|
||||
.parse(process.argv);
|
||||
|
||||
console.log('you ordered a pizza with:');
|
||||
if (program.peppers) console.log(' - peppers');
|
||||
if (program.pineapple) console.log(' - pineapple');
|
||||
if (program.bbqSauce) console.log(' - bbq');
|
||||
console.log(' - %s cheese', program.cheese);
|
||||
```
|
||||
|
||||
Short flags may be passed as a single arg, for example `-abc` is equivalent to `-a -b -c`. Multi-word options such as "--template-engine" are camel-cased, becoming `program.templateEngine` etc.
|
||||
|
||||
Note that multi-word options starting with `--no` prefix negate the boolean value of the following word. For example, `--no-sauce` sets the value of `program.sauce` to false.
|
||||
|
||||
```js
|
||||
#!/usr/bin/env node
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var program = require('commander');
|
||||
|
||||
program
|
||||
.option('--no-sauce', 'Remove sauce')
|
||||
.parse(process.argv);
|
||||
|
||||
console.log('you ordered a pizza');
|
||||
if (program.sauce) console.log(' with sauce');
|
||||
else console.log(' without sauce');
|
||||
```
|
||||
|
||||
## Version option
|
||||
|
||||
Calling the `version` implicitly adds the `-V` and `--version` options to the command.
|
||||
When either of these options is present, the command prints the version number and exits.
|
||||
|
||||
$ ./examples/pizza -V
|
||||
0.0.1
|
||||
|
||||
If you want your program to respond to the `-v` option instead of the `-V` option, simply pass custom flags to the `version` method using the same syntax as the `option` method.
|
||||
|
||||
```js
|
||||
program
|
||||
.version('0.0.1', '-v, --version')
|
||||
```
|
||||
|
||||
The version flags can be named anything, but the long option is required.
|
||||
|
||||
## Command-specific options
|
||||
|
||||
You can attach options to a command.
|
||||
|
||||
```js
|
||||
#!/usr/bin/env node
|
||||
|
||||
var program = require('commander');
|
||||
|
||||
program
|
||||
.command('rm <dir>')
|
||||
.option('-r, --recursive', 'Remove recursively')
|
||||
.action(function (dir, cmd) {
|
||||
console.log('remove ' + dir + (cmd.recursive ? ' recursively' : ''))
|
||||
})
|
||||
|
||||
program.parse(process.argv)
|
||||
```
|
||||
|
||||
A command's options are validated when the command is used. Any unknown options will be reported as an error. However, if an action-based command does not define an action, then the options are not validated.
|
||||
|
||||
## Coercion
|
||||
|
||||
```js
|
||||
function range(val) {
|
||||
return val.split('..').map(Number);
|
||||
}
|
||||
|
||||
function list(val) {
|
||||
return val.split(',');
|
||||
}
|
||||
|
||||
function collect(val, memo) {
|
||||
memo.push(val);
|
||||
return memo;
|
||||
}
|
||||
|
||||
function increaseVerbosity(v, total) {
|
||||
return total + 1;
|
||||
}
|
||||
|
||||
program
|
||||
.version('0.1.0')
|
||||
.usage('[options] <file ...>')
|
||||
.option('-i, --integer <n>', 'An integer argument', parseInt)
|
||||
.option('-f, --float <n>', 'A float argument', parseFloat)
|
||||
.option('-r, --range <a>..<b>', 'A range', range)
|
||||
.option('-l, --list <items>', 'A list', list)
|
||||
.option('-o, --optional [value]', 'An optional value')
|
||||
.option('-c, --collect [value]', 'A repeatable value', collect, [])
|
||||
.option('-v, --verbose', 'A value that can be increased', increaseVerbosity, 0)
|
||||
.parse(process.argv);
|
||||
|
||||
console.log(' int: %j', program.integer);
|
||||
console.log(' float: %j', program.float);
|
||||
console.log(' optional: %j', program.optional);
|
||||
program.range = program.range || [];
|
||||
console.log(' range: %j..%j', program.range[0], program.range[1]);
|
||||
console.log(' list: %j', program.list);
|
||||
console.log(' collect: %j', program.collect);
|
||||
console.log(' verbosity: %j', program.verbose);
|
||||
console.log(' args: %j', program.args);
|
||||
```
|
||||
|
||||
## Regular Expression
|
||||
```js
|
||||
program
|
||||
.version('0.1.0')
|
||||
.option('-s --size <size>', 'Pizza size', /^(large|medium|small)$/i, 'medium')
|
||||
.option('-d --drink [drink]', 'Drink', /^(coke|pepsi|izze)$/i)
|
||||
.parse(process.argv);
|
||||
|
||||
console.log(' size: %j', program.size);
|
||||
console.log(' drink: %j', program.drink);
|
||||
```
|
||||
|
||||
## Variadic arguments
|
||||
|
||||
The last argument of a command can be variadic, and only the last argument. To make an argument variadic you have to
|
||||
append `...` to the argument name. Here is an example:
|
||||
|
||||
```js
|
||||
#!/usr/bin/env node
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var program = require('commander');
|
||||
|
||||
program
|
||||
.version('0.1.0')
|
||||
.command('rmdir <dir> [otherDirs...]')
|
||||
.action(function (dir, otherDirs) {
|
||||
console.log('rmdir %s', dir);
|
||||
if (otherDirs) {
|
||||
otherDirs.forEach(function (oDir) {
|
||||
console.log('rmdir %s', oDir);
|
||||
});
|
||||
}
|
||||
});
|
||||
|
||||
program.parse(process.argv);
|
||||
```
|
||||
|
||||
An `Array` is used for the value of a variadic argument. This applies to `program.args` as well as the argument passed
|
||||
to your action as demonstrated above.
|
||||
|
||||
## Specify the argument syntax
|
||||
|
||||
```js
|
||||
#!/usr/bin/env node
|
||||
|
||||
var program = require('commander');
|
||||
|
||||
program
|
||||
.version('0.1.0')
|
||||
.arguments('<cmd> [env]')
|
||||
.action(function (cmd, env) {
|
||||
cmdValue = cmd;
|
||||
envValue = env;
|
||||
});
|
||||
|
||||
program.parse(process.argv);
|
||||
|
||||
if (typeof cmdValue === 'undefined') {
|
||||
console.error('no command given!');
|
||||
process.exit(1);
|
||||
}
|
||||
console.log('command:', cmdValue);
|
||||
console.log('environment:', envValue || "no environment given");
|
||||
```
|
||||
Angled brackets (e.g. `<cmd>`) indicate required input. Square brackets (e.g. `[env]`) indicate optional input.
|
||||
|
||||
## Git-style sub-commands
|
||||
|
||||
```js
|
||||
// file: ./examples/pm
|
||||
var program = require('commander');
|
||||
|
||||
program
|
||||
.version('0.1.0')
|
||||
.command('install [name]', 'install one or more packages')
|
||||
.command('search [query]', 'search with optional query')
|
||||
.command('list', 'list packages installed', {isDefault: true})
|
||||
.parse(process.argv);
|
||||
```
|
||||
|
||||
When `.command()` is invoked with a description argument, no `.action(callback)` should be called to handle sub-commands, otherwise there will be an error. This tells commander that you're going to use separate executables for sub-commands, much like `git(1)` and other popular tools.
|
||||
The commander will try to search the executables in the directory of the entry script (like `./examples/pm`) with the name `program-command`, like `pm-install`, `pm-search`.
|
||||
|
||||
Options can be passed with the call to `.command()`. Specifying `true` for `opts.noHelp` will remove the option from the generated help output. Specifying `true` for `opts.isDefault` will run the subcommand if no other subcommand is specified.
|
||||
|
||||
If the program is designed to be installed globally, make sure the executables have proper modes, like `755`.
|
||||
|
||||
### `--harmony`
|
||||
|
||||
You can enable `--harmony` option in two ways:
|
||||
* Use `#! /usr/bin/env node --harmony` in the sub-commands scripts. Note some os version don’t support this pattern.
|
||||
* Use the `--harmony` option when call the command, like `node --harmony examples/pm publish`. The `--harmony` option will be preserved when spawning sub-command process.
|
||||
|
||||
## Automated --help
|
||||
|
||||
The help information is auto-generated based on the information commander already knows about your program, so the following `--help` info is for free:
|
||||
|
||||
```
|
||||
$ ./examples/pizza --help
|
||||
|
||||
Usage: pizza [options]
|
||||
|
||||
An application for pizzas ordering
|
||||
|
||||
Options:
|
||||
|
||||
-h, --help output usage information
|
||||
-V, --version output the version number
|
||||
-p, --peppers Add peppers
|
||||
-P, --pineapple Add pineapple
|
||||
-b, --bbq Add bbq sauce
|
||||
-c, --cheese <type> Add the specified type of cheese [marble]
|
||||
-C, --no-cheese You do not want any cheese
|
||||
|
||||
```
|
||||
|
||||
## Custom help
|
||||
|
||||
You can display arbitrary `-h, --help` information
|
||||
by listening for "--help". Commander will automatically
|
||||
exit once you are done so that the remainder of your program
|
||||
does not execute causing undesired behaviours, for example
|
||||
in the following executable "stuff" will not output when
|
||||
`--help` is used.
|
||||
|
||||
```js
|
||||
#!/usr/bin/env node
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var program = require('commander');
|
||||
|
||||
program
|
||||
.version('0.1.0')
|
||||
.option('-f, --foo', 'enable some foo')
|
||||
.option('-b, --bar', 'enable some bar')
|
||||
.option('-B, --baz', 'enable some baz');
|
||||
|
||||
// must be before .parse() since
|
||||
// node's emit() is immediate
|
||||
|
||||
program.on('--help', function(){
|
||||
console.log(' Examples:');
|
||||
console.log('');
|
||||
console.log(' $ custom-help --help');
|
||||
console.log(' $ custom-help -h');
|
||||
console.log('');
|
||||
});
|
||||
|
||||
program.parse(process.argv);
|
||||
|
||||
console.log('stuff');
|
||||
```
|
||||
|
||||
Yields the following help output when `node script-name.js -h` or `node script-name.js --help` are run:
|
||||
|
||||
```
|
||||
|
||||
Usage: custom-help [options]
|
||||
|
||||
Options:
|
||||
|
||||
-h, --help output usage information
|
||||
-V, --version output the version number
|
||||
-f, --foo enable some foo
|
||||
-b, --bar enable some bar
|
||||
-B, --baz enable some baz
|
||||
|
||||
Examples:
|
||||
|
||||
$ custom-help --help
|
||||
$ custom-help -h
|
||||
|
||||
```
|
||||
|
||||
## .outputHelp(cb)
|
||||
|
||||
Output help information without exiting.
|
||||
Optional callback cb allows post-processing of help text before it is displayed.
|
||||
|
||||
If you want to display help by default (e.g. if no command was provided), you can use something like:
|
||||
|
||||
```js
|
||||
var program = require('commander');
|
||||
var colors = require('colors');
|
||||
|
||||
program
|
||||
.version('0.1.0')
|
||||
.command('getstream [url]', 'get stream URL')
|
||||
.parse(process.argv);
|
||||
|
||||
if (!process.argv.slice(2).length) {
|
||||
program.outputHelp(make_red);
|
||||
}
|
||||
|
||||
function make_red(txt) {
|
||||
return colors.red(txt); //display the help text in red on the console
|
||||
}
|
||||
```
|
||||
|
||||
## .help(cb)
|
||||
|
||||
Output help information and exit immediately.
|
||||
Optional callback cb allows post-processing of help text before it is displayed.
|
||||
|
||||
## Examples
|
||||
|
||||
```js
|
||||
var program = require('commander');
|
||||
|
||||
program
|
||||
.version('0.1.0')
|
||||
.option('-C, --chdir <path>', 'change the working directory')
|
||||
.option('-c, --config <path>', 'set config path. defaults to ./deploy.conf')
|
||||
.option('-T, --no-tests', 'ignore test hook');
|
||||
|
||||
program
|
||||
.command('setup [env]')
|
||||
.description('run setup commands for all envs')
|
||||
.option("-s, --setup_mode [mode]", "Which setup mode to use")
|
||||
.action(function(env, options){
|
||||
var mode = options.setup_mode || "normal";
|
||||
env = env || 'all';
|
||||
console.log('setup for %s env(s) with %s mode', env, mode);
|
||||
});
|
||||
|
||||
program
|
||||
.command('exec <cmd>')
|
||||
.alias('ex')
|
||||
.description('execute the given remote cmd')
|
||||
.option("-e, --exec_mode <mode>", "Which exec mode to use")
|
||||
.action(function(cmd, options){
|
||||
console.log('exec "%s" using %s mode', cmd, options.exec_mode);
|
||||
}).on('--help', function() {
|
||||
console.log(' Examples:');
|
||||
console.log();
|
||||
console.log(' $ deploy exec sequential');
|
||||
console.log(' $ deploy exec async');
|
||||
console.log();
|
||||
});
|
||||
|
||||
program
|
||||
.command('*')
|
||||
.action(function(env){
|
||||
console.log('deploying "%s"', env);
|
||||
});
|
||||
|
||||
program.parse(process.argv);
|
||||
```
|
||||
|
||||
More Demos can be found in the [examples](https://github.com/tj/commander.js/tree/master/examples) directory.
|
||||
|
||||
## License
|
||||
|
||||
MIT
|
File diff suppressed because it is too large
Load Diff
|
@ -0,0 +1,37 @@
|
|||
{
|
||||
"name": "commander",
|
||||
"version": "2.15.1",
|
||||
"description": "the complete solution for node.js command-line programs",
|
||||
"keywords": [
|
||||
"commander",
|
||||
"command",
|
||||
"option",
|
||||
"parser"
|
||||
],
|
||||
"author": "TJ Holowaychuk <tj@vision-media.ca>",
|
||||
"license": "MIT",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/tj/commander.js.git"
|
||||
},
|
||||
"scripts": {
|
||||
"lint": "eslint index.js",
|
||||
"test": "make test && npm run test-typings",
|
||||
"test-typings": "node_modules/typescript/bin/tsc -p tsconfig.json"
|
||||
},
|
||||
"main": "index",
|
||||
"files": [
|
||||
"index.js",
|
||||
"typings/index.d.ts"
|
||||
],
|
||||
"dependencies": {},
|
||||
"devDependencies": {
|
||||
"@types/node": "^7.0.55",
|
||||
"eslint": "^3.19.0",
|
||||
"should": "^11.2.1",
|
||||
"sinon": "^2.4.1",
|
||||
"standard": "^10.0.3",
|
||||
"typescript": "^2.7.2"
|
||||
},
|
||||
"typings": "typings/index.d.ts"
|
||||
}
|
|
@ -0,0 +1,309 @@
|
|||
// Type definitions for commander 2.11
|
||||
// Project: https://github.com/visionmedia/commander.js
|
||||
// Definitions by: Alan Agius <https://github.com/alan-agius4>, Marcelo Dezem <https://github.com/mdezem>, vvakame <https://github.com/vvakame>, Jules Randolph <https://github.com/sveinburne>
|
||||
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
|
||||
|
||||
declare namespace local {
|
||||
|
||||
class Option {
|
||||
flags: string;
|
||||
required: boolean;
|
||||
optional: boolean;
|
||||
bool: boolean;
|
||||
short?: string;
|
||||
long: string;
|
||||
description: string;
|
||||
|
||||
/**
|
||||
* Initialize a new `Option` with the given `flags` and `description`.
|
||||
*
|
||||
* @param {string} flags
|
||||
* @param {string} [description]
|
||||
*/
|
||||
constructor(flags: string, description?: string);
|
||||
}
|
||||
|
||||
class Command extends NodeJS.EventEmitter {
|
||||
[key: string]: any;
|
||||
|
||||
args: string[];
|
||||
|
||||
/**
|
||||
* Initialize a new `Command`.
|
||||
*
|
||||
* @param {string} [name]
|
||||
*/
|
||||
constructor(name?: string);
|
||||
|
||||
/**
|
||||
* Set the program version to `str`.
|
||||
*
|
||||
* This method auto-registers the "-V, --version" flag
|
||||
* which will print the version number when passed.
|
||||
*
|
||||
* @param {string} str
|
||||
* @param {string} [flags]
|
||||
* @returns {Command} for chaining
|
||||
*/
|
||||
version(str: string, flags?: string): Command;
|
||||
|
||||
/**
|
||||
* Add command `name`.
|
||||
*
|
||||
* The `.action()` callback is invoked when the
|
||||
* command `name` is specified via __ARGV__,
|
||||
* and the remaining arguments are applied to the
|
||||
* function for access.
|
||||
*
|
||||
* When the `name` is "*" an un-matched command
|
||||
* will be passed as the first arg, followed by
|
||||
* the rest of __ARGV__ remaining.
|
||||
*
|
||||
* @example
|
||||
* program
|
||||
* .version('0.0.1')
|
||||
* .option('-C, --chdir <path>', 'change the working directory')
|
||||
* .option('-c, --config <path>', 'set config path. defaults to ./deploy.conf')
|
||||
* .option('-T, --no-tests', 'ignore test hook')
|
||||
*
|
||||
* program
|
||||
* .command('setup')
|
||||
* .description('run remote setup commands')
|
||||
* .action(function() {
|
||||
* console.log('setup');
|
||||
* });
|
||||
*
|
||||
* program
|
||||
* .command('exec <cmd>')
|
||||
* .description('run the given remote command')
|
||||
* .action(function(cmd) {
|
||||
* console.log('exec "%s"', cmd);
|
||||
* });
|
||||
*
|
||||
* program
|
||||
* .command('teardown <dir> [otherDirs...]')
|
||||
* .description('run teardown commands')
|
||||
* .action(function(dir, otherDirs) {
|
||||
* console.log('dir "%s"', dir);
|
||||
* if (otherDirs) {
|
||||
* otherDirs.forEach(function (oDir) {
|
||||
* console.log('dir "%s"', oDir);
|
||||
* });
|
||||
* }
|
||||
* });
|
||||
*
|
||||
* program
|
||||
* .command('*')
|
||||
* .description('deploy the given env')
|
||||
* .action(function(env) {
|
||||
* console.log('deploying "%s"', env);
|
||||
* });
|
||||
*
|
||||
* program.parse(process.argv);
|
||||
*
|
||||
* @param {string} name
|
||||
* @param {string} [desc] for git-style sub-commands
|
||||
* @param {CommandOptions} [opts] command options
|
||||
* @returns {Command} the new command
|
||||
*/
|
||||
command(name: string, desc?: string, opts?: commander.CommandOptions): Command;
|
||||
|
||||
/**
|
||||
* Define argument syntax for the top-level command.
|
||||
*
|
||||
* @param {string} desc
|
||||
* @returns {Command} for chaining
|
||||
*/
|
||||
arguments(desc: string): Command;
|
||||
|
||||
/**
|
||||
* Parse expected `args`.
|
||||
*
|
||||
* For example `["[type]"]` becomes `[{ required: false, name: 'type' }]`.
|
||||
*
|
||||
* @param {string[]} args
|
||||
* @returns {Command} for chaining
|
||||
*/
|
||||
parseExpectedArgs(args: string[]): Command;
|
||||
|
||||
/**
|
||||
* Register callback `fn` for the command.
|
||||
*
|
||||
* @example
|
||||
* program
|
||||
* .command('help')
|
||||
* .description('display verbose help')
|
||||
* .action(function() {
|
||||
* // output help here
|
||||
* });
|
||||
*
|
||||
* @param {(...args: any[]) => void} fn
|
||||
* @returns {Command} for chaining
|
||||
*/
|
||||
action(fn: (...args: any[]) => void): Command;
|
||||
|
||||
/**
|
||||
* Define option with `flags`, `description` and optional
|
||||
* coercion `fn`.
|
||||
*
|
||||
* The `flags` string should contain both the short and long flags,
|
||||
* separated by comma, a pipe or space. The following are all valid
|
||||
* all will output this way when `--help` is used.
|
||||
*
|
||||
* "-p, --pepper"
|
||||
* "-p|--pepper"
|
||||
* "-p --pepper"
|
||||
*
|
||||
* @example
|
||||
* // simple boolean defaulting to false
|
||||
* program.option('-p, --pepper', 'add pepper');
|
||||
*
|
||||
* --pepper
|
||||
* program.pepper
|
||||
* // => Boolean
|
||||
*
|
||||
* // simple boolean defaulting to true
|
||||
* program.option('-C, --no-cheese', 'remove cheese');
|
||||
*
|
||||
* program.cheese
|
||||
* // => true
|
||||
*
|
||||
* --no-cheese
|
||||
* program.cheese
|
||||
* // => false
|
||||
*
|
||||
* // required argument
|
||||
* program.option('-C, --chdir <path>', 'change the working directory');
|
||||
*
|
||||
* --chdir /tmp
|
||||
* program.chdir
|
||||
* // => "/tmp"
|
||||
*
|
||||
* // optional argument
|
||||
* program.option('-c, --cheese [type]', 'add cheese [marble]');
|
||||
*
|
||||
* @param {string} flags
|
||||
* @param {string} [description]
|
||||
* @param {((arg1: any, arg2: any) => void) | RegExp} [fn] function or default
|
||||
* @param {*} [defaultValue]
|
||||
* @returns {Command} for chaining
|
||||
*/
|
||||
option(flags: string, description?: string, fn?: ((arg1: any, arg2: any) => void) | RegExp, defaultValue?: any): Command;
|
||||
option(flags: string, description?: string, defaultValue?: any): Command;
|
||||
|
||||
/**
|
||||
* Allow unknown options on the command line.
|
||||
*
|
||||
* @param {boolean} [arg] if `true` or omitted, no error will be thrown for unknown options.
|
||||
* @returns {Command} for chaining
|
||||
*/
|
||||
allowUnknownOption(arg?: boolean): Command;
|
||||
|
||||
/**
|
||||
* Parse `argv`, settings options and invoking commands when defined.
|
||||
*
|
||||
* @param {string[]} argv
|
||||
* @returns {Command} for chaining
|
||||
*/
|
||||
parse(argv: string[]): Command;
|
||||
|
||||
/**
|
||||
* Parse options from `argv` returning `argv` void of these options.
|
||||
*
|
||||
* @param {string[]} argv
|
||||
* @returns {ParseOptionsResult}
|
||||
*/
|
||||
parseOptions(argv: string[]): commander.ParseOptionsResult;
|
||||
|
||||
/**
|
||||
* Return an object containing options as key-value pairs
|
||||
*
|
||||
* @returns {{[key: string]: string}}
|
||||
*/
|
||||
opts(): { [key: string]: string };
|
||||
|
||||
/**
|
||||
* Set the description to `str`.
|
||||
*
|
||||
* @param {string} str
|
||||
* @return {(Command | string)}
|
||||
*/
|
||||
description(str: string): Command;
|
||||
description(): string;
|
||||
|
||||
/**
|
||||
* Set an alias for the command.
|
||||
*
|
||||
* @param {string} alias
|
||||
* @return {(Command | string)}
|
||||
*/
|
||||
alias(alias: string): Command;
|
||||
alias(): string;
|
||||
|
||||
/**
|
||||
* Set or get the command usage.
|
||||
*
|
||||
* @param {string} str
|
||||
* @return {(Command | string)}
|
||||
*/
|
||||
usage(str: string): Command;
|
||||
usage(): string;
|
||||
|
||||
/**
|
||||
* Set the name of the command.
|
||||
*
|
||||
* @param {string} str
|
||||
* @return {Command}
|
||||
*/
|
||||
name(str: string): Command;
|
||||
|
||||
/**
|
||||
* Get the name of the command.
|
||||
*
|
||||
* @return {string}
|
||||
*/
|
||||
name(): string;
|
||||
|
||||
/**
|
||||
* Output help information for this command.
|
||||
*
|
||||
* @param {(str: string) => string} [cb]
|
||||
*/
|
||||
outputHelp(cb?: (str: string) => string): void;
|
||||
|
||||
/** Output help information and exit.
|
||||
*
|
||||
* @param {(str: string) => string} [cb]
|
||||
*/
|
||||
help(cb?: (str: string) => string): void;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
declare namespace commander {
|
||||
|
||||
type Command = local.Command
|
||||
|
||||
type Option = local.Option
|
||||
|
||||
interface CommandOptions {
|
||||
noHelp?: boolean;
|
||||
isDefault?: boolean;
|
||||
}
|
||||
|
||||
interface ParseOptionsResult {
|
||||
args: string[];
|
||||
unknown: string[];
|
||||
}
|
||||
|
||||
interface CommanderStatic extends Command {
|
||||
Command: typeof local.Command;
|
||||
Option: typeof local.Option;
|
||||
CommandOptions: CommandOptions;
|
||||
ParseOptionsResult: ParseOptionsResult;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
declare const commander: commander.CommanderStatic;
|
||||
export = commander;
|
|
@ -0,0 +1,4 @@
|
|||
language: node_js
|
||||
node_js:
|
||||
- 0.4
|
||||
- 0.6
|
|
@ -0,0 +1,18 @@
|
|||
This software is released under the MIT license:
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
this software and associated documentation files (the "Software"), to deal in
|
||||
the Software without restriction, including without limitation the rights to
|
||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
|
||||
the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
|
||||
FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
|
||||
COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
|
||||
IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
||||
CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
@ -0,0 +1,62 @@
|
|||
concat-map
|
||||
==========
|
||||
|
||||
Concatenative mapdashery.
|
||||
|
||||
[](http://ci.testling.com/substack/node-concat-map)
|
||||
|
||||
[](http://travis-ci.org/substack/node-concat-map)
|
||||
|
||||
example
|
||||
=======
|
||||
|
||||
``` js
|
||||
var concatMap = require('concat-map');
|
||||
var xs = [ 1, 2, 3, 4, 5, 6 ];
|
||||
var ys = concatMap(xs, function (x) {
|
||||
return x % 2 ? [ x - 0.1, x, x + 0.1 ] : [];
|
||||
});
|
||||
console.dir(ys);
|
||||
```
|
||||
|
||||
***
|
||||
|
||||
```
|
||||
[ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ]
|
||||
```
|
||||
|
||||
methods
|
||||
=======
|
||||
|
||||
``` js
|
||||
var concatMap = require('concat-map')
|
||||
```
|
||||
|
||||
concatMap(xs, fn)
|
||||
-----------------
|
||||
|
||||
Return an array of concatenated elements by calling `fn(x, i)` for each element
|
||||
`x` and each index `i` in the array `xs`.
|
||||
|
||||
When `fn(x, i)` returns an array, its result will be concatenated with the
|
||||
result array. If `fn(x, i)` returns anything else, that value will be pushed
|
||||
onto the end of the result array.
|
||||
|
||||
install
|
||||
=======
|
||||
|
||||
With [npm](http://npmjs.org) do:
|
||||
|
||||
```
|
||||
npm install concat-map
|
||||
```
|
||||
|
||||
license
|
||||
=======
|
||||
|
||||
MIT
|
||||
|
||||
notes
|
||||
=====
|
||||
|
||||
This module was written while sitting high above the ground in a tree.
|
|
@ -0,0 +1,6 @@
|
|||
var concatMap = require('../');
|
||||
var xs = [ 1, 2, 3, 4, 5, 6 ];
|
||||
var ys = concatMap(xs, function (x) {
|
||||
return x % 2 ? [ x - 0.1, x, x + 0.1 ] : [];
|
||||
});
|
||||
console.dir(ys);
|
|
@ -0,0 +1,13 @@
|
|||
module.exports = function (xs, fn) {
|
||||
var res = [];
|
||||
for (var i = 0; i < xs.length; i++) {
|
||||
var x = fn(xs[i], i);
|
||||
if (isArray(x)) res.push.apply(res, x);
|
||||
else res.push(x);
|
||||
}
|
||||
return res;
|
||||
};
|
||||
|
||||
var isArray = Array.isArray || function (xs) {
|
||||
return Object.prototype.toString.call(xs) === '[object Array]';
|
||||
};
|
|
@ -0,0 +1,43 @@
|
|||
{
|
||||
"name" : "concat-map",
|
||||
"description" : "concatenative mapdashery",
|
||||
"version" : "0.0.1",
|
||||
"repository" : {
|
||||
"type" : "git",
|
||||
"url" : "git://github.com/substack/node-concat-map.git"
|
||||
},
|
||||
"main" : "index.js",
|
||||
"keywords" : [
|
||||
"concat",
|
||||
"concatMap",
|
||||
"map",
|
||||
"functional",
|
||||
"higher-order"
|
||||
],
|
||||
"directories" : {
|
||||
"example" : "example",
|
||||
"test" : "test"
|
||||
},
|
||||
"scripts" : {
|
||||
"test" : "tape test/*.js"
|
||||
},
|
||||
"devDependencies" : {
|
||||
"tape" : "~2.4.0"
|
||||
},
|
||||
"license" : "MIT",
|
||||
"author" : {
|
||||
"name" : "James Halliday",
|
||||
"email" : "mail@substack.net",
|
||||
"url" : "http://substack.net"
|
||||
},
|
||||
"testling" : {
|
||||
"files" : "test/*.js",
|
||||
"browsers" : {
|
||||
"ie" : [ 6, 7, 8, 9 ],
|
||||
"ff" : [ 3.5, 10, 15.0 ],
|
||||
"chrome" : [ 10, 22 ],
|
||||
"safari" : [ 5.1 ],
|
||||
"opera" : [ 12 ]
|
||||
}
|
||||
}
|
||||
}
|
|
@ -0,0 +1,39 @@
|
|||
var concatMap = require('../');
|
||||
var test = require('tape');
|
||||
|
||||
test('empty or not', function (t) {
|
||||
var xs = [ 1, 2, 3, 4, 5, 6 ];
|
||||
var ixes = [];
|
||||
var ys = concatMap(xs, function (x, ix) {
|
||||
ixes.push(ix);
|
||||
return x % 2 ? [ x - 0.1, x, x + 0.1 ] : [];
|
||||
});
|
||||
t.same(ys, [ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ]);
|
||||
t.same(ixes, [ 0, 1, 2, 3, 4, 5 ]);
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('always something', function (t) {
|
||||
var xs = [ 'a', 'b', 'c', 'd' ];
|
||||
var ys = concatMap(xs, function (x) {
|
||||
return x === 'b' ? [ 'B', 'B', 'B' ] : [ x ];
|
||||
});
|
||||
t.same(ys, [ 'a', 'B', 'B', 'B', 'c', 'd' ]);
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('scalars', function (t) {
|
||||
var xs = [ 'a', 'b', 'c', 'd' ];
|
||||
var ys = concatMap(xs, function (x) {
|
||||
return x === 'b' ? [ 'B', 'B', 'B' ] : x;
|
||||
});
|
||||
t.same(ys, [ 'a', 'B', 'B', 'B', 'c', 'd' ]);
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('undefs', function (t) {
|
||||
var xs = [ 'a', 'b', 'c', 'd' ];
|
||||
var ys = concatMap(xs, function () {});
|
||||
t.same(ys, [ undefined, undefined, undefined, undefined ]);
|
||||
t.end();
|
||||
});
|
|
@ -0,0 +1,20 @@
|
|||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014-2017 TJ Holowaychuk <tj@vision-media.ca>
|
||||
Copyright (c) 2018-2021 Josh Junon
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of this software
|
||||
and associated documentation files (the 'Software'), to deal in the Software without restriction,
|
||||
including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense,
|
||||
and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all copies or substantial
|
||||
portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT
|
||||
LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
|
||||
WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
|
@ -0,0 +1,481 @@
|
|||
# debug
|
||||
[](https://travis-ci.org/debug-js/debug) [](https://coveralls.io/github/debug-js/debug?branch=master) [](https://visionmedia-community-slackin.now.sh/) [](#backers)
|
||||
[](#sponsors)
|
||||
|
||||
<img width="647" src="https://user-images.githubusercontent.com/71256/29091486-fa38524c-7c37-11e7-895f-e7ec8e1039b6.png">
|
||||
|
||||
A tiny JavaScript debugging utility modelled after Node.js core's debugging
|
||||
technique. Works in Node.js and web browsers.
|
||||
|
||||
## Installation
|
||||
|
||||
```bash
|
||||
$ npm install debug
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
`debug` exposes a function; simply pass this function the name of your module, and it will return a decorated version of `console.error` for you to pass debug statements to. This will allow you to toggle the debug output for different parts of your module as well as the module as a whole.
|
||||
|
||||
Example [_app.js_](./examples/node/app.js):
|
||||
|
||||
```js
|
||||
var debug = require('debug')('http')
|
||||
, http = require('http')
|
||||
, name = 'My App';
|
||||
|
||||
// fake app
|
||||
|
||||
debug('booting %o', name);
|
||||
|
||||
http.createServer(function(req, res){
|
||||
debug(req.method + ' ' + req.url);
|
||||
res.end('hello\n');
|
||||
}).listen(3000, function(){
|
||||
debug('listening');
|
||||
});
|
||||
|
||||
// fake worker of some kind
|
||||
|
||||
require('./worker');
|
||||
```
|
||||
|
||||
Example [_worker.js_](./examples/node/worker.js):
|
||||
|
||||
```js
|
||||
var a = require('debug')('worker:a')
|
||||
, b = require('debug')('worker:b');
|
||||
|
||||
function work() {
|
||||
a('doing lots of uninteresting work');
|
||||
setTimeout(work, Math.random() * 1000);
|
||||
}
|
||||
|
||||
work();
|
||||
|
||||
function workb() {
|
||||
b('doing some work');
|
||||
setTimeout(workb, Math.random() * 2000);
|
||||
}
|
||||
|
||||
workb();
|
||||
```
|
||||
|
||||
The `DEBUG` environment variable is then used to enable these based on space or
|
||||
comma-delimited names.
|
||||
|
||||
Here are some examples:
|
||||
|
||||
<img width="647" alt="screen shot 2017-08-08 at 12 53 04 pm" src="https://user-images.githubusercontent.com/71256/29091703-a6302cdc-7c38-11e7-8304-7c0b3bc600cd.png">
|
||||
<img width="647" alt="screen shot 2017-08-08 at 12 53 38 pm" src="https://user-images.githubusercontent.com/71256/29091700-a62a6888-7c38-11e7-800b-db911291ca2b.png">
|
||||
<img width="647" alt="screen shot 2017-08-08 at 12 53 25 pm" src="https://user-images.githubusercontent.com/71256/29091701-a62ea114-7c38-11e7-826a-2692bedca740.png">
|
||||
|
||||
#### Windows command prompt notes
|
||||
|
||||
##### CMD
|
||||
|
||||
On Windows the environment variable is set using the `set` command.
|
||||
|
||||
```cmd
|
||||
set DEBUG=*,-not_this
|
||||
```
|
||||
|
||||
Example:
|
||||
|
||||
```cmd
|
||||
set DEBUG=* & node app.js
|
||||
```
|
||||
|
||||
##### PowerShell (VS Code default)
|
||||
|
||||
PowerShell uses different syntax to set environment variables.
|
||||
|
||||
```cmd
|
||||
$env:DEBUG = "*,-not_this"
|
||||
```
|
||||
|
||||
Example:
|
||||
|
||||
```cmd
|
||||
$env:DEBUG='app';node app.js
|
||||
```
|
||||
|
||||
Then, run the program to be debugged as usual.
|
||||
|
||||
npm script example:
|
||||
```js
|
||||
"windowsDebug": "@powershell -Command $env:DEBUG='*';node app.js",
|
||||
```
|
||||
|
||||
## Namespace Colors
|
||||
|
||||
Every debug instance has a color generated for it based on its namespace name.
|
||||
This helps when visually parsing the debug output to identify which debug instance
|
||||
a debug line belongs to.
|
||||
|
||||
#### Node.js
|
||||
|
||||
In Node.js, colors are enabled when stderr is a TTY. You also _should_ install
|
||||
the [`supports-color`](https://npmjs.org/supports-color) module alongside debug,
|
||||
otherwise debug will only use a small handful of basic colors.
|
||||
|
||||
<img width="521" src="https://user-images.githubusercontent.com/71256/29092181-47f6a9e6-7c3a-11e7-9a14-1928d8a711cd.png">
|
||||
|
||||
#### Web Browser
|
||||
|
||||
Colors are also enabled on "Web Inspectors" that understand the `%c` formatting
|
||||
option. These are WebKit web inspectors, Firefox ([since version
|
||||
31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/))
|
||||
and the Firebug plugin for Firefox (any version).
|
||||
|
||||
<img width="524" src="https://user-images.githubusercontent.com/71256/29092033-b65f9f2e-7c39-11e7-8e32-f6f0d8e865c1.png">
|
||||
|
||||
|
||||
## Millisecond diff
|
||||
|
||||
When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.
|
||||
|
||||
<img width="647" src="https://user-images.githubusercontent.com/71256/29091486-fa38524c-7c37-11e7-895f-e7ec8e1039b6.png">
|
||||
|
||||
When stdout is not a TTY, `Date#toISOString()` is used, making it more useful for logging the debug information as shown below:
|
||||
|
||||
<img width="647" src="https://user-images.githubusercontent.com/71256/29091956-6bd78372-7c39-11e7-8c55-c948396d6edd.png">
|
||||
|
||||
|
||||
## Conventions
|
||||
|
||||
If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser". If you append a "*" to the end of your name, it will always be enabled regardless of the setting of the DEBUG environment variable. You can then use it for normal output as well as debug output.
|
||||
|
||||
## Wildcards
|
||||
|
||||
The `*` character may be used as a wildcard. Suppose for example your library has
|
||||
debuggers named "connect:bodyParser", "connect:compress", "connect:session",
|
||||
instead of listing all three with
|
||||
`DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do
|
||||
`DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`.
|
||||
|
||||
You can also exclude specific debuggers by prefixing them with a "-" character.
|
||||
For example, `DEBUG=*,-connect:*` would include all debuggers except those
|
||||
starting with "connect:".
|
||||
|
||||
## Environment Variables
|
||||
|
||||
When running through Node.js, you can set a few environment variables that will
|
||||
change the behavior of the debug logging:
|
||||
|
||||
| Name | Purpose |
|
||||
|-----------|-------------------------------------------------|
|
||||
| `DEBUG` | Enables/disables specific debugging namespaces. |
|
||||
| `DEBUG_HIDE_DATE` | Hide date from debug output (non-TTY). |
|
||||
| `DEBUG_COLORS`| Whether or not to use colors in the debug output. |
|
||||
| `DEBUG_DEPTH` | Object inspection depth. |
|
||||
| `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. |
|
||||
|
||||
|
||||
__Note:__ The environment variables beginning with `DEBUG_` end up being
|
||||
converted into an Options object that gets used with `%o`/`%O` formatters.
|
||||
See the Node.js documentation for
|
||||
[`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options)
|
||||
for the complete list.
|
||||
|
||||
## Formatters
|
||||
|
||||
Debug uses [printf-style](https://wikipedia.org/wiki/Printf_format_string) formatting.
|
||||
Below are the officially supported formatters:
|
||||
|
||||
| Formatter | Representation |
|
||||
|-----------|----------------|
|
||||
| `%O` | Pretty-print an Object on multiple lines. |
|
||||
| `%o` | Pretty-print an Object all on a single line. |
|
||||
| `%s` | String. |
|
||||
| `%d` | Number (both integer and float). |
|
||||
| `%j` | JSON. Replaced with the string '[Circular]' if the argument contains circular references. |
|
||||
| `%%` | Single percent sign ('%'). This does not consume an argument. |
|
||||
|
||||
|
||||
### Custom formatters
|
||||
|
||||
You can add custom formatters by extending the `debug.formatters` object.
|
||||
For example, if you wanted to add support for rendering a Buffer as hex with
|
||||
`%h`, you could do something like:
|
||||
|
||||
```js
|
||||
const createDebug = require('debug')
|
||||
createDebug.formatters.h = (v) => {
|
||||
return v.toString('hex')
|
||||
}
|
||||
|
||||
// …elsewhere
|
||||
const debug = createDebug('foo')
|
||||
debug('this is hex: %h', new Buffer('hello world'))
|
||||
// foo this is hex: 68656c6c6f20776f726c6421 +0ms
|
||||
```
|
||||
|
||||
|
||||
## Browser Support
|
||||
|
||||
You can build a browser-ready script using [browserify](https://github.com/substack/node-browserify),
|
||||
or just use the [browserify-as-a-service](https://wzrd.in/) [build](https://wzrd.in/standalone/debug@latest),
|
||||
if you don't want to build it yourself.
|
||||
|
||||
Debug's enable state is currently persisted by `localStorage`.
|
||||
Consider the situation shown below where you have `worker:a` and `worker:b`,
|
||||
and wish to debug both. You can enable this using `localStorage.debug`:
|
||||
|
||||
```js
|
||||
localStorage.debug = 'worker:*'
|
||||
```
|
||||
|
||||
And then refresh the page.
|
||||
|
||||
```js
|
||||
a = debug('worker:a');
|
||||
b = debug('worker:b');
|
||||
|
||||
setInterval(function(){
|
||||
a('doing some work');
|
||||
}, 1000);
|
||||
|
||||
setInterval(function(){
|
||||
b('doing some work');
|
||||
}, 1200);
|
||||
```
|
||||
|
||||
In Chromium-based web browsers (e.g. Brave, Chrome, and Electron), the JavaScript console will—by default—only show messages logged by `debug` if the "Verbose" log level is _enabled_.
|
||||
|
||||
<img width="647" src="https://user-images.githubusercontent.com/7143133/152083257-29034707-c42c-4959-8add-3cee850e6fcf.png">
|
||||
|
||||
## Output streams
|
||||
|
||||
By default `debug` will log to stderr, however this can be configured per-namespace by overriding the `log` method:
|
||||
|
||||
Example [_stdout.js_](./examples/node/stdout.js):
|
||||
|
||||
```js
|
||||
var debug = require('debug');
|
||||
var error = debug('app:error');
|
||||
|
||||
// by default stderr is used
|
||||
error('goes to stderr!');
|
||||
|
||||
var log = debug('app:log');
|
||||
// set this namespace to log via console.log
|
||||
log.log = console.log.bind(console); // don't forget to bind to console!
|
||||
log('goes to stdout');
|
||||
error('still goes to stderr!');
|
||||
|
||||
// set all output to go via console.info
|
||||
// overrides all per-namespace log settings
|
||||
debug.log = console.info.bind(console);
|
||||
error('now goes to stdout via console.info');
|
||||
log('still goes to stdout, but via console.info now');
|
||||
```
|
||||
|
||||
## Extend
|
||||
You can simply extend debugger
|
||||
```js
|
||||
const log = require('debug')('auth');
|
||||
|
||||
//creates new debug instance with extended namespace
|
||||
const logSign = log.extend('sign');
|
||||
const logLogin = log.extend('login');
|
||||
|
||||
log('hello'); // auth hello
|
||||
logSign('hello'); //auth:sign hello
|
||||
logLogin('hello'); //auth:login hello
|
||||
```
|
||||
|
||||
## Set dynamically
|
||||
|
||||
You can also enable debug dynamically by calling the `enable()` method :
|
||||
|
||||
```js
|
||||
let debug = require('debug');
|
||||
|
||||
console.log(1, debug.enabled('test'));
|
||||
|
||||
debug.enable('test');
|
||||
console.log(2, debug.enabled('test'));
|
||||
|
||||
debug.disable();
|
||||
console.log(3, debug.enabled('test'));
|
||||
|
||||
```
|
||||
|
||||
print :
|
||||
```
|
||||
1 false
|
||||
2 true
|
||||
3 false
|
||||
```
|
||||
|
||||
Usage :
|
||||
`enable(namespaces)`
|
||||
`namespaces` can include modes separated by a colon and wildcards.
|
||||
|
||||
Note that calling `enable()` completely overrides previously set DEBUG variable :
|
||||
|
||||
```
|
||||
$ DEBUG=foo node -e 'var dbg = require("debug"); dbg.enable("bar"); console.log(dbg.enabled("foo"))'
|
||||
=> false
|
||||
```
|
||||
|
||||
`disable()`
|
||||
|
||||
Will disable all namespaces. The functions returns the namespaces currently
|
||||
enabled (and skipped). This can be useful if you want to disable debugging
|
||||
temporarily without knowing what was enabled to begin with.
|
||||
|
||||
For example:
|
||||
|
||||
```js
|
||||
let debug = require('debug');
|
||||
debug.enable('foo:*,-foo:bar');
|
||||
let namespaces = debug.disable();
|
||||
debug.enable(namespaces);
|
||||
```
|
||||
|
||||
Note: There is no guarantee that the string will be identical to the initial
|
||||
enable string, but semantically they will be identical.
|
||||
|
||||
## Checking whether a debug target is enabled
|
||||
|
||||
After you've created a debug instance, you can determine whether or not it is
|
||||
enabled by checking the `enabled` property:
|
||||
|
||||
```javascript
|
||||
const debug = require('debug')('http');
|
||||
|
||||
if (debug.enabled) {
|
||||
// do stuff...
|
||||
}
|
||||
```
|
||||
|
||||
You can also manually toggle this property to force the debug instance to be
|
||||
enabled or disabled.
|
||||
|
||||
## Usage in child processes
|
||||
|
||||
Due to the way `debug` detects if the output is a TTY or not, colors are not shown in child processes when `stderr` is piped. A solution is to pass the `DEBUG_COLORS=1` environment variable to the child process.
|
||||
For example:
|
||||
|
||||
```javascript
|
||||
worker = fork(WORKER_WRAP_PATH, [workerPath], {
|
||||
stdio: [
|
||||
/* stdin: */ 0,
|
||||
/* stdout: */ 'pipe',
|
||||
/* stderr: */ 'pipe',
|
||||
'ipc',
|
||||
],
|
||||
env: Object.assign({}, process.env, {
|
||||
DEBUG_COLORS: 1 // without this settings, colors won't be shown
|
||||
}),
|
||||
});
|
||||
|
||||
worker.stderr.pipe(process.stderr, { end: false });
|
||||
```
|
||||
|
||||
|
||||
## Authors
|
||||
|
||||
- TJ Holowaychuk
|
||||
- Nathan Rajlich
|
||||
- Andrew Rhyne
|
||||
- Josh Junon
|
||||
|
||||
## Backers
|
||||
|
||||
Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/debug#backer)]
|
||||
|
||||
<a href="https://opencollective.com/debug/backer/0/website" target="_blank"><img src="https://opencollective.com/debug/backer/0/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/1/website" target="_blank"><img src="https://opencollective.com/debug/backer/1/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/2/website" target="_blank"><img src="https://opencollective.com/debug/backer/2/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/3/website" target="_blank"><img src="https://opencollective.com/debug/backer/3/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/4/website" target="_blank"><img src="https://opencollective.com/debug/backer/4/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/5/website" target="_blank"><img src="https://opencollective.com/debug/backer/5/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/6/website" target="_blank"><img src="https://opencollective.com/debug/backer/6/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/7/website" target="_blank"><img src="https://opencollective.com/debug/backer/7/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/8/website" target="_blank"><img src="https://opencollective.com/debug/backer/8/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/9/website" target="_blank"><img src="https://opencollective.com/debug/backer/9/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/10/website" target="_blank"><img src="https://opencollective.com/debug/backer/10/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/11/website" target="_blank"><img src="https://opencollective.com/debug/backer/11/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/12/website" target="_blank"><img src="https://opencollective.com/debug/backer/12/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/13/website" target="_blank"><img src="https://opencollective.com/debug/backer/13/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/14/website" target="_blank"><img src="https://opencollective.com/debug/backer/14/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/15/website" target="_blank"><img src="https://opencollective.com/debug/backer/15/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/16/website" target="_blank"><img src="https://opencollective.com/debug/backer/16/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/17/website" target="_blank"><img src="https://opencollective.com/debug/backer/17/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/18/website" target="_blank"><img src="https://opencollective.com/debug/backer/18/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/19/website" target="_blank"><img src="https://opencollective.com/debug/backer/19/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/20/website" target="_blank"><img src="https://opencollective.com/debug/backer/20/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/21/website" target="_blank"><img src="https://opencollective.com/debug/backer/21/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/22/website" target="_blank"><img src="https://opencollective.com/debug/backer/22/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/23/website" target="_blank"><img src="https://opencollective.com/debug/backer/23/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/24/website" target="_blank"><img src="https://opencollective.com/debug/backer/24/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/25/website" target="_blank"><img src="https://opencollective.com/debug/backer/25/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/26/website" target="_blank"><img src="https://opencollective.com/debug/backer/26/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/27/website" target="_blank"><img src="https://opencollective.com/debug/backer/27/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/28/website" target="_blank"><img src="https://opencollective.com/debug/backer/28/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/backer/29/website" target="_blank"><img src="https://opencollective.com/debug/backer/29/avatar.svg"></a>
|
||||
|
||||
|
||||
## Sponsors
|
||||
|
||||
Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/debug#sponsor)]
|
||||
|
||||
<a href="https://opencollective.com/debug/sponsor/0/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/0/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/1/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/1/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/2/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/2/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/3/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/3/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/4/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/4/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/5/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/5/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/6/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/6/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/7/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/7/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/8/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/8/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/9/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/9/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/10/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/10/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/11/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/11/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/12/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/12/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/13/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/13/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/14/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/14/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/15/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/15/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/16/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/16/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/17/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/17/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/18/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/18/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/19/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/19/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/20/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/20/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/21/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/21/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/22/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/22/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/23/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/23/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/24/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/24/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/25/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/25/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/26/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/26/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/27/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/27/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/28/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/28/avatar.svg"></a>
|
||||
<a href="https://opencollective.com/debug/sponsor/29/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/29/avatar.svg"></a>
|
||||
|
||||
## License
|
||||
|
||||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014-2017 TJ Holowaychuk <tj@vision-media.ca>
|
||||
Copyright (c) 2018-2021 Josh Junon
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
'Software'), to deal in the Software without restriction, including
|
||||
without limitation the rights to use, copy, modify, merge, publish,
|
||||
distribute, sublicense, and/or sell copies of the Software, and to
|
||||
permit persons to whom the Software is furnished to do so, subject to
|
||||
the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
||||
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
|
||||
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
|
||||
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
|
||||
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
@ -0,0 +1,59 @@
|
|||
{
|
||||
"name": "debug",
|
||||
"version": "4.3.4",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/debug-js/debug.git"
|
||||
},
|
||||
"description": "Lightweight debugging utility for Node.js and the browser",
|
||||
"keywords": [
|
||||
"debug",
|
||||
"log",
|
||||
"debugger"
|
||||
],
|
||||
"files": [
|
||||
"src",
|
||||
"LICENSE",
|
||||
"README.md"
|
||||
],
|
||||
"author": "Josh Junon <josh.junon@protonmail.com>",
|
||||
"contributors": [
|
||||
"TJ Holowaychuk <tj@vision-media.ca>",
|
||||
"Nathan Rajlich <nathan@tootallnate.net> (http://n8.io)",
|
||||
"Andrew Rhyne <rhyneandrew@gmail.com>"
|
||||
],
|
||||
"license": "MIT",
|
||||
"scripts": {
|
||||
"lint": "xo",
|
||||
"test": "npm run test:node && npm run test:browser && npm run lint",
|
||||
"test:node": "istanbul cover _mocha -- test.js",
|
||||
"test:browser": "karma start --single-run",
|
||||
"test:coverage": "cat ./coverage/lcov.info | coveralls"
|
||||
},
|
||||
"dependencies": {
|
||||
"ms": "2.1.2"
|
||||
},
|
||||
"devDependencies": {
|
||||
"brfs": "^2.0.1",
|
||||
"browserify": "^16.2.3",
|
||||
"coveralls": "^3.0.2",
|
||||
"istanbul": "^0.4.5",
|
||||
"karma": "^3.1.4",
|
||||
"karma-browserify": "^6.0.0",
|
||||
"karma-chrome-launcher": "^2.2.0",
|
||||
"karma-mocha": "^1.3.0",
|
||||
"mocha": "^5.2.0",
|
||||
"mocha-lcov-reporter": "^1.2.0",
|
||||
"xo": "^0.23.0"
|
||||
},
|
||||
"peerDependenciesMeta": {
|
||||
"supports-color": {
|
||||
"optional": true
|
||||
}
|
||||
},
|
||||
"main": "./src/index.js",
|
||||
"browser": "./src/browser.js",
|
||||
"engines": {
|
||||
"node": ">=6.0"
|
||||
}
|
||||
}
|
|
@ -0,0 +1,269 @@
|
|||
/* eslint-env browser */
|
||||
|
||||
/**
|
||||
* This is the web browser implementation of `debug()`.
|
||||
*/
|
||||
|
||||
exports.formatArgs = formatArgs;
|
||||
exports.save = save;
|
||||
exports.load = load;
|
||||
exports.useColors = useColors;
|
||||
exports.storage = localstorage();
|
||||
exports.destroy = (() => {
|
||||
let warned = false;
|
||||
|
||||
return () => {
|
||||
if (!warned) {
|
||||
warned = true;
|
||||
console.warn('Instance method `debug.destroy()` is deprecated and no longer does anything. It will be removed in the next major version of `debug`.');
|
||||
}
|
||||
};
|
||||
})();
|
||||
|
||||
/**
|
||||
* Colors.
|
||||
*/
|
||||
|
||||
exports.colors = [
|
||||
'#0000CC',
|
||||
'#0000FF',
|
||||
'#0033CC',
|
||||
'#0033FF',
|
||||
'#0066CC',
|
||||
'#0066FF',
|
||||
'#0099CC',
|
||||
'#0099FF',
|
||||
'#00CC00',
|
||||
'#00CC33',
|
||||
'#00CC66',
|
||||
'#00CC99',
|
||||
'#00CCCC',
|
||||
'#00CCFF',
|
||||
'#3300CC',
|
||||
'#3300FF',
|
||||
'#3333CC',
|
||||
'#3333FF',
|
||||
'#3366CC',
|
||||
'#3366FF',
|
||||
'#3399CC',
|
||||
'#3399FF',
|
||||
'#33CC00',
|
||||
'#33CC33',
|
||||
'#33CC66',
|
||||
'#33CC99',
|
||||
'#33CCCC',
|
||||
'#33CCFF',
|
||||
'#6600CC',
|
||||
'#6600FF',
|
||||
'#6633CC',
|
||||
'#6633FF',
|
||||
'#66CC00',
|
||||
'#66CC33',
|
||||
'#9900CC',
|
||||
'#9900FF',
|
||||
'#9933CC',
|
||||
'#9933FF',
|
||||
'#99CC00',
|
||||
'#99CC33',
|
||||
'#CC0000',
|
||||
'#CC0033',
|
||||
'#CC0066',
|
||||
'#CC0099',
|
||||
'#CC00CC',
|
||||
'#CC00FF',
|
||||
'#CC3300',
|
||||
'#CC3333',
|
||||
'#CC3366',
|
||||
'#CC3399',
|
||||
'#CC33CC',
|
||||
'#CC33FF',
|
||||
'#CC6600',
|
||||
'#CC6633',
|
||||
'#CC9900',
|
||||
'#CC9933',
|
||||
'#CCCC00',
|
||||
'#CCCC33',
|
||||
'#FF0000',
|
||||
'#FF0033',
|
||||
'#FF0066',
|
||||
'#FF0099',
|
||||
'#FF00CC',
|
||||
'#FF00FF',
|
||||
'#FF3300',
|
||||
'#FF3333',
|
||||
'#FF3366',
|
||||
'#FF3399',
|
||||
'#FF33CC',
|
||||
'#FF33FF',
|
||||
'#FF6600',
|
||||
'#FF6633',
|
||||
'#FF9900',
|
||||
'#FF9933',
|
||||
'#FFCC00',
|
||||
'#FFCC33'
|
||||
];
|
||||
|
||||
/**
|
||||
* Currently only WebKit-based Web Inspectors, Firefox >= v31,
|
||||
* and the Firebug extension (any Firefox version) are known
|
||||
* to support "%c" CSS customizations.
|
||||
*
|
||||
* TODO: add a `localStorage` variable to explicitly enable/disable colors
|
||||
*/
|
||||
|
||||
// eslint-disable-next-line complexity
|
||||
function useColors() {
|
||||
// NB: In an Electron preload script, document will be defined but not fully
|
||||
// initialized. Since we know we're in Chrome, we'll just detect this case
|
||||
// explicitly
|
||||
if (typeof window !== 'undefined' && window.process && (window.process.type === 'renderer' || window.process.__nwjs)) {
|
||||
return true;
|
||||
}
|
||||
|
||||
// Internet Explorer and Edge do not support colors.
|
||||
if (typeof navigator !== 'undefined' && navigator.userAgent && navigator.userAgent.toLowerCase().match(/(edge|trident)\/(\d+)/)) {
|
||||
return false;
|
||||
}
|
||||
|
||||
// Is webkit? http://stackoverflow.com/a/16459606/376773
|
||||
// document is undefined in react-native: https://github.com/facebook/react-native/pull/1632
|
||||
return (typeof document !== 'undefined' && document.documentElement && document.documentElement.style && document.documentElement.style.WebkitAppearance) ||
|
||||
// Is firebug? http://stackoverflow.com/a/398120/376773
|
||||
(typeof window !== 'undefined' && window.console && (window.console.firebug || (window.console.exception && window.console.table))) ||
|
||||
// Is firefox >= v31?
|
||||
// https://developer.mozilla.org/en-US/docs/Tools/Web_Console#Styling_messages
|
||||
(typeof navigator !== 'undefined' && navigator.userAgent && navigator.userAgent.toLowerCase().match(/firefox\/(\d+)/) && parseInt(RegExp.$1, 10) >= 31) ||
|
||||
// Double check webkit in userAgent just in case we are in a worker
|
||||
(typeof navigator !== 'undefined' && navigator.userAgent && navigator.userAgent.toLowerCase().match(/applewebkit\/(\d+)/));
|
||||
}
|
||||
|
||||
/**
|
||||
* Colorize log arguments if enabled.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function formatArgs(args) {
|
||||
args[0] = (this.useColors ? '%c' : '') +
|
||||
this.namespace +
|
||||
(this.useColors ? ' %c' : ' ') +
|
||||
args[0] +
|
||||
(this.useColors ? '%c ' : ' ') +
|
||||
'+' + module.exports.humanize(this.diff);
|
||||
|
||||
if (!this.useColors) {
|
||||
return;
|
||||
}
|
||||
|
||||
const c = 'color: ' + this.color;
|
||||
args.splice(1, 0, c, 'color: inherit');
|
||||
|
||||
// The final "%c" is somewhat tricky, because there could be other
|
||||
// arguments passed either before or after the %c, so we need to
|
||||
// figure out the correct index to insert the CSS into
|
||||
let index = 0;
|
||||
let lastC = 0;
|
||||
args[0].replace(/%[a-zA-Z%]/g, match => {
|
||||
if (match === '%%') {
|
||||
return;
|
||||
}
|
||||
index++;
|
||||
if (match === '%c') {
|
||||
// We only are interested in the *last* %c
|
||||
// (the user may have provided their own)
|
||||
lastC = index;
|
||||
}
|
||||
});
|
||||
|
||||
args.splice(lastC, 0, c);
|
||||
}
|
||||
|
||||
/**
|
||||
* Invokes `console.debug()` when available.
|
||||
* No-op when `console.debug` is not a "function".
|
||||
* If `console.debug` is not available, falls back
|
||||
* to `console.log`.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
exports.log = console.debug || console.log || (() => {});
|
||||
|
||||
/**
|
||||
* Save `namespaces`.
|
||||
*
|
||||
* @param {String} namespaces
|
||||
* @api private
|
||||
*/
|
||||
function save(namespaces) {
|
||||
try {
|
||||
if (namespaces) {
|
||||
exports.storage.setItem('debug', namespaces);
|
||||
} else {
|
||||
exports.storage.removeItem('debug');
|
||||
}
|
||||
} catch (error) {
|
||||
// Swallow
|
||||
// XXX (@Qix-) should we be logging these?
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Load `namespaces`.
|
||||
*
|
||||
* @return {String} returns the previously persisted debug modes
|
||||
* @api private
|
||||
*/
|
||||
function load() {
|
||||
let r;
|
||||
try {
|
||||
r = exports.storage.getItem('debug');
|
||||
} catch (error) {
|
||||
// Swallow
|
||||
// XXX (@Qix-) should we be logging these?
|
||||
}
|
||||
|
||||
// If debug isn't set in LS, and we're in Electron, try to load $DEBUG
|
||||
if (!r && typeof process !== 'undefined' && 'env' in process) {
|
||||
r = process.env.DEBUG;
|
||||
}
|
||||
|
||||
return r;
|
||||
}
|
||||
|
||||
/**
|
||||
* Localstorage attempts to return the localstorage.
|
||||
*
|
||||
* This is necessary because safari throws
|
||||
* when a user disables cookies/localstorage
|
||||
* and you attempt to access it.
|
||||
*
|
||||
* @return {LocalStorage}
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function localstorage() {
|
||||
try {
|
||||
// TVMLKit (Apple TV JS Runtime) does not have a window object, just localStorage in the global context
|
||||
// The Browser also has localStorage in the global context.
|
||||
return localStorage;
|
||||
} catch (error) {
|
||||
// Swallow
|
||||
// XXX (@Qix-) should we be logging these?
|
||||
}
|
||||
}
|
||||
|
||||
module.exports = require('./common')(exports);
|
||||
|
||||
const {formatters} = module.exports;
|
||||
|
||||
/**
|
||||
* Map %j to `JSON.stringify()`, since no Web Inspectors do that by default.
|
||||
*/
|
||||
|
||||
formatters.j = function (v) {
|
||||
try {
|
||||
return JSON.stringify(v);
|
||||
} catch (error) {
|
||||
return '[UnexpectedJSONParseError]: ' + error.message;
|
||||
}
|
||||
};
|
|
@ -0,0 +1,274 @@
|
|||
|
||||
/**
|
||||
* This is the common logic for both the Node.js and web browser
|
||||
* implementations of `debug()`.
|
||||
*/
|
||||
|
||||
function setup(env) {
|
||||
createDebug.debug = createDebug;
|
||||
createDebug.default = createDebug;
|
||||
createDebug.coerce = coerce;
|
||||
createDebug.disable = disable;
|
||||
createDebug.enable = enable;
|
||||
createDebug.enabled = enabled;
|
||||
createDebug.humanize = require('ms');
|
||||
createDebug.destroy = destroy;
|
||||
|
||||
Object.keys(env).forEach(key => {
|
||||
createDebug[key] = env[key];
|
||||
});
|
||||
|
||||
/**
|
||||
* The currently active debug mode names, and names to skip.
|
||||
*/
|
||||
|
||||
createDebug.names = [];
|
||||
createDebug.skips = [];
|
||||
|
||||
/**
|
||||
* Map of special "%n" handling functions, for the debug "format" argument.
|
||||
*
|
||||
* Valid key names are a single, lower or upper-case letter, i.e. "n" and "N".
|
||||
*/
|
||||
createDebug.formatters = {};
|
||||
|
||||
/**
|
||||
* Selects a color for a debug namespace
|
||||
* @param {String} namespace The namespace string for the debug instance to be colored
|
||||
* @return {Number|String} An ANSI color code for the given namespace
|
||||
* @api private
|
||||
*/
|
||||
function selectColor(namespace) {
|
||||
let hash = 0;
|
||||
|
||||
for (let i = 0; i < namespace.length; i++) {
|
||||
hash = ((hash << 5) - hash) + namespace.charCodeAt(i);
|
||||
hash |= 0; // Convert to 32bit integer
|
||||
}
|
||||
|
||||
return createDebug.colors[Math.abs(hash) % createDebug.colors.length];
|
||||
}
|
||||
createDebug.selectColor = selectColor;
|
||||
|
||||
/**
|
||||
* Create a debugger with the given `namespace`.
|
||||
*
|
||||
* @param {String} namespace
|
||||
* @return {Function}
|
||||
* @api public
|
||||
*/
|
||||
function createDebug(namespace) {
|
||||
let prevTime;
|
||||
let enableOverride = null;
|
||||
let namespacesCache;
|
||||
let enabledCache;
|
||||
|
||||
function debug(...args) {
|
||||
// Disabled?
|
||||
if (!debug.enabled) {
|
||||
return;
|
||||
}
|
||||
|
||||
const self = debug;
|
||||
|
||||
// Set `diff` timestamp
|
||||
const curr = Number(new Date());
|
||||
const ms = curr - (prevTime || curr);
|
||||
self.diff = ms;
|
||||
self.prev = prevTime;
|
||||
self.curr = curr;
|
||||
prevTime = curr;
|
||||
|
||||
args[0] = createDebug.coerce(args[0]);
|
||||
|
||||
if (typeof args[0] !== 'string') {
|
||||
// Anything else let's inspect with %O
|
||||
args.unshift('%O');
|
||||
}
|
||||
|
||||
// Apply any `formatters` transformations
|
||||
let index = 0;
|
||||
args[0] = args[0].replace(/%([a-zA-Z%])/g, (match, format) => {
|
||||
// If we encounter an escaped % then don't increase the array index
|
||||
if (match === '%%') {
|
||||
return '%';
|
||||
}
|
||||
index++;
|
||||
const formatter = createDebug.formatters[format];
|
||||
if (typeof formatter === 'function') {
|
||||
const val = args[index];
|
||||
match = formatter.call(self, val);
|
||||
|
||||
// Now we need to remove `args[index]` since it's inlined in the `format`
|
||||
args.splice(index, 1);
|
||||
index--;
|
||||
}
|
||||
return match;
|
||||
});
|
||||
|
||||
// Apply env-specific formatting (colors, etc.)
|
||||
createDebug.formatArgs.call(self, args);
|
||||
|
||||
const logFn = self.log || createDebug.log;
|
||||
logFn.apply(self, args);
|
||||
}
|
||||
|
||||
debug.namespace = namespace;
|
||||
debug.useColors = createDebug.useColors();
|
||||
debug.color = createDebug.selectColor(namespace);
|
||||
debug.extend = extend;
|
||||
debug.destroy = createDebug.destroy; // XXX Temporary. Will be removed in the next major release.
|
||||
|
||||
Object.defineProperty(debug, 'enabled', {
|
||||
enumerable: true,
|
||||
configurable: false,
|
||||
get: () => {
|
||||
if (enableOverride !== null) {
|
||||
return enableOverride;
|
||||
}
|
||||
if (namespacesCache !== createDebug.namespaces) {
|
||||
namespacesCache = createDebug.namespaces;
|
||||
enabledCache = createDebug.enabled(namespace);
|
||||
}
|
||||
|
||||
return enabledCache;
|
||||
},
|
||||
set: v => {
|
||||
enableOverride = v;
|
||||
}
|
||||
});
|
||||
|
||||
// Env-specific initialization logic for debug instances
|
||||
if (typeof createDebug.init === 'function') {
|
||||
createDebug.init(debug);
|
||||
}
|
||||
|
||||
return debug;
|
||||
}
|
||||
|
||||
function extend(namespace, delimiter) {
|
||||
const newDebug = createDebug(this.namespace + (typeof delimiter === 'undefined' ? ':' : delimiter) + namespace);
|
||||
newDebug.log = this.log;
|
||||
return newDebug;
|
||||
}
|
||||
|
||||
/**
|
||||
* Enables a debug mode by namespaces. This can include modes
|
||||
* separated by a colon and wildcards.
|
||||
*
|
||||
* @param {String} namespaces
|
||||
* @api public
|
||||
*/
|
||||
function enable(namespaces) {
|
||||
createDebug.save(namespaces);
|
||||
createDebug.namespaces = namespaces;
|
||||
|
||||
createDebug.names = [];
|
||||
createDebug.skips = [];
|
||||
|
||||
let i;
|
||||
const split = (typeof namespaces === 'string' ? namespaces : '').split(/[\s,]+/);
|
||||
const len = split.length;
|
||||
|
||||
for (i = 0; i < len; i++) {
|
||||
if (!split[i]) {
|
||||
// ignore empty strings
|
||||
continue;
|
||||
}
|
||||
|
||||
namespaces = split[i].replace(/\*/g, '.*?');
|
||||
|
||||
if (namespaces[0] === '-') {
|
||||
createDebug.skips.push(new RegExp('^' + namespaces.slice(1) + '$'));
|
||||
} else {
|
||||
createDebug.names.push(new RegExp('^' + namespaces + '$'));
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Disable debug output.
|
||||
*
|
||||
* @return {String} namespaces
|
||||
* @api public
|
||||
*/
|
||||
function disable() {
|
||||
const namespaces = [
|
||||
...createDebug.names.map(toNamespace),
|
||||
...createDebug.skips.map(toNamespace).map(namespace => '-' + namespace)
|
||||
].join(',');
|
||||
createDebug.enable('');
|
||||
return namespaces;
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns true if the given mode name is enabled, false otherwise.
|
||||
*
|
||||
* @param {String} name
|
||||
* @return {Boolean}
|
||||
* @api public
|
||||
*/
|
||||
function enabled(name) {
|
||||
if (name[name.length - 1] === '*') {
|
||||
return true;
|
||||
}
|
||||
|
||||
let i;
|
||||
let len;
|
||||
|
||||
for (i = 0, len = createDebug.skips.length; i < len; i++) {
|
||||
if (createDebug.skips[i].test(name)) {
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
for (i = 0, len = createDebug.names.length; i < len; i++) {
|
||||
if (createDebug.names[i].test(name)) {
|
||||
return true;
|
||||
}
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
/**
|
||||
* Convert regexp to namespace
|
||||
*
|
||||
* @param {RegExp} regxep
|
||||
* @return {String} namespace
|
||||
* @api private
|
||||
*/
|
||||
function toNamespace(regexp) {
|
||||
return regexp.toString()
|
||||
.substring(2, regexp.toString().length - 2)
|
||||
.replace(/\.\*\?$/, '*');
|
||||
}
|
||||
|
||||
/**
|
||||
* Coerce `val`.
|
||||
*
|
||||
* @param {Mixed} val
|
||||
* @return {Mixed}
|
||||
* @api private
|
||||
*/
|
||||
function coerce(val) {
|
||||
if (val instanceof Error) {
|
||||
return val.stack || val.message;
|
||||
}
|
||||
return val;
|
||||
}
|
||||
|
||||
/**
|
||||
* XXX DO NOT USE. This is a temporary stub function.
|
||||
* XXX It WILL be removed in the next major release.
|
||||
*/
|
||||
function destroy() {
|
||||
console.warn('Instance method `debug.destroy()` is deprecated and no longer does anything. It will be removed in the next major version of `debug`.');
|
||||
}
|
||||
|
||||
createDebug.enable(createDebug.load());
|
||||
|
||||
return createDebug;
|
||||
}
|
||||
|
||||
module.exports = setup;
|
|
@ -0,0 +1,10 @@
|
|||
/**
|
||||
* Detect Electron renderer / nwjs process, which is node, but we should
|
||||
* treat as a browser.
|
||||
*/
|
||||
|
||||
if (typeof process === 'undefined' || process.type === 'renderer' || process.browser === true || process.__nwjs) {
|
||||
module.exports = require('./browser.js');
|
||||
} else {
|
||||
module.exports = require('./node.js');
|
||||
}
|
|
@ -0,0 +1,263 @@
|
|||
/**
|
||||
* Module dependencies.
|
||||
*/
|
||||
|
||||
const tty = require('tty');
|
||||
const util = require('util');
|
||||
|
||||
/**
|
||||
* This is the Node.js implementation of `debug()`.
|
||||
*/
|
||||
|
||||
exports.init = init;
|
||||
exports.log = log;
|
||||
exports.formatArgs = formatArgs;
|
||||
exports.save = save;
|
||||
exports.load = load;
|
||||
exports.useColors = useColors;
|
||||
exports.destroy = util.deprecate(
|
||||
() => {},
|
||||
'Instance method `debug.destroy()` is deprecated and no longer does anything. It will be removed in the next major version of `debug`.'
|
||||
);
|
||||
|
||||
/**
|
||||
* Colors.
|
||||
*/
|
||||
|
||||
exports.colors = [6, 2, 3, 4, 5, 1];
|
||||
|
||||
try {
|
||||
// Optional dependency (as in, doesn't need to be installed, NOT like optionalDependencies in package.json)
|
||||
// eslint-disable-next-line import/no-extraneous-dependencies
|
||||
const supportsColor = require('supports-color');
|
||||
|
||||
if (supportsColor && (supportsColor.stderr || supportsColor).level >= 2) {
|
||||
exports.colors = [
|
||||
20,
|
||||
21,
|
||||
26,
|
||||
27,
|
||||
32,
|
||||
33,
|
||||
38,
|
||||
39,
|
||||
40,
|
||||
41,
|
||||
42,
|
||||
43,
|
||||
44,
|
||||
45,
|
||||
56,
|
||||
57,
|
||||
62,
|
||||
63,
|
||||
68,
|
||||
69,
|
||||
74,
|
||||
75,
|
||||
76,
|
||||
77,
|
||||
78,
|
||||
79,
|
||||
80,
|
||||
81,
|
||||
92,
|
||||
93,
|
||||
98,
|
||||
99,
|
||||
112,
|
||||
113,
|
||||
128,
|
||||
129,
|
||||
134,
|
||||
135,
|
||||
148,
|
||||
149,
|
||||
160,
|
||||
161,
|
||||
162,
|
||||
163,
|
||||
164,
|
||||
165,
|
||||
166,
|
||||
167,
|
||||
168,
|
||||
169,
|
||||
170,
|
||||
171,
|
||||
172,
|
||||
173,
|
||||
178,
|
||||
179,
|
||||
184,
|
||||
185,
|
||||
196,
|
||||
197,
|
||||
198,
|
||||
199,
|
||||
200,
|
||||
201,
|
||||
202,
|
||||
203,
|
||||
204,
|
||||
205,
|
||||
206,
|
||||
207,
|
||||
208,
|
||||
209,
|
||||
214,
|
||||
215,
|
||||
220,
|
||||
221
|
||||
];
|
||||
}
|
||||
} catch (error) {
|
||||
// Swallow - we only care if `supports-color` is available; it doesn't have to be.
|
||||
}
|
||||
|
||||
/**
|
||||
* Build up the default `inspectOpts` object from the environment variables.
|
||||
*
|
||||
* $ DEBUG_COLORS=no DEBUG_DEPTH=10 DEBUG_SHOW_HIDDEN=enabled node script.js
|
||||
*/
|
||||
|
||||
exports.inspectOpts = Object.keys(process.env).filter(key => {
|
||||
return /^debug_/i.test(key);
|
||||
}).reduce((obj, key) => {
|
||||
// Camel-case
|
||||
const prop = key
|
||||
.substring(6)
|
||||
.toLowerCase()
|
||||
.replace(/_([a-z])/g, (_, k) => {
|
||||
return k.toUpperCase();
|
||||
});
|
||||
|
||||
// Coerce string value into JS value
|
||||
let val = process.env[key];
|
||||
if (/^(yes|on|true|enabled)$/i.test(val)) {
|
||||
val = true;
|
||||
} else if (/^(no|off|false|disabled)$/i.test(val)) {
|
||||
val = false;
|
||||
} else if (val === 'null') {
|
||||
val = null;
|
||||
} else {
|
||||
val = Number(val);
|
||||
}
|
||||
|
||||
obj[prop] = val;
|
||||
return obj;
|
||||
}, {});
|
||||
|
||||
/**
|
||||
* Is stdout a TTY? Colored output is enabled when `true`.
|
||||
*/
|
||||
|
||||
function useColors() {
|
||||
return 'colors' in exports.inspectOpts ?
|
||||
Boolean(exports.inspectOpts.colors) :
|
||||
tty.isatty(process.stderr.fd);
|
||||
}
|
||||
|
||||
/**
|
||||
* Adds ANSI color escape codes if enabled.
|
||||
*
|
||||
* @api public
|
||||
*/
|
||||
|
||||
function formatArgs(args) {
|
||||
const {namespace: name, useColors} = this;
|
||||
|
||||
if (useColors) {
|
||||
const c = this.color;
|
||||
const colorCode = '\u001B[3' + (c < 8 ? c : '8;5;' + c);
|
||||
const prefix = ` ${colorCode};1m${name} \u001B[0m`;
|
||||
|
||||
args[0] = prefix + args[0].split('\n').join('\n' + prefix);
|
||||
args.push(colorCode + 'm+' + module.exports.humanize(this.diff) + '\u001B[0m');
|
||||
} else {
|
||||
args[0] = getDate() + name + ' ' + args[0];
|
||||
}
|
||||
}
|
||||
|
||||
function getDate() {
|
||||
if (exports.inspectOpts.hideDate) {
|
||||
return '';
|
||||
}
|
||||
return new Date().toISOString() + ' ';
|
||||
}
|
||||
|
||||
/**
|
||||
* Invokes `util.format()` with the specified arguments and writes to stderr.
|
||||
*/
|
||||
|
||||
function log(...args) {
|
||||
return process.stderr.write(util.format(...args) + '\n');
|
||||
}
|
||||
|
||||
/**
|
||||
* Save `namespaces`.
|
||||
*
|
||||
* @param {String} namespaces
|
||||
* @api private
|
||||
*/
|
||||
function save(namespaces) {
|
||||
if (namespaces) {
|
||||
process.env.DEBUG = namespaces;
|
||||
} else {
|
||||
// If you set a process.env field to null or undefined, it gets cast to the
|
||||
// string 'null' or 'undefined'. Just delete instead.
|
||||
delete process.env.DEBUG;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Load `namespaces`.
|
||||
*
|
||||
* @return {String} returns the previously persisted debug modes
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function load() {
|
||||
return process.env.DEBUG;
|
||||
}
|
||||
|
||||
/**
|
||||
* Init logic for `debug` instances.
|
||||
*
|
||||
* Create a new `inspectOpts` object in case `useColors` is set
|
||||
* differently for a particular `debug` instance.
|
||||
*/
|
||||
|
||||
function init(debug) {
|
||||
debug.inspectOpts = {};
|
||||
|
||||
const keys = Object.keys(exports.inspectOpts);
|
||||
for (let i = 0; i < keys.length; i++) {
|
||||
debug.inspectOpts[keys[i]] = exports.inspectOpts[keys[i]];
|
||||
}
|
||||
}
|
||||
|
||||
module.exports = require('./common')(exports);
|
||||
|
||||
const {formatters} = module.exports;
|
||||
|
||||
/**
|
||||
* Map %o to `util.inspect()`, all on a single line.
|
||||
*/
|
||||
|
||||
formatters.o = function (v) {
|
||||
this.inspectOpts.colors = this.useColors;
|
||||
return util.inspect(v, this.inspectOpts)
|
||||
.split('\n')
|
||||
.map(str => str.trim())
|
||||
.join(' ');
|
||||
};
|
||||
|
||||
/**
|
||||
* Map %O to `util.inspect()`, allowing multiple lines if needed.
|
||||
*/
|
||||
|
||||
formatters.O = function (v) {
|
||||
this.inspectOpts.colors = this.useColors;
|
||||
return util.inspect(v, this.inspectOpts);
|
||||
};
|
|
@ -0,0 +1,39 @@
|
|||
# How to Contribute
|
||||
|
||||
## Pull Requests
|
||||
|
||||
We also accept [pull requests][pull-request]!
|
||||
|
||||
Generally we like to see pull requests that
|
||||
- Maintain the existing code style
|
||||
- Are focused on a single change (i.e. avoid large refactoring or style adjustments in untouched code if not the primary goal of the pull request)
|
||||
- Have [good commit messages](http://tbaggery.com/2008/04/19/a-note-about-git-commit-messages.html)
|
||||
- Have tests
|
||||
- Don't decrease the current code coverage (see coverage/lcov-report/index.html)
|
||||
|
||||
## Building
|
||||
|
||||
```
|
||||
npm install
|
||||
npm test
|
||||
````
|
||||
|
||||
The `npm test -- dev` implements watching for tests within Node and `karma start` may be used for manual testing in browsers.
|
||||
|
||||
If you notice any problems, please report them to the GitHub issue tracker at
|
||||
[http://github.com/kpdecker/jsdiff/issues](http://github.com/kpdecker/jsdiff/issues).
|
||||
|
||||
## Releasing
|
||||
|
||||
JsDiff utilizes the [release yeoman generator][generator-release] to perform most release tasks.
|
||||
|
||||
A full release may be completed with the following:
|
||||
|
||||
```
|
||||
yo release
|
||||
npm publish
|
||||
yo release:publish components jsdiff dist/components/
|
||||
```
|
||||
|
||||
[generator-release]: https://github.com/walmartlabs/generator-release
|
||||
[pull-request]: https://github.com/kpdecker/jsdiff/pull/new/master
|
|
@ -0,0 +1,31 @@
|
|||
Software License Agreement (BSD License)
|
||||
|
||||
Copyright (c) 2009-2015, Kevin Decker <kpdecker@gmail.com>
|
||||
|
||||
All rights reserved.
|
||||
|
||||
Redistribution and use of this software in source and binary forms, with or without modification,
|
||||
are permitted provided that the following conditions are met:
|
||||
|
||||
* Redistributions of source code must retain the above
|
||||
copyright notice, this list of conditions and the
|
||||
following disclaimer.
|
||||
|
||||
* Redistributions in binary form must reproduce the above
|
||||
copyright notice, this list of conditions and the
|
||||
following disclaimer in the documentation and/or other
|
||||
materials provided with the distribution.
|
||||
|
||||
* Neither the name of Kevin Decker nor the names of its
|
||||
contributors may be used to endorse or promote products
|
||||
derived from this software without specific prior
|
||||
written permission.
|
||||
|
||||
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR
|
||||
IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND
|
||||
FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
|
||||
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
|
||||
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
|
||||
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER
|
||||
IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT
|
||||
OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
|
@ -0,0 +1,211 @@
|
|||
# jsdiff
|
||||
|
||||
[](http://travis-ci.org/kpdecker/jsdiff)
|
||||
[](https://saucelabs.com/u/jsdiff)
|
||||
|
||||
A javascript text differencing implementation.
|
||||
|
||||
Based on the algorithm proposed in
|
||||
["An O(ND) Difference Algorithm and its Variations" (Myers, 1986)](http://citeseerx.ist.psu.edu/viewdoc/summary?doi=10.1.1.4.6927).
|
||||
|
||||
## Installation
|
||||
```bash
|
||||
npm install diff --save
|
||||
```
|
||||
or
|
||||
```bash
|
||||
bower install jsdiff --save
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
* `JsDiff.diffChars(oldStr, newStr[, options])` - diffs two blocks of text, comparing character by character.
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
Options
|
||||
* `ignoreCase`: `true` to ignore casing difference. Defaults to `false`.
|
||||
|
||||
* `JsDiff.diffWords(oldStr, newStr[, options])` - diffs two blocks of text, comparing word by word, ignoring whitespace.
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
Options
|
||||
* `ignoreCase`: Same as in `diffChars`.
|
||||
|
||||
* `JsDiff.diffWordsWithSpace(oldStr, newStr[, options])` - diffs two blocks of text, comparing word by word, treating whitespace as significant.
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
* `JsDiff.diffLines(oldStr, newStr[, options])` - diffs two blocks of text, comparing line by line.
|
||||
|
||||
Options
|
||||
* `ignoreWhitespace`: `true` to ignore leading and trailing whitespace. This is the same as `diffTrimmedLines`
|
||||
* `newlineIsToken`: `true` to treat newline characters as separate tokens. This allows for changes to the newline structure to occur independently of the line content and to be treated as such. In general this is the more human friendly form of `diffLines` and `diffLines` is better suited for patches and other computer friendly output.
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
* `JsDiff.diffTrimmedLines(oldStr, newStr[, options])` - diffs two blocks of text, comparing line by line, ignoring leading and trailing whitespace.
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
* `JsDiff.diffSentences(oldStr, newStr[, options])` - diffs two blocks of text, comparing sentence by sentence.
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
* `JsDiff.diffCss(oldStr, newStr[, options])` - diffs two blocks of text, comparing CSS tokens.
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
* `JsDiff.diffJson(oldObj, newObj[, options])` - diffs two JSON objects, comparing the fields defined on each. The order of fields, etc does not matter in this comparison.
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
* `JsDiff.diffArrays(oldArr, newArr[, options])` - diffs two arrays, comparing each item for strict equality (===).
|
||||
|
||||
Options
|
||||
* `comparator`: `function(left, right)` for custom equality checks
|
||||
|
||||
Returns a list of change objects (See below).
|
||||
|
||||
* `JsDiff.createTwoFilesPatch(oldFileName, newFileName, oldStr, newStr, oldHeader, newHeader)` - creates a unified diff patch.
|
||||
|
||||
Parameters:
|
||||
* `oldFileName` : String to be output in the filename section of the patch for the removals
|
||||
* `newFileName` : String to be output in the filename section of the patch for the additions
|
||||
* `oldStr` : Original string value
|
||||
* `newStr` : New string value
|
||||
* `oldHeader` : Additional information to include in the old file header
|
||||
* `newHeader` : Additional information to include in the new file header
|
||||
* `options` : An object with options. Currently, only `context` is supported and describes how many lines of context should be included.
|
||||
|
||||
* `JsDiff.createPatch(fileName, oldStr, newStr, oldHeader, newHeader)` - creates a unified diff patch.
|
||||
|
||||
Just like JsDiff.createTwoFilesPatch, but with oldFileName being equal to newFileName.
|
||||
|
||||
|
||||
* `JsDiff.structuredPatch(oldFileName, newFileName, oldStr, newStr, oldHeader, newHeader, options)` - returns an object with an array of hunk objects.
|
||||
|
||||
This method is similar to createTwoFilesPatch, but returns a data structure
|
||||
suitable for further processing. Parameters are the same as createTwoFilesPatch. The data structure returned may look like this:
|
||||
|
||||
```js
|
||||
{
|
||||
oldFileName: 'oldfile', newFileName: 'newfile',
|
||||
oldHeader: 'header1', newHeader: 'header2',
|
||||
hunks: [{
|
||||
oldStart: 1, oldLines: 3, newStart: 1, newLines: 3,
|
||||
lines: [' line2', ' line3', '-line4', '+line5', '\\ No newline at end of file'],
|
||||
}]
|
||||
}
|
||||
```
|
||||
|
||||
* `JsDiff.applyPatch(source, patch[, options])` - applies a unified diff patch.
|
||||
|
||||
Return a string containing new version of provided data. `patch` may be a string diff or the output from the `parsePatch` or `structuredPatch` methods.
|
||||
|
||||
The optional `options` object may have the following keys:
|
||||
|
||||
- `fuzzFactor`: Number of lines that are allowed to differ before rejecting a patch. Defaults to 0.
|
||||
- `compareLine(lineNumber, line, operation, patchContent)`: Callback used to compare to given lines to determine if they should be considered equal when patching. Defaults to strict equality but may be overridden to provide fuzzier comparison. Should return false if the lines should be rejected.
|
||||
|
||||
* `JsDiff.applyPatches(patch, options)` - applies one or more patches.
|
||||
|
||||
This method will iterate over the contents of the patch and apply to data provided through callbacks. The general flow for each patch index is:
|
||||
|
||||
- `options.loadFile(index, callback)` is called. The caller should then load the contents of the file and then pass that to the `callback(err, data)` callback. Passing an `err` will terminate further patch execution.
|
||||
- `options.patched(index, content, callback)` is called once the patch has been applied. `content` will be the return value from `applyPatch`. When it's ready, the caller should call `callback(err)` callback. Passing an `err` will terminate further patch execution.
|
||||
|
||||
Once all patches have been applied or an error occurs, the `options.complete(err)` callback is made.
|
||||
|
||||
* `JsDiff.parsePatch(diffStr)` - Parses a patch into structured data
|
||||
|
||||
Return a JSON object representation of the a patch, suitable for use with the `applyPatch` method. This parses to the same structure returned by `JsDiff.structuredPatch`.
|
||||
|
||||
* `convertChangesToXML(changes)` - converts a list of changes to a serialized XML format
|
||||
|
||||
|
||||
All methods above which accept the optional `callback` method will run in sync mode when that parameter is omitted and in async mode when supplied. This allows for larger diffs without blocking the event loop. This may be passed either directly as the final parameter or as the `callback` field in the `options` object.
|
||||
|
||||
### Change Objects
|
||||
Many of the methods above return change objects. These objects consist of the following fields:
|
||||
|
||||
* `value`: Text content
|
||||
* `added`: True if the value was inserted into the new string
|
||||
* `removed`: True of the value was removed from the old string
|
||||
|
||||
Note that some cases may omit a particular flag field. Comparison on the flag fields should always be done in a truthy or falsy manner.
|
||||
|
||||
## Examples
|
||||
|
||||
Basic example in Node
|
||||
|
||||
```js
|
||||
require('colors');
|
||||
var jsdiff = require('diff');
|
||||
|
||||
var one = 'beep boop';
|
||||
var other = 'beep boob blah';
|
||||
|
||||
var diff = jsdiff.diffChars(one, other);
|
||||
|
||||
diff.forEach(function(part){
|
||||
// green for additions, red for deletions
|
||||
// grey for common parts
|
||||
var color = part.added ? 'green' :
|
||||
part.removed ? 'red' : 'grey';
|
||||
process.stderr.write(part.value[color]);
|
||||
});
|
||||
|
||||
console.log();
|
||||
```
|
||||
Running the above program should yield
|
||||
|
||||
<img src="images/node_example.png" alt="Node Example">
|
||||
|
||||
Basic example in a web page
|
||||
|
||||
```html
|
||||
<pre id="display"></pre>
|
||||
<script src="diff.js"></script>
|
||||
<script>
|
||||
var one = 'beep boop',
|
||||
other = 'beep boob blah',
|
||||
color = '',
|
||||
span = null;
|
||||
|
||||
var diff = JsDiff.diffChars(one, other),
|
||||
display = document.getElementById('display'),
|
||||
fragment = document.createDocumentFragment();
|
||||
|
||||
diff.forEach(function(part){
|
||||
// green for additions, red for deletions
|
||||
// grey for common parts
|
||||
color = part.added ? 'green' :
|
||||
part.removed ? 'red' : 'grey';
|
||||
span = document.createElement('span');
|
||||
span.style.color = color;
|
||||
span.appendChild(document
|
||||
.createTextNode(part.value));
|
||||
fragment.appendChild(span);
|
||||
});
|
||||
|
||||
display.appendChild(fragment);
|
||||
</script>
|
||||
```
|
||||
|
||||
Open the above .html file in a browser and you should see
|
||||
|
||||
<img src="images/web_example.png" alt="Node Example">
|
||||
|
||||
**[Full online demo](http://kpdecker.github.com/jsdiff)**
|
||||
|
||||
## Compatibility
|
||||
|
||||
[](https://saucelabs.com/u/jsdiff)
|
||||
|
||||
jsdiff supports all ES3 environments with some known issues on IE8 and below. Under these browsers some diff algorithms such as word diff and others may fail due to lack of support for capturing groups in the `split` operation.
|
||||
|
||||
## License
|
||||
|
||||
See [LICENSE](https://github.com/kpdecker/jsdiff/blob/master/LICENSE).
|
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,416 @@
|
|||
/*!
|
||||
|
||||
diff v3.5.0
|
||||
|
||||
Software License Agreement (BSD License)
|
||||
|
||||
Copyright (c) 2009-2015, Kevin Decker <kpdecker@gmail.com>
|
||||
|
||||
All rights reserved.
|
||||
|
||||
Redistribution and use of this software in source and binary forms, with or without modification,
|
||||
are permitted provided that the following conditions are met:
|
||||
|
||||
* Redistributions of source code must retain the above
|
||||
copyright notice, this list of conditions and the
|
||||
following disclaimer.
|
||||
|
||||
* Redistributions in binary form must reproduce the above
|
||||
copyright notice, this list of conditions and the
|
||||
following disclaimer in the documentation and/or other
|
||||
materials provided with the distribution.
|
||||
|
||||
* Neither the name of Kevin Decker nor the names of its
|
||||
contributors may be used to endorse or promote products
|
||||
derived from this software without specific prior
|
||||
written permission.
|
||||
|
||||
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR
|
||||
IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND
|
||||
FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
|
||||
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
|
||||
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
|
||||
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER
|
||||
IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT
|
||||
OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
||||
@license
|
||||
*/
|
||||
!function(a,b){"object"==typeof exports&&"object"==typeof module?module.exports=b():"function"==typeof define&&define.amd?define([],b):"object"==typeof exports?exports.JsDiff=b():a.JsDiff=b()}(this,function(){/******/
|
||||
return function(a){/******/
|
||||
// The require function
|
||||
/******/
|
||||
function b(d){/******/
|
||||
// Check if module is in cache
|
||||
/******/
|
||||
if(c[d])/******/
|
||||
return c[d].exports;/******/
|
||||
// Create a new module (and put it into the cache)
|
||||
/******/
|
||||
var e=c[d]={/******/
|
||||
exports:{},/******/
|
||||
id:d,/******/
|
||||
loaded:!1};/******/
|
||||
// Return the exports of the module
|
||||
/******/
|
||||
/******/
|
||||
// Execute the module function
|
||||
/******/
|
||||
/******/
|
||||
// Flag the module as loaded
|
||||
/******/
|
||||
return a[d].call(e.exports,e,e.exports,b),e.loaded=!0,e.exports}// webpackBootstrap
|
||||
/******/
|
||||
// The module cache
|
||||
/******/
|
||||
var c={};/******/
|
||||
// Load entry module and return exports
|
||||
/******/
|
||||
/******/
|
||||
// expose the modules object (__webpack_modules__)
|
||||
/******/
|
||||
/******/
|
||||
// expose the module cache
|
||||
/******/
|
||||
/******/
|
||||
// __webpack_public_path__
|
||||
/******/
|
||||
return b.m=a,b.c=c,b.p="",b(0)}([/* 0 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";/*istanbul ignore start*/
|
||||
function d(a){return a&&a.__esModule?a:{"default":a}}b.__esModule=!0,b.canonicalize=b.convertChangesToXML=b.convertChangesToDMP=b.merge=b.parsePatch=b.applyPatches=b.applyPatch=b.createPatch=b.createTwoFilesPatch=b.structuredPatch=b.diffArrays=b.diffJson=b.diffCss=b.diffSentences=b.diffTrimmedLines=b.diffLines=b.diffWordsWithSpace=b.diffWords=b.diffChars=b.Diff=void 0;/*istanbul ignore end*/
|
||||
var/*istanbul ignore start*/e=c(1),f=d(e),/*istanbul ignore start*/g=c(2),/*istanbul ignore start*/h=c(3),/*istanbul ignore start*/i=c(5),/*istanbul ignore start*/j=c(6),/*istanbul ignore start*/k=c(7),/*istanbul ignore start*/l=c(8),/*istanbul ignore start*/m=c(9),/*istanbul ignore start*/n=c(10),/*istanbul ignore start*/o=c(11),/*istanbul ignore start*/p=c(13),/*istanbul ignore start*/q=c(14),/*istanbul ignore start*/r=c(16),/*istanbul ignore start*/s=c(17);/* See LICENSE file for terms of use */
|
||||
/*
|
||||
* Text diff implementation.
|
||||
*
|
||||
* This library supports the following APIS:
|
||||
* JsDiff.diffChars: Character by character diff
|
||||
* JsDiff.diffWords: Word (as defined by \b regex) diff which ignores whitespace
|
||||
* JsDiff.diffLines: Line based diff
|
||||
*
|
||||
* JsDiff.diffCss: Diff targeted at CSS content
|
||||
*
|
||||
* These methods are based on the implementation proposed in
|
||||
* "An O(ND) Difference Algorithm and its Variations" (Myers, 1986).
|
||||
* http://citeseerx.ist.psu.edu/viewdoc/summary?doi=10.1.1.4.6927
|
||||
*/
|
||||
b.Diff=f["default"],/*istanbul ignore start*/
|
||||
b.diffChars=g.diffChars,/*istanbul ignore start*/
|
||||
b.diffWords=h.diffWords,/*istanbul ignore start*/
|
||||
b.diffWordsWithSpace=h.diffWordsWithSpace,/*istanbul ignore start*/
|
||||
b.diffLines=i.diffLines,/*istanbul ignore start*/
|
||||
b.diffTrimmedLines=i.diffTrimmedLines,/*istanbul ignore start*/
|
||||
b.diffSentences=j.diffSentences,/*istanbul ignore start*/
|
||||
b.diffCss=k.diffCss,/*istanbul ignore start*/
|
||||
b.diffJson=l.diffJson,/*istanbul ignore start*/
|
||||
b.diffArrays=m.diffArrays,/*istanbul ignore start*/
|
||||
b.structuredPatch=q.structuredPatch,/*istanbul ignore start*/
|
||||
b.createTwoFilesPatch=q.createTwoFilesPatch,/*istanbul ignore start*/
|
||||
b.createPatch=q.createPatch,/*istanbul ignore start*/
|
||||
b.applyPatch=n.applyPatch,/*istanbul ignore start*/
|
||||
b.applyPatches=n.applyPatches,/*istanbul ignore start*/
|
||||
b.parsePatch=o.parsePatch,/*istanbul ignore start*/
|
||||
b.merge=p.merge,/*istanbul ignore start*/
|
||||
b.convertChangesToDMP=r.convertChangesToDMP,/*istanbul ignore start*/
|
||||
b.convertChangesToXML=s.convertChangesToXML,/*istanbul ignore start*/
|
||||
b.canonicalize=l.canonicalize},/* 1 */
|
||||
/***/
|
||||
function(a,b){/*istanbul ignore start*/
|
||||
"use strict";function c(){}function d(a,b,c,d,e){for(var f=0,g=b.length,h=0,i=0;f<g;f++){var j=b[f];if(j.removed){
|
||||
// Reverse add and remove so removes are output first to match common convention
|
||||
// The diffing algorithm is tied to add then remove output and this is the simplest
|
||||
// route to get the desired output with minimal overhead.
|
||||
if(j.value=a.join(d.slice(i,i+j.count)),i+=j.count,f&&b[f-1].added){var k=b[f-1];b[f-1]=b[f],b[f]=k}}else{if(!j.added&&e){var l=c.slice(h,h+j.count);l=l.map(function(a,b){var c=d[i+b];return c.length>a.length?c:a}),j.value=a.join(l)}else j.value=a.join(c.slice(h,h+j.count));h+=j.count,
|
||||
// Common case
|
||||
j.added||(i+=j.count)}}
|
||||
// Special case handle for when one terminal is ignored (i.e. whitespace).
|
||||
// For this case we merge the terminal into the prior string and drop the change.
|
||||
// This is only available for string mode.
|
||||
var m=b[g-1];return g>1&&"string"==typeof m.value&&(m.added||m.removed)&&a.equals("",m.value)&&(b[g-2].value+=m.value,b.pop()),b}function e(a){return{newPos:a.newPos,components:a.components.slice(0)}}b.__esModule=!0,b["default"]=/*istanbul ignore end*/c,c.prototype={/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
diff:function(a,b){function c(a){return h?(setTimeout(function(){h(void 0,a)},0),!0):a}
|
||||
// Main worker method. checks all permutations of a given edit length for acceptance.
|
||||
function f(){for(var f=-1*l;f<=l;f+=2){var g=/*istanbul ignore start*/void 0,h=n[f-1],m=n[f+1],o=(m?m.newPos:0)-f;h&&(
|
||||
// No one else is going to attempt to use this value, clear it
|
||||
n[f-1]=void 0);var p=h&&h.newPos+1<j,q=m&&0<=o&&o<k;if(p||q){
|
||||
// If we have hit the end of both strings, then we are done
|
||||
if(
|
||||
// Select the diagonal that we want to branch from. We select the prior
|
||||
// path whose position in the new string is the farthest from the origin
|
||||
// and does not pass the bounds of the diff graph
|
||||
!p||q&&h.newPos<m.newPos?(g=e(m),i.pushComponent(g.components,void 0,!0)):(g=h,// No need to clone, we've pulled it from the list
|
||||
g.newPos++,i.pushComponent(g.components,!0,void 0)),o=i.extractCommon(g,b,a,f),g.newPos+1>=j&&o+1>=k)return c(d(i,g.components,b,a,i.useLongestToken));
|
||||
// Otherwise track this path as a potential candidate and continue.
|
||||
n[f]=g}else
|
||||
// If this path is a terminal then prune
|
||||
n[f]=void 0}l++}/*istanbul ignore start*/
|
||||
var/*istanbul ignore end*/g=arguments.length>2&&void 0!==arguments[2]?arguments[2]:{},h=g.callback;"function"==typeof g&&(h=g,g={}),this.options=g;var i=this;
|
||||
// Allow subclasses to massage the input prior to running
|
||||
a=this.castInput(a),b=this.castInput(b),a=this.removeEmpty(this.tokenize(a)),b=this.removeEmpty(this.tokenize(b));var j=b.length,k=a.length,l=1,m=j+k,n=[{newPos:-1,components:[]}],o=this.extractCommon(n[0],b,a,0);if(n[0].newPos+1>=j&&o+1>=k)
|
||||
// Identity per the equality and tokenizer
|
||||
return c([{value:this.join(b),count:b.length}]);
|
||||
// Performs the length of edit iteration. Is a bit fugly as this has to support the
|
||||
// sync and async mode which is never fun. Loops over execEditLength until a value
|
||||
// is produced.
|
||||
if(h)!function q(){setTimeout(function(){
|
||||
// This should not happen, but we want to be safe.
|
||||
/* istanbul ignore next */
|
||||
// This should not happen, but we want to be safe.
|
||||
/* istanbul ignore next */
|
||||
return l>m?h():void(f()||q())},0)}();else for(;l<=m;){var p=f();if(p)return p}},/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
pushComponent:function(a,b,c){var d=a[a.length-1];d&&d.added===b&&d.removed===c?
|
||||
// We need to clone here as the component clone operation is just
|
||||
// as shallow array clone
|
||||
a[a.length-1]={count:d.count+1,added:b,removed:c}:a.push({count:1,added:b,removed:c})},/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
extractCommon:function(a,b,c,d){for(var e=b.length,f=c.length,g=a.newPos,h=g-d,i=0;g+1<e&&h+1<f&&this.equals(b[g+1],c[h+1]);)g++,h++,i++;return i&&a.components.push({count:i}),a.newPos=g,h},/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
equals:function(a,b){return this.options.comparator?this.options.comparator(a,b):a===b||this.options.ignoreCase&&a.toLowerCase()===b.toLowerCase()},/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
removeEmpty:function(a){for(var b=[],c=0;c<a.length;c++)a[c]&&b.push(a[c]);return b},/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
castInput:function(a){return a},/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
tokenize:function(a){return a.split("")},/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
join:function(a){return a.join("")}}},/* 2 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";function d(a){return a&&a.__esModule?a:{"default":a}}function e(a,b,c){return h.diff(a,b,c)}b.__esModule=!0,b.characterDiff=void 0,b.diffChars=e;var/*istanbul ignore start*/f=c(1),g=d(f),h=/*istanbul ignore start*/b.characterDiff=new/*istanbul ignore start*/g["default"]},/* 3 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";/*istanbul ignore start*/
|
||||
function d(a){return a&&a.__esModule?a:{"default":a}}function e(a,b,c){/*istanbul ignore start*/
|
||||
return c=(0,i.generateOptions)(c,{ignoreWhitespace:!0}),l.diff(a,b,c)}function f(a,b,c){return l.diff(a,b,c)}b.__esModule=!0,b.wordDiff=void 0,b.diffWords=e,/*istanbul ignore start*/
|
||||
b.diffWordsWithSpace=f;var/*istanbul ignore start*/g=c(1),h=d(g),/*istanbul ignore start*/i=c(4),j=/^[A-Za-z\xC0-\u02C6\u02C8-\u02D7\u02DE-\u02FF\u1E00-\u1EFF]+$/,k=/\S/,l=/*istanbul ignore start*/b.wordDiff=new/*istanbul ignore start*/h["default"];l.equals=function(a,b){return this.options.ignoreCase&&(a=a.toLowerCase(),b=b.toLowerCase()),a===b||this.options.ignoreWhitespace&&!k.test(a)&&!k.test(b)},l.tokenize=function(a){
|
||||
// Join the boundary splits that we do not consider to be boundaries. This is primarily the extended Latin character set.
|
||||
for(var b=a.split(/(\s+|\b)/),c=0;c<b.length-1;c++)
|
||||
// If we have an empty string in the next field and we have only word chars before and after, merge
|
||||
!b[c+1]&&b[c+2]&&j.test(b[c])&&j.test(b[c+2])&&(b[c]+=b[c+2],b.splice(c+1,2),c--);return b}},/* 4 */
|
||||
/***/
|
||||
function(a,b){/*istanbul ignore start*/
|
||||
"use strict";function c(a,b){if("function"==typeof a)b.callback=a;else if(a)for(var c in a)/* istanbul ignore else */
|
||||
a.hasOwnProperty(c)&&(b[c]=a[c]);return b}b.__esModule=!0,b.generateOptions=c},/* 5 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";/*istanbul ignore start*/
|
||||
function d(a){return a&&a.__esModule?a:{"default":a}}function e(a,b,c){return j.diff(a,b,c)}function f(a,b,c){var d=/*istanbul ignore start*/(0,i.generateOptions)(c,{ignoreWhitespace:!0});return j.diff(a,b,d)}b.__esModule=!0,b.lineDiff=void 0,b.diffLines=e,/*istanbul ignore start*/
|
||||
b.diffTrimmedLines=f;var/*istanbul ignore start*/g=c(1),h=d(g),/*istanbul ignore start*/i=c(4),j=/*istanbul ignore start*/b.lineDiff=new/*istanbul ignore start*/h["default"];j.tokenize=function(a){var b=[],c=a.split(/(\n|\r\n)/);
|
||||
// Ignore the final empty token that occurs if the string ends with a new line
|
||||
c[c.length-1]||c.pop();
|
||||
// Merge the content and line separators into single tokens
|
||||
for(var d=0;d<c.length;d++){var e=c[d];d%2&&!this.options.newlineIsToken?b[b.length-1]+=e:(this.options.ignoreWhitespace&&(e=e.trim()),b.push(e))}return b}},/* 6 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";function d(a){return a&&a.__esModule?a:{"default":a}}function e(a,b,c){return h.diff(a,b,c)}b.__esModule=!0,b.sentenceDiff=void 0,b.diffSentences=e;var/*istanbul ignore start*/f=c(1),g=d(f),h=/*istanbul ignore start*/b.sentenceDiff=new/*istanbul ignore start*/g["default"];h.tokenize=function(a){return a.split(/(\S.+?[.!?])(?=\s+|$)/)}},/* 7 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";function d(a){return a&&a.__esModule?a:{"default":a}}function e(a,b,c){return h.diff(a,b,c)}b.__esModule=!0,b.cssDiff=void 0,b.diffCss=e;var/*istanbul ignore start*/f=c(1),g=d(f),h=/*istanbul ignore start*/b.cssDiff=new/*istanbul ignore start*/g["default"];h.tokenize=function(a){return a.split(/([{}:;,]|\s+)/)}},/* 8 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";/*istanbul ignore start*/
|
||||
function d(a){return a&&a.__esModule?a:{"default":a}}function e(a,b,c){return l.diff(a,b,c)}
|
||||
// This function handles the presence of circular references by bailing out when encountering an
|
||||
// object that is already on the "stack" of items being processed. Accepts an optional replacer
|
||||
function f(a,b,c,d,e){b=b||[],c=c||[],d&&(a=d(e,a));var h=/*istanbul ignore start*/void 0;for(h=0;h<b.length;h+=1)if(b[h]===a)return c[h];var i=/*istanbul ignore start*/void 0;if("[object Array]"===k.call(a)){for(b.push(a),i=new Array(a.length),c.push(i),h=0;h<a.length;h+=1)i[h]=f(a[h],b,c,d,e);return b.pop(),c.pop(),i}if(a&&a.toJSON&&(a=a.toJSON()),/*istanbul ignore start*/"object"===("undefined"==typeof/*istanbul ignore end*/a?"undefined":g(a))&&null!==a){b.push(a),i={},c.push(i);var j=[],l=/*istanbul ignore start*/void 0;for(l in a)/* istanbul ignore else */
|
||||
a.hasOwnProperty(l)&&j.push(l);for(j.sort(),h=0;h<j.length;h+=1)l=j[h],i[l]=f(a[l],b,c,d,l);b.pop(),c.pop()}else i=a;return i}b.__esModule=!0,b.jsonDiff=void 0;var g="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(a){return typeof a}:function(a){return a&&"function"==typeof Symbol&&a.constructor===Symbol&&a!==Symbol.prototype?"symbol":typeof a};b.diffJson=e,/*istanbul ignore start*/
|
||||
b.canonicalize=f;var/*istanbul ignore start*/h=c(1),i=d(h),/*istanbul ignore start*/j=c(5),k=Object.prototype.toString,l=/*istanbul ignore start*/b.jsonDiff=new/*istanbul ignore start*/i["default"];
|
||||
// Discriminate between two lines of pretty-printed, serialized JSON where one of them has a
|
||||
// dangling comma and the other doesn't. Turns out including the dangling comma yields the nicest output:
|
||||
l.useLongestToken=!0,l.tokenize=/*istanbul ignore start*/j.lineDiff.tokenize,l.castInput=function(a){/*istanbul ignore start*/
|
||||
var b=/*istanbul ignore end*/this.options,c=b.undefinedReplacement,d=b.stringifyReplacer,e=void 0===d?function(a,b){/*istanbul ignore end*/
|
||||
return"undefined"==typeof b?c:b}:d;return"string"==typeof a?a:JSON.stringify(f(a,null,null,e),e," ")},l.equals=function(a,b){/*istanbul ignore start*/
|
||||
return i["default"].prototype.equals.call(l,a.replace(/,([\r\n])/g,"$1"),b.replace(/,([\r\n])/g,"$1"))}},/* 9 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";function d(a){return a&&a.__esModule?a:{"default":a}}function e(a,b,c){return h.diff(a,b,c)}b.__esModule=!0,b.arrayDiff=void 0,b.diffArrays=e;var/*istanbul ignore start*/f=c(1),g=d(f),h=/*istanbul ignore start*/b.arrayDiff=new/*istanbul ignore start*/g["default"];h.tokenize=function(a){return a.slice()},h.join=h.removeEmpty=function(a){return a}},/* 10 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";function d(a){return a&&a.__esModule?a:{"default":a}}/*istanbul ignore end*/
|
||||
function e(a,b){/**
|
||||
* Checks if the hunk exactly fits on the provided location
|
||||
*/
|
||||
function c(a,b){for(var c=0;c<a.lines.length;c++){var d=a.lines[c],f=d.length>0?d[0]:" ",g=d.length>0?d.substr(1):d;if(" "===f||"-"===f){
|
||||
// Context sanity check
|
||||
if(!j(b+1,e[b],f,g)&&(k++,k>l))return!1;b++}}return!0}/*istanbul ignore start*/
|
||||
var/*istanbul ignore end*/d=arguments.length>2&&void 0!==arguments[2]?arguments[2]:{};if("string"==typeof b&&(b=/*istanbul ignore start*/(0,g.parsePatch)(b)),Array.isArray(b)){if(b.length>1)throw new Error("applyPatch only works with a single input.");b=b[0]}
|
||||
// Search best fit offsets for each hunk based on the previous ones
|
||||
for(var e=a.split(/\r\n|[\n\v\f\r\x85]/),f=a.match(/\r\n|[\n\v\f\r\x85]/g)||[],h=b.hunks,j=d.compareLine||function(a,b,c,d){/*istanbul ignore end*/
|
||||
return b===d},k=0,l=d.fuzzFactor||0,m=0,n=0,o=/*istanbul ignore start*/void 0,p=/*istanbul ignore start*/void 0,q=0;q<h.length;q++){for(var r=h[q],s=e.length-r.oldLines,t=0,u=n+r.oldStart-1,v=/*istanbul ignore start*/(0,i["default"])(u,m,s);void 0!==t;t=v())if(c(r,u+t)){r.offset=n+=t;break}if(void 0===t)return!1;
|
||||
// Set lower text limit to end of the current hunk, so next ones don't try
|
||||
// to fit over already patched text
|
||||
m=r.offset+r.oldStart+r.oldLines}for(var w=0,x=0;x<h.length;x++){var y=h[x],z=y.oldStart+y.offset+w-1;w+=y.newLines-y.oldLines,z<0&&(
|
||||
// Creating a new file
|
||||
z=0);for(var A=0;A<y.lines.length;A++){var B=y.lines[A],C=B.length>0?B[0]:" ",D=B.length>0?B.substr(1):B,E=y.linedelimiters[A];if(" "===C)z++;else if("-"===C)e.splice(z,1),f.splice(z,1);else if("+"===C)e.splice(z,0,D),f.splice(z,0,E),z++;else if("\\"===C){var F=y.lines[A-1]?y.lines[A-1][0]:null;"+"===F?o=!0:"-"===F&&(p=!0)}}}
|
||||
// Handle EOFNL insertion/removal
|
||||
if(o)for(;!e[e.length-1];)e.pop(),f.pop();else p&&(e.push(""),f.push("\n"));for(var G=0;G<e.length-1;G++)e[G]=e[G]+f[G];return e.join("")}
|
||||
// Wrapper that supports multiple file patches via callbacks.
|
||||
function f(a,b){function c(){var f=a[d++];return f?void b.loadFile(f,function(a,d){if(a)return b.complete(a);var g=e(d,f,b);b.patched(f,g,function(a){return a?b.complete(a):void c()})}):b.complete()}"string"==typeof a&&(a=/*istanbul ignore start*/(0,g.parsePatch)(a));var d=0;c()}b.__esModule=!0,b.applyPatch=e,/*istanbul ignore start*/
|
||||
b.applyPatches=f;var/*istanbul ignore start*/g=c(11),/*istanbul ignore start*/h=c(12),i=d(h)},/* 11 */
|
||||
/***/
|
||||
function(a,b){/*istanbul ignore start*/
|
||||
"use strict";function c(a){function b(){var a={};
|
||||
// Parse diff metadata
|
||||
for(h.push(a);i<f.length;){var b=f[i];
|
||||
// File header found, end parsing diff metadata
|
||||
if(/^(\-\-\-|\+\+\+|@@)\s/.test(b))break;
|
||||
// Diff index
|
||||
var g=/^(?:Index:|diff(?: -r \w+)+)\s+(.+?)\s*$/.exec(b);g&&(a.index=g[1]),i++}for(
|
||||
// Parse file headers if they are defined. Unified diff requires them, but
|
||||
// there's no technical issues to have an isolated hunk without file header
|
||||
c(a),c(a),
|
||||
// Parse hunks
|
||||
a.hunks=[];i<f.length;){var j=f[i];if(/^(Index:|diff|\-\-\-|\+\+\+)\s/.test(j))break;if(/^@@/.test(j))a.hunks.push(d());else{if(j&&e.strict)
|
||||
// Ignore unexpected content unless in strict mode
|
||||
throw new Error("Unknown line "+(i+1)+" "+JSON.stringify(j));i++}}}
|
||||
// Parses the --- and +++ headers, if none are found, no lines
|
||||
// are consumed.
|
||||
function c(a){var b=/^(---|\+\+\+)\s+(.*)$/.exec(f[i]);if(b){var c="---"===b[1]?"old":"new",d=b[2].split("\t",2),e=d[0].replace(/\\\\/g,"\\");/^".*"$/.test(e)&&(e=e.substr(1,e.length-2)),a[c+"FileName"]=e,a[c+"Header"]=(d[1]||"").trim(),i++}}
|
||||
// Parses a hunk
|
||||
// This assumes that we are at the start of a hunk.
|
||||
function d(){for(var a=i,b=f[i++],c=b.split(/@@ -(\d+)(?:,(\d+))? \+(\d+)(?:,(\d+))? @@/),d={oldStart:+c[1],oldLines:+c[2]||1,newStart:+c[3],newLines:+c[4]||1,lines:[],linedelimiters:[]},h=0,j=0;i<f.length&&!(0===f[i].indexOf("--- ")&&i+2<f.length&&0===f[i+1].indexOf("+++ ")&&0===f[i+2].indexOf("@@"));i++){var k=0==f[i].length&&i!=f.length-1?" ":f[i][0];if("+"!==k&&"-"!==k&&" "!==k&&"\\"!==k)break;d.lines.push(f[i]),d.linedelimiters.push(g[i]||"\n"),"+"===k?h++:"-"===k?j++:" "===k&&(h++,j++)}
|
||||
// Perform optional sanity checking
|
||||
if(
|
||||
// Handle the empty block count case
|
||||
h||1!==d.newLines||(d.newLines=0),j||1!==d.oldLines||(d.oldLines=0),e.strict){if(h!==d.newLines)throw new Error("Added line count did not match for hunk at line "+(a+1));if(j!==d.oldLines)throw new Error("Removed line count did not match for hunk at line "+(a+1))}return d}for(/*istanbul ignore start*/
|
||||
var/*istanbul ignore end*/e=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},f=a.split(/\r\n|[\n\v\f\r\x85]/),g=a.match(/\r\n|[\n\v\f\r\x85]/g)||[],h=[],i=0;i<f.length;)b();return h}b.__esModule=!0,b.parsePatch=c},/* 12 */
|
||||
/***/
|
||||
function(a,b){/*istanbul ignore start*/
|
||||
"use strict";b.__esModule=!0,b["default"]=/*istanbul ignore end*/function(a,b,c){var d=!0,e=!1,f=!1,g=1;return function h(){if(d&&!f){
|
||||
// Check if trying to fit beyond text length, and if not, check it fits
|
||||
// after offset location (or desired location on first iteration)
|
||||
if(e?g++:d=!1,a+g<=c)return g;f=!0}if(!e)
|
||||
// Check if trying to fit before text beginning, and if not, check it fits
|
||||
// before offset location
|
||||
// Check if trying to fit before text beginning, and if not, check it fits
|
||||
// before offset location
|
||||
return f||(d=!0),b<=a-g?-g++:(e=!0,h())}}},/* 13 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";/*istanbul ignore start*/
|
||||
function d(a){if(Array.isArray(a)){for(var b=0,c=Array(a.length);b<a.length;b++)c[b]=a[b];return c}return Array.from(a)}/*istanbul ignore end*/
|
||||
function e(a){/*istanbul ignore start*/
|
||||
var b=/*istanbul ignore end*/v(a.lines),c=b.oldLines,d=b.newLines;void 0!==c?a.oldLines=c:delete a.oldLines,void 0!==d?a.newLines=d:delete a.newLines}function f(a,b,c){a=g(a,c),b=g(b,c);var d={};
|
||||
// For index we just let it pass through as it doesn't have any necessary meaning.
|
||||
// Leaving sanity checks on this to the API consumer that may know more about the
|
||||
// meaning in their own context.
|
||||
(a.index||b.index)&&(d.index=a.index||b.index),(a.newFileName||b.newFileName)&&(h(a)?h(b)?(
|
||||
// Both changed... figure it out
|
||||
d.oldFileName=i(d,a.oldFileName,b.oldFileName),d.newFileName=i(d,a.newFileName,b.newFileName),d.oldHeader=i(d,a.oldHeader,b.oldHeader),d.newHeader=i(d,a.newHeader,b.newHeader)):(
|
||||
// No header or no change in theirs, use ours
|
||||
d.oldFileName=a.oldFileName,d.newFileName=a.newFileName,d.oldHeader=a.oldHeader,d.newHeader=a.newHeader):(
|
||||
// No header or no change in ours, use theirs (and ours if theirs does not exist)
|
||||
d.oldFileName=b.oldFileName||a.oldFileName,d.newFileName=b.newFileName||a.newFileName,d.oldHeader=b.oldHeader||a.oldHeader,d.newHeader=b.newHeader||a.newHeader)),d.hunks=[];for(var e=0,f=0,m=0,n=0;e<a.hunks.length||f<b.hunks.length;){var o=a.hunks[e]||{oldStart:1/0},p=b.hunks[f]||{oldStart:1/0};if(j(o,p))
|
||||
// This patch does not overlap with any of the others, yay.
|
||||
d.hunks.push(k(o,m)),e++,n+=o.newLines-o.oldLines;else if(j(p,o))
|
||||
// This patch does not overlap with any of the others, yay.
|
||||
d.hunks.push(k(p,n)),f++,m+=p.newLines-p.oldLines;else{
|
||||
// Overlap, merge as best we can
|
||||
var q={oldStart:Math.min(o.oldStart,p.oldStart),oldLines:0,newStart:Math.min(o.newStart+m,p.oldStart+n),newLines:0,lines:[]};l(q,o.oldStart,o.lines,p.oldStart,p.lines),f++,e++,d.hunks.push(q)}}return d}function g(a,b){if("string"==typeof a){if(/^@@/m.test(a)||/^Index:/m.test(a))/*istanbul ignore start*/
|
||||
return(0,x.parsePatch)(a)[0];if(!b)throw new Error("Must provide a base reference or pass in a patch");/*istanbul ignore start*/
|
||||
return(0,w.structuredPatch)(void 0,void 0,b,a)}return a}function h(a){return a.newFileName&&a.newFileName!==a.oldFileName}function i(a,b,c){return b===c?b:(a.conflict=!0,{mine:b,theirs:c})}function j(a,b){return a.oldStart<b.oldStart&&a.oldStart+a.oldLines<b.oldStart}function k(a,b){return{oldStart:a.oldStart,oldLines:a.oldLines,newStart:a.newStart+b,newLines:a.newLines,lines:a.lines}}function l(a,b,c,f,g){
|
||||
// This will generally result in a conflicted hunk, but there are cases where the context
|
||||
// is the only overlap where we can successfully merge the content here.
|
||||
var h={offset:b,lines:c,index:0},i={offset:f,lines:g,index:0};
|
||||
// Now in the overlap content. Scan through and select the best changes from each.
|
||||
for(
|
||||
// Handle any leading content
|
||||
p(a,h,i),p(a,i,h);h.index<h.lines.length&&i.index<i.lines.length;){var j=h.lines[h.index],k=i.lines[i.index];if("-"!==j[0]&&"+"!==j[0]||"-"!==k[0]&&"+"!==k[0])if("+"===j[0]&&" "===k[0]){/*istanbul ignore start*/
|
||||
var l;/*istanbul ignore end*/
|
||||
// Mine inserted
|
||||
/*istanbul ignore start*/
|
||||
(l=/*istanbul ignore end*/a.lines).push.apply(/*istanbul ignore start*/l,/*istanbul ignore start*/d(/*istanbul ignore end*/r(h)))}else if("+"===k[0]&&" "===j[0]){/*istanbul ignore start*/
|
||||
var s;/*istanbul ignore end*/
|
||||
// Theirs inserted
|
||||
/*istanbul ignore start*/
|
||||
(s=/*istanbul ignore end*/a.lines).push.apply(/*istanbul ignore start*/s,/*istanbul ignore start*/d(/*istanbul ignore end*/r(i)))}else"-"===j[0]&&" "===k[0]?
|
||||
// Mine removed or edited
|
||||
n(a,h,i):"-"===k[0]&&" "===j[0]?
|
||||
// Their removed or edited
|
||||
n(a,i,h,!0):j===k?(
|
||||
// Context identity
|
||||
a.lines.push(j),h.index++,i.index++):
|
||||
// Context mismatch
|
||||
o(a,r(h),r(i));else
|
||||
// Both modified ...
|
||||
m(a,h,i)}
|
||||
// Now push anything that may be remaining
|
||||
q(a,h),q(a,i),e(a)}function m(a,b,c){var e=r(b),f=r(c);if(t(e)&&t(f)){
|
||||
// Special case for remove changes that are supersets of one another
|
||||
if(/*istanbul ignore start*/(0,y.arrayStartsWith)(e,f)&&u(c,e,e.length-f.length)){/*istanbul ignore start*/
|
||||
var g;/*istanbul ignore end*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
return void(g=a.lines).push.apply(g,d(e))}if(/*istanbul ignore start*/(0,y.arrayStartsWith)(f,e)&&u(b,f,f.length-e.length)){/*istanbul ignore start*/
|
||||
var h;/*istanbul ignore end*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
return void(h=a.lines).push.apply(h,d(f))}}else if(/*istanbul ignore start*/(0,y.arrayEqual)(e,f)){/*istanbul ignore start*/
|
||||
var i;/*istanbul ignore end*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore start*/
|
||||
/*istanbul ignore end*/
|
||||
return void(i=a.lines).push.apply(i,d(e))}o(a,e,f)}function n(a,b,c,e){var f=r(b),g=s(c,f);if(g.merged){/*istanbul ignore start*/
|
||||
var h;/*istanbul ignore end*/
|
||||
/*istanbul ignore start*/
|
||||
(h=/*istanbul ignore end*/a.lines).push.apply(/*istanbul ignore start*/h,/*istanbul ignore start*/d(/*istanbul ignore end*/g.merged))}else o(a,e?g:f,e?f:g)}function o(a,b,c){a.conflict=!0,a.lines.push({conflict:!0,mine:b,theirs:c})}function p(a,b,c){for(;b.offset<c.offset&&b.index<b.lines.length;){var d=b.lines[b.index++];a.lines.push(d),b.offset++}}function q(a,b){for(;b.index<b.lines.length;){var c=b.lines[b.index++];a.lines.push(c)}}function r(a){for(var b=[],c=a.lines[a.index][0];a.index<a.lines.length;){var d=a.lines[a.index];if(
|
||||
// Group additions that are immediately after subtractions and treat them as one "atomic" modify change.
|
||||
"-"===c&&"+"===d[0]&&(c="+"),c!==d[0])break;b.push(d),a.index++}return b}function s(a,b){for(var c=[],d=[],e=0,f=!1,g=!1;e<b.length&&a.index<a.lines.length;){var h=a.lines[a.index],i=b[e];
|
||||
// Once we've hit our add, then we are done
|
||||
if("+"===i[0])break;
|
||||
// Consume any additions in the other block as a conflict to attempt
|
||||
// to pull in the remaining context after this
|
||||
if(f=f||" "!==h[0],d.push(i),e++,"+"===h[0])for(g=!0;"+"===h[0];)c.push(h),h=a.lines[++a.index];i.substr(1)===h.substr(1)?(c.push(h),a.index++):g=!0}if("+"===(b[e]||"")[0]&&f&&(g=!0),g)return c;for(;e<b.length;)d.push(b[e++]);return{merged:d,changes:c}}function t(a){return a.reduce(function(a,b){return a&&"-"===b[0]},!0)}function u(a,b,c){for(var d=0;d<c;d++){var e=b[b.length-c+d].substr(1);if(a.lines[a.index+d]!==" "+e)return!1}return a.index+=c,!0}function v(a){var b=0,c=0;return a.forEach(function(a){if("string"!=typeof a){var d=v(a.mine),e=v(a.theirs);void 0!==b&&(d.oldLines===e.oldLines?b+=d.oldLines:b=void 0),void 0!==c&&(d.newLines===e.newLines?c+=d.newLines:c=void 0)}else void 0===c||"+"!==a[0]&&" "!==a[0]||c++,void 0===b||"-"!==a[0]&&" "!==a[0]||b++}),{oldLines:b,newLines:c}}b.__esModule=!0,b.calcLineCount=e,/*istanbul ignore start*/
|
||||
b.merge=f;var/*istanbul ignore start*/w=c(14),/*istanbul ignore start*/x=c(11),/*istanbul ignore start*/y=c(15)},/* 14 */
|
||||
/***/
|
||||
function(a,b,c){/*istanbul ignore start*/
|
||||
"use strict";/*istanbul ignore start*/
|
||||
function d(a){if(Array.isArray(a)){for(var b=0,c=Array(a.length);b<a.length;b++)c[b]=a[b];return c}return Array.from(a)}/*istanbul ignore end*/
|
||||
function e(a,b,c,e,f,g,i){// Append an empty value to make cleanup easier
|
||||
function j(a){return a.map(function(a){return" "+a})}i||(i={}),"undefined"==typeof i.context&&(i.context=4);var k=/*istanbul ignore start*/(0,h.diffLines)(c,e,i);k.push({value:"",lines:[]});for(var l=[],m=0,n=0,o=[],p=1,q=1,r=function(/*istanbul ignore end*/a){var b=k[a],f=b.lines||b.value.replace(/\n$/,"").split("\n");if(b.lines=f,b.added||b.removed){/*istanbul ignore start*/
|
||||
var g;/*istanbul ignore end*/
|
||||
// If we have previous context, start with that
|
||||
if(!m){var h=k[a-1];m=p,n=q,h&&(o=i.context>0?j(h.lines.slice(-i.context)):[],m-=o.length,n-=o.length)}
|
||||
// Output our changes
|
||||
/*istanbul ignore start*/
|
||||
(g=/*istanbul ignore end*/o).push.apply(/*istanbul ignore start*/g,/*istanbul ignore start*/d(/*istanbul ignore end*/f.map(function(a){return(b.added?"+":"-")+a}))),
|
||||
// Track the updated file position
|
||||
b.added?q+=f.length:p+=f.length}else{
|
||||
// Identical context lines. Track line changes
|
||||
if(m)
|
||||
// Close out any changes that have been output (or join overlapping)
|
||||
if(f.length<=2*i.context&&a<k.length-2){/*istanbul ignore start*/
|
||||
var r;/*istanbul ignore end*/
|
||||
// Overlapping
|
||||
/*istanbul ignore start*/
|
||||
(r=/*istanbul ignore end*/o).push.apply(/*istanbul ignore start*/r,/*istanbul ignore start*/d(/*istanbul ignore end*/j(f)))}else{/*istanbul ignore start*/
|
||||
var s,t=Math.min(f.length,i.context);/*istanbul ignore start*/
|
||||
(s=/*istanbul ignore end*/o).push.apply(/*istanbul ignore start*/s,/*istanbul ignore start*/d(/*istanbul ignore end*/j(f.slice(0,t))));var u={oldStart:m,oldLines:p-m+t,newStart:n,newLines:q-n+t,lines:o};if(a>=k.length-2&&f.length<=i.context){
|
||||
// EOF is inside this hunk
|
||||
var v=/\n$/.test(c),w=/\n$/.test(e);0!=f.length||v?v&&w||o.push("\\ No newline at end of file"):
|
||||
// special case: old has no eol and no trailing context; no-nl can end up before adds
|
||||
o.splice(u.oldLines,0,"\\ No newline at end of file")}l.push(u),m=0,n=0,o=[]}p+=f.length,q+=f.length}},s=0;s<k.length;s++)/*istanbul ignore start*/
|
||||
r(/*istanbul ignore end*/s);return{oldFileName:a,newFileName:b,oldHeader:f,newHeader:g,hunks:l}}function f(a,b,c,d,f,g,h){var i=e(a,b,c,d,f,g,h),j=[];a==b&&j.push("Index: "+a),j.push("==================================================================="),j.push("--- "+i.oldFileName+("undefined"==typeof i.oldHeader?"":"\t"+i.oldHeader)),j.push("+++ "+i.newFileName+("undefined"==typeof i.newHeader?"":"\t"+i.newHeader));for(var k=0;k<i.hunks.length;k++){var l=i.hunks[k];j.push("@@ -"+l.oldStart+","+l.oldLines+" +"+l.newStart+","+l.newLines+" @@"),j.push.apply(j,l.lines)}return j.join("\n")+"\n"}function g(a,b,c,d,e,g){return f(a,a,b,c,d,e,g)}b.__esModule=!0,b.structuredPatch=e,/*istanbul ignore start*/
|
||||
b.createTwoFilesPatch=f,/*istanbul ignore start*/
|
||||
b.createPatch=g;var/*istanbul ignore start*/h=c(5)},/* 15 */
|
||||
/***/
|
||||
function(a,b){/*istanbul ignore start*/
|
||||
"use strict";function c(a,b){return a.length===b.length&&d(a,b)}function d(a,b){if(b.length>a.length)return!1;for(var c=0;c<b.length;c++)if(b[c]!==a[c])return!1;return!0}b.__esModule=!0,b.arrayEqual=c,/*istanbul ignore start*/
|
||||
b.arrayStartsWith=d},/* 16 */
|
||||
/***/
|
||||
function(a,b){/*istanbul ignore start*/
|
||||
"use strict";
|
||||
// See: http://code.google.com/p/google-diff-match-patch/wiki/API
|
||||
function c(a){for(var b=[],c=/*istanbul ignore start*/void 0,d=/*istanbul ignore start*/void 0,e=0;e<a.length;e++)c=a[e],d=c.added?1:c.removed?-1:0,b.push([d,c.value]);return b}b.__esModule=!0,b.convertChangesToDMP=c},/* 17 */
|
||||
/***/
|
||||
function(a,b){/*istanbul ignore start*/
|
||||
"use strict";function c(a){for(var b=[],c=0;c<a.length;c++){var e=a[c];e.added?b.push("<ins>"):e.removed&&b.push("<del>"),b.push(d(e.value)),e.added?b.push("</ins>"):e.removed&&b.push("</del>")}return b.join("")}function d(a){var b=a;return b=b.replace(/&/g,"&"),b=b.replace(/</g,"<"),b=b.replace(/>/g,">"),b=b.replace(/"/g,""")}b.__esModule=!0,b.convertChangesToXML=c}])});
|
|
@ -0,0 +1,24 @@
|
|||
/*istanbul ignore start*/"use strict";
|
||||
|
||||
exports.__esModule = true;
|
||||
exports. /*istanbul ignore end*/convertChangesToDMP = convertChangesToDMP;
|
||||
// See: http://code.google.com/p/google-diff-match-patch/wiki/API
|
||||
function convertChangesToDMP(changes) {
|
||||
var ret = [],
|
||||
change = /*istanbul ignore start*/void 0 /*istanbul ignore end*/,
|
||||
operation = /*istanbul ignore start*/void 0 /*istanbul ignore end*/;
|
||||
for (var i = 0; i < changes.length; i++) {
|
||||
change = changes[i];
|
||||
if (change.added) {
|
||||
operation = 1;
|
||||
} else if (change.removed) {
|
||||
operation = -1;
|
||||
} else {
|
||||
operation = 0;
|
||||
}
|
||||
|
||||
ret.push([operation, change.value]);
|
||||
}
|
||||
return ret;
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy9jb252ZXJ0L2RtcC5qcyJdLCJuYW1lcyI6WyJjb252ZXJ0Q2hhbmdlc1RvRE1QIiwiY2hhbmdlcyIsInJldCIsImNoYW5nZSIsIm9wZXJhdGlvbiIsImkiLCJsZW5ndGgiLCJhZGRlZCIsInJlbW92ZWQiLCJwdXNoIiwidmFsdWUiXSwibWFwcGluZ3MiOiI7OztnQ0FDZ0JBLG1CLEdBQUFBLG1CO0FBRGhCO0FBQ08sU0FBU0EsbUJBQVQsQ0FBNkJDLE9BQTdCLEVBQXNDO0FBQzNDLE1BQUlDLE1BQU0sRUFBVjtBQUFBLE1BQ0lDLHdDQURKO0FBQUEsTUFFSUMsMkNBRko7QUFHQSxPQUFLLElBQUlDLElBQUksQ0FBYixFQUFnQkEsSUFBSUosUUFBUUssTUFBNUIsRUFBb0NELEdBQXBDLEVBQXlDO0FBQ3ZDRixhQUFTRixRQUFRSSxDQUFSLENBQVQ7QUFDQSxRQUFJRixPQUFPSSxLQUFYLEVBQWtCO0FBQ2hCSCxrQkFBWSxDQUFaO0FBQ0QsS0FGRCxNQUVPLElBQUlELE9BQU9LLE9BQVgsRUFBb0I7QUFDekJKLGtCQUFZLENBQUMsQ0FBYjtBQUNELEtBRk0sTUFFQTtBQUNMQSxrQkFBWSxDQUFaO0FBQ0Q7O0FBRURGLFFBQUlPLElBQUosQ0FBUyxDQUFDTCxTQUFELEVBQVlELE9BQU9PLEtBQW5CLENBQVQ7QUFDRDtBQUNELFNBQU9SLEdBQVA7QUFDRCIsImZpbGUiOiJkbXAuanMiLCJzb3VyY2VzQ29udGVudCI6WyIvLyBTZWU6IGh0dHA6Ly9jb2RlLmdvb2dsZS5jb20vcC9nb29nbGUtZGlmZi1tYXRjaC1wYXRjaC93aWtpL0FQSVxuZXhwb3J0IGZ1bmN0aW9uIGNvbnZlcnRDaGFuZ2VzVG9ETVAoY2hhbmdlcykge1xuICBsZXQgcmV0ID0gW10sXG4gICAgICBjaGFuZ2UsXG4gICAgICBvcGVyYXRpb247XG4gIGZvciAobGV0IGkgPSAwOyBpIDwgY2hhbmdlcy5sZW5ndGg7IGkrKykge1xuICAgIGNoYW5nZSA9IGNoYW5nZXNbaV07XG4gICAgaWYgKGNoYW5nZS5hZGRlZCkge1xuICAgICAgb3BlcmF0aW9uID0gMTtcbiAgICB9IGVsc2UgaWYgKGNoYW5nZS5yZW1vdmVkKSB7XG4gICAgICBvcGVyYXRpb24gPSAtMTtcbiAgICB9IGVsc2Uge1xuICAgICAgb3BlcmF0aW9uID0gMDtcbiAgICB9XG5cbiAgICByZXQucHVzaChbb3BlcmF0aW9uLCBjaGFuZ2UudmFsdWVdKTtcbiAgfVxuICByZXR1cm4gcmV0O1xufVxuIl19
|
|
@ -0,0 +1,35 @@
|
|||
/*istanbul ignore start*/'use strict';
|
||||
|
||||
exports.__esModule = true;
|
||||
exports. /*istanbul ignore end*/convertChangesToXML = convertChangesToXML;
|
||||
function convertChangesToXML(changes) {
|
||||
var ret = [];
|
||||
for (var i = 0; i < changes.length; i++) {
|
||||
var change = changes[i];
|
||||
if (change.added) {
|
||||
ret.push('<ins>');
|
||||
} else if (change.removed) {
|
||||
ret.push('<del>');
|
||||
}
|
||||
|
||||
ret.push(escapeHTML(change.value));
|
||||
|
||||
if (change.added) {
|
||||
ret.push('</ins>');
|
||||
} else if (change.removed) {
|
||||
ret.push('</del>');
|
||||
}
|
||||
}
|
||||
return ret.join('');
|
||||
}
|
||||
|
||||
function escapeHTML(s) {
|
||||
var n = s;
|
||||
n = n.replace(/&/g, '&');
|
||||
n = n.replace(/</g, '<');
|
||||
n = n.replace(/>/g, '>');
|
||||
n = n.replace(/"/g, '"');
|
||||
|
||||
return n;
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy9jb252ZXJ0L3htbC5qcyJdLCJuYW1lcyI6WyJjb252ZXJ0Q2hhbmdlc1RvWE1MIiwiY2hhbmdlcyIsInJldCIsImkiLCJsZW5ndGgiLCJjaGFuZ2UiLCJhZGRlZCIsInB1c2giLCJyZW1vdmVkIiwiZXNjYXBlSFRNTCIsInZhbHVlIiwiam9pbiIsInMiLCJuIiwicmVwbGFjZSJdLCJtYXBwaW5ncyI6Ijs7O2dDQUFnQkEsbUIsR0FBQUEsbUI7QUFBVCxTQUFTQSxtQkFBVCxDQUE2QkMsT0FBN0IsRUFBc0M7QUFDM0MsTUFBSUMsTUFBTSxFQUFWO0FBQ0EsT0FBSyxJQUFJQyxJQUFJLENBQWIsRUFBZ0JBLElBQUlGLFFBQVFHLE1BQTVCLEVBQW9DRCxHQUFwQyxFQUF5QztBQUN2QyxRQUFJRSxTQUFTSixRQUFRRSxDQUFSLENBQWI7QUFDQSxRQUFJRSxPQUFPQyxLQUFYLEVBQWtCO0FBQ2hCSixVQUFJSyxJQUFKLENBQVMsT0FBVDtBQUNELEtBRkQsTUFFTyxJQUFJRixPQUFPRyxPQUFYLEVBQW9CO0FBQ3pCTixVQUFJSyxJQUFKLENBQVMsT0FBVDtBQUNEOztBQUVETCxRQUFJSyxJQUFKLENBQVNFLFdBQVdKLE9BQU9LLEtBQWxCLENBQVQ7O0FBRUEsUUFBSUwsT0FBT0MsS0FBWCxFQUFrQjtBQUNoQkosVUFBSUssSUFBSixDQUFTLFFBQVQ7QUFDRCxLQUZELE1BRU8sSUFBSUYsT0FBT0csT0FBWCxFQUFvQjtBQUN6Qk4sVUFBSUssSUFBSixDQUFTLFFBQVQ7QUFDRDtBQUNGO0FBQ0QsU0FBT0wsSUFBSVMsSUFBSixDQUFTLEVBQVQsQ0FBUDtBQUNEOztBQUVELFNBQVNGLFVBQVQsQ0FBb0JHLENBQXBCLEVBQXVCO0FBQ3JCLE1BQUlDLElBQUlELENBQVI7QUFDQUMsTUFBSUEsRUFBRUMsT0FBRixDQUFVLElBQVYsRUFBZ0IsT0FBaEIsQ0FBSjtBQUNBRCxNQUFJQSxFQUFFQyxPQUFGLENBQVUsSUFBVixFQUFnQixNQUFoQixDQUFKO0FBQ0FELE1BQUlBLEVBQUVDLE9BQUYsQ0FBVSxJQUFWLEVBQWdCLE1BQWhCLENBQUo7QUFDQUQsTUFBSUEsRUFBRUMsT0FBRixDQUFVLElBQVYsRUFBZ0IsUUFBaEIsQ0FBSjs7QUFFQSxTQUFPRCxDQUFQO0FBQ0QiLCJmaWxlIjoieG1sLmpzIiwic291cmNlc0NvbnRlbnQiOlsiZXhwb3J0IGZ1bmN0aW9uIGNvbnZlcnRDaGFuZ2VzVG9YTUwoY2hhbmdlcykge1xuICBsZXQgcmV0ID0gW107XG4gIGZvciAobGV0IGkgPSAwOyBpIDwgY2hhbmdlcy5sZW5ndGg7IGkrKykge1xuICAgIGxldCBjaGFuZ2UgPSBjaGFuZ2VzW2ldO1xuICAgIGlmIChjaGFuZ2UuYWRkZWQpIHtcbiAgICAgIHJldC5wdXNoKCc8aW5zPicpO1xuICAgIH0gZWxzZSBpZiAoY2hhbmdlLnJlbW92ZWQpIHtcbiAgICAgIHJldC5wdXNoKCc8ZGVsPicpO1xuICAgIH1cblxuICAgIHJldC5wdXNoKGVzY2FwZUhUTUwoY2hhbmdlLnZhbHVlKSk7XG5cbiAgICBpZiAoY2hhbmdlLmFkZGVkKSB7XG4gICAgICByZXQucHVzaCgnPC9pbnM+Jyk7XG4gICAgfSBlbHNlIGlmIChjaGFuZ2UucmVtb3ZlZCkge1xuICAgICAgcmV0LnB1c2goJzwvZGVsPicpO1xuICAgIH1cbiAgfVxuICByZXR1cm4gcmV0LmpvaW4oJycpO1xufVxuXG5mdW5jdGlvbiBlc2NhcGVIVE1MKHMpIHtcbiAgbGV0IG4gPSBzO1xuICBuID0gbi5yZXBsYWNlKC8mL2csICcmYW1wOycpO1xuICBuID0gbi5yZXBsYWNlKC88L2csICcmbHQ7Jyk7XG4gIG4gPSBuLnJlcGxhY2UoLz4vZywgJyZndDsnKTtcbiAgbiA9IG4ucmVwbGFjZSgvXCIvZywgJyZxdW90OycpO1xuXG4gIHJldHVybiBuO1xufVxuIl19
|
|
@ -0,0 +1,24 @@
|
|||
/*istanbul ignore start*/'use strict';
|
||||
|
||||
exports.__esModule = true;
|
||||
exports.arrayDiff = undefined;
|
||||
exports. /*istanbul ignore end*/diffArrays = diffArrays;
|
||||
|
||||
var /*istanbul ignore start*/_base = require('./base') /*istanbul ignore end*/;
|
||||
|
||||
/*istanbul ignore start*/var _base2 = _interopRequireDefault(_base);
|
||||
|
||||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; }
|
||||
|
||||
/*istanbul ignore end*/var arrayDiff = /*istanbul ignore start*/exports. /*istanbul ignore end*/arrayDiff = new /*istanbul ignore start*/_base2['default'] /*istanbul ignore end*/();
|
||||
arrayDiff.tokenize = function (value) {
|
||||
return value.slice();
|
||||
};
|
||||
arrayDiff.join = arrayDiff.removeEmpty = function (value) {
|
||||
return value;
|
||||
};
|
||||
|
||||
function diffArrays(oldArr, newArr, callback) {
|
||||
return arrayDiff.diff(oldArr, newArr, callback);
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy9kaWZmL2FycmF5LmpzIl0sIm5hbWVzIjpbImRpZmZBcnJheXMiLCJhcnJheURpZmYiLCJ0b2tlbml6ZSIsInZhbHVlIiwic2xpY2UiLCJqb2luIiwicmVtb3ZlRW1wdHkiLCJvbGRBcnIiLCJuZXdBcnIiLCJjYWxsYmFjayIsImRpZmYiXSwibWFwcGluZ3MiOiI7Ozs7Z0NBVWdCQSxVLEdBQUFBLFU7O0FBVmhCOzs7Ozs7dUJBRU8sSUFBTUMsaUZBQVksd0VBQWxCO0FBQ1BBLFVBQVVDLFFBQVYsR0FBcUIsVUFBU0MsS0FBVCxFQUFnQjtBQUNuQyxTQUFPQSxNQUFNQyxLQUFOLEVBQVA7QUFDRCxDQUZEO0FBR0FILFVBQVVJLElBQVYsR0FBaUJKLFVBQVVLLFdBQVYsR0FBd0IsVUFBU0gsS0FBVCxFQUFnQjtBQUN2RCxTQUFPQSxLQUFQO0FBQ0QsQ0FGRDs7QUFJTyxTQUFTSCxVQUFULENBQW9CTyxNQUFwQixFQUE0QkMsTUFBNUIsRUFBb0NDLFFBQXBDLEVBQThDO0FBQUUsU0FBT1IsVUFBVVMsSUFBVixDQUFlSCxNQUFmLEVBQXVCQyxNQUF2QixFQUErQkMsUUFBL0IsQ0FBUDtBQUFrRCIsImZpbGUiOiJhcnJheS5qcyIsInNvdXJjZXNDb250ZW50IjpbImltcG9ydCBEaWZmIGZyb20gJy4vYmFzZSc7XG5cbmV4cG9ydCBjb25zdCBhcnJheURpZmYgPSBuZXcgRGlmZigpO1xuYXJyYXlEaWZmLnRva2VuaXplID0gZnVuY3Rpb24odmFsdWUpIHtcbiAgcmV0dXJuIHZhbHVlLnNsaWNlKCk7XG59O1xuYXJyYXlEaWZmLmpvaW4gPSBhcnJheURpZmYucmVtb3ZlRW1wdHkgPSBmdW5jdGlvbih2YWx1ZSkge1xuICByZXR1cm4gdmFsdWU7XG59O1xuXG5leHBvcnQgZnVuY3Rpb24gZGlmZkFycmF5cyhvbGRBcnIsIG5ld0FyciwgY2FsbGJhY2spIHsgcmV0dXJuIGFycmF5RGlmZi5kaWZmKG9sZEFyciwgbmV3QXJyLCBjYWxsYmFjayk7IH1cbiJdfQ==
|
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,17 @@
|
|||
/*istanbul ignore start*/'use strict';
|
||||
|
||||
exports.__esModule = true;
|
||||
exports.characterDiff = undefined;
|
||||
exports. /*istanbul ignore end*/diffChars = diffChars;
|
||||
|
||||
var /*istanbul ignore start*/_base = require('./base') /*istanbul ignore end*/;
|
||||
|
||||
/*istanbul ignore start*/var _base2 = _interopRequireDefault(_base);
|
||||
|
||||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; }
|
||||
|
||||
/*istanbul ignore end*/var characterDiff = /*istanbul ignore start*/exports. /*istanbul ignore end*/characterDiff = new /*istanbul ignore start*/_base2['default'] /*istanbul ignore end*/();
|
||||
function diffChars(oldStr, newStr, options) {
|
||||
return characterDiff.diff(oldStr, newStr, options);
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy9kaWZmL2NoYXJhY3Rlci5qcyJdLCJuYW1lcyI6WyJkaWZmQ2hhcnMiLCJjaGFyYWN0ZXJEaWZmIiwib2xkU3RyIiwibmV3U3RyIiwib3B0aW9ucyIsImRpZmYiXSwibWFwcGluZ3MiOiI7Ozs7Z0NBR2dCQSxTLEdBQUFBLFM7O0FBSGhCOzs7Ozs7dUJBRU8sSUFBTUMseUZBQWdCLHdFQUF0QjtBQUNBLFNBQVNELFNBQVQsQ0FBbUJFLE1BQW5CLEVBQTJCQyxNQUEzQixFQUFtQ0MsT0FBbkMsRUFBNEM7QUFBRSxTQUFPSCxjQUFjSSxJQUFkLENBQW1CSCxNQUFuQixFQUEyQkMsTUFBM0IsRUFBbUNDLE9BQW5DLENBQVA7QUFBcUQiLCJmaWxlIjoiY2hhcmFjdGVyLmpzIiwic291cmNlc0NvbnRlbnQiOlsiaW1wb3J0IERpZmYgZnJvbSAnLi9iYXNlJztcblxuZXhwb3J0IGNvbnN0IGNoYXJhY3RlckRpZmYgPSBuZXcgRGlmZigpO1xuZXhwb3J0IGZ1bmN0aW9uIGRpZmZDaGFycyhvbGRTdHIsIG5ld1N0ciwgb3B0aW9ucykgeyByZXR1cm4gY2hhcmFjdGVyRGlmZi5kaWZmKG9sZFN0ciwgbmV3U3RyLCBvcHRpb25zKTsgfVxuIl19
|
|
@ -0,0 +1,21 @@
|
|||
/*istanbul ignore start*/'use strict';
|
||||
|
||||
exports.__esModule = true;
|
||||
exports.cssDiff = undefined;
|
||||
exports. /*istanbul ignore end*/diffCss = diffCss;
|
||||
|
||||
var /*istanbul ignore start*/_base = require('./base') /*istanbul ignore end*/;
|
||||
|
||||
/*istanbul ignore start*/var _base2 = _interopRequireDefault(_base);
|
||||
|
||||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; }
|
||||
|
||||
/*istanbul ignore end*/var cssDiff = /*istanbul ignore start*/exports. /*istanbul ignore end*/cssDiff = new /*istanbul ignore start*/_base2['default'] /*istanbul ignore end*/();
|
||||
cssDiff.tokenize = function (value) {
|
||||
return value.split(/([{}:;,]|\s+)/);
|
||||
};
|
||||
|
||||
function diffCss(oldStr, newStr, callback) {
|
||||
return cssDiff.diff(oldStr, newStr, callback);
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy9kaWZmL2Nzcy5qcyJdLCJuYW1lcyI6WyJkaWZmQ3NzIiwiY3NzRGlmZiIsInRva2VuaXplIiwidmFsdWUiLCJzcGxpdCIsIm9sZFN0ciIsIm5ld1N0ciIsImNhbGxiYWNrIiwiZGlmZiJdLCJtYXBwaW5ncyI6Ijs7OztnQ0FPZ0JBLE8sR0FBQUEsTzs7QUFQaEI7Ozs7Ozt1QkFFTyxJQUFNQyw2RUFBVSx3RUFBaEI7QUFDUEEsUUFBUUMsUUFBUixHQUFtQixVQUFTQyxLQUFULEVBQWdCO0FBQ2pDLFNBQU9BLE1BQU1DLEtBQU4sQ0FBWSxlQUFaLENBQVA7QUFDRCxDQUZEOztBQUlPLFNBQVNKLE9BQVQsQ0FBaUJLLE1BQWpCLEVBQXlCQyxNQUF6QixFQUFpQ0MsUUFBakMsRUFBMkM7QUFBRSxTQUFPTixRQUFRTyxJQUFSLENBQWFILE1BQWIsRUFBcUJDLE1BQXJCLEVBQTZCQyxRQUE3QixDQUFQO0FBQWdEIiwiZmlsZSI6ImNzcy5qcyIsInNvdXJjZXNDb250ZW50IjpbImltcG9ydCBEaWZmIGZyb20gJy4vYmFzZSc7XG5cbmV4cG9ydCBjb25zdCBjc3NEaWZmID0gbmV3IERpZmYoKTtcbmNzc0RpZmYudG9rZW5pemUgPSBmdW5jdGlvbih2YWx1ZSkge1xuICByZXR1cm4gdmFsdWUuc3BsaXQoLyhbe306OyxdfFxccyspLyk7XG59O1xuXG5leHBvcnQgZnVuY3Rpb24gZGlmZkNzcyhvbGRTdHIsIG5ld1N0ciwgY2FsbGJhY2spIHsgcmV0dXJuIGNzc0RpZmYuZGlmZihvbGRTdHIsIG5ld1N0ciwgY2FsbGJhY2spOyB9XG4iXX0=
|
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,50 @@
|
|||
/*istanbul ignore start*/'use strict';
|
||||
|
||||
exports.__esModule = true;
|
||||
exports.lineDiff = undefined;
|
||||
exports. /*istanbul ignore end*/diffLines = diffLines;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffTrimmedLines = diffTrimmedLines;
|
||||
|
||||
var /*istanbul ignore start*/_base = require('./base') /*istanbul ignore end*/;
|
||||
|
||||
/*istanbul ignore start*/var _base2 = _interopRequireDefault(_base);
|
||||
|
||||
/*istanbul ignore end*/var /*istanbul ignore start*/_params = require('../util/params') /*istanbul ignore end*/;
|
||||
|
||||
/*istanbul ignore start*/function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; }
|
||||
|
||||
/*istanbul ignore end*/var lineDiff = /*istanbul ignore start*/exports. /*istanbul ignore end*/lineDiff = new /*istanbul ignore start*/_base2['default'] /*istanbul ignore end*/();
|
||||
lineDiff.tokenize = function (value) {
|
||||
var retLines = [],
|
||||
linesAndNewlines = value.split(/(\n|\r\n)/);
|
||||
|
||||
// Ignore the final empty token that occurs if the string ends with a new line
|
||||
if (!linesAndNewlines[linesAndNewlines.length - 1]) {
|
||||
linesAndNewlines.pop();
|
||||
}
|
||||
|
||||
// Merge the content and line separators into single tokens
|
||||
for (var i = 0; i < linesAndNewlines.length; i++) {
|
||||
var line = linesAndNewlines[i];
|
||||
|
||||
if (i % 2 && !this.options.newlineIsToken) {
|
||||
retLines[retLines.length - 1] += line;
|
||||
} else {
|
||||
if (this.options.ignoreWhitespace) {
|
||||
line = line.trim();
|
||||
}
|
||||
retLines.push(line);
|
||||
}
|
||||
}
|
||||
|
||||
return retLines;
|
||||
};
|
||||
|
||||
function diffLines(oldStr, newStr, callback) {
|
||||
return lineDiff.diff(oldStr, newStr, callback);
|
||||
}
|
||||
function diffTrimmedLines(oldStr, newStr, callback) {
|
||||
var options = /*istanbul ignore start*/(0, _params.generateOptions) /*istanbul ignore end*/(callback, { ignoreWhitespace: true });
|
||||
return lineDiff.diff(oldStr, newStr, options);
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy9kaWZmL2xpbmUuanMiXSwibmFtZXMiOlsiZGlmZkxpbmVzIiwiZGlmZlRyaW1tZWRMaW5lcyIsImxpbmVEaWZmIiwidG9rZW5pemUiLCJ2YWx1ZSIsInJldExpbmVzIiwibGluZXNBbmROZXdsaW5lcyIsInNwbGl0IiwibGVuZ3RoIiwicG9wIiwiaSIsImxpbmUiLCJvcHRpb25zIiwibmV3bGluZUlzVG9rZW4iLCJpZ25vcmVXaGl0ZXNwYWNlIiwidHJpbSIsInB1c2giLCJvbGRTdHIiLCJuZXdTdHIiLCJjYWxsYmFjayIsImRpZmYiXSwibWFwcGluZ3MiOiI7Ozs7Z0NBOEJnQkEsUyxHQUFBQSxTO3lEQUNBQyxnQixHQUFBQSxnQjs7QUEvQmhCOzs7O3VCQUNBOzs7O3VCQUVPLElBQU1DLCtFQUFXLHdFQUFqQjtBQUNQQSxTQUFTQyxRQUFULEdBQW9CLFVBQVNDLEtBQVQsRUFBZ0I7QUFDbEMsTUFBSUMsV0FBVyxFQUFmO0FBQUEsTUFDSUMsbUJBQW1CRixNQUFNRyxLQUFOLENBQVksV0FBWixDQUR2Qjs7QUFHQTtBQUNBLE1BQUksQ0FBQ0QsaUJBQWlCQSxpQkFBaUJFLE1BQWpCLEdBQTBCLENBQTNDLENBQUwsRUFBb0Q7QUFDbERGLHFCQUFpQkcsR0FBakI7QUFDRDs7QUFFRDtBQUNBLE9BQUssSUFBSUMsSUFBSSxDQUFiLEVBQWdCQSxJQUFJSixpQkFBaUJFLE1BQXJDLEVBQTZDRSxHQUE3QyxFQUFrRDtBQUNoRCxRQUFJQyxPQUFPTCxpQkFBaUJJLENBQWpCLENBQVg7O0FBRUEsUUFBSUEsSUFBSSxDQUFKLElBQVMsQ0FBQyxLQUFLRSxPQUFMLENBQWFDLGNBQTNCLEVBQTJDO0FBQ3pDUixlQUFTQSxTQUFTRyxNQUFULEdBQWtCLENBQTNCLEtBQWlDRyxJQUFqQztBQUNELEtBRkQsTUFFTztBQUNMLFVBQUksS0FBS0MsT0FBTCxDQUFhRSxnQkFBakIsRUFBbUM7QUFDakNILGVBQU9BLEtBQUtJLElBQUwsRUFBUDtBQUNEO0FBQ0RWLGVBQVNXLElBQVQsQ0FBY0wsSUFBZDtBQUNEO0FBQ0Y7O0FBRUQsU0FBT04sUUFBUDtBQUNELENBeEJEOztBQTBCTyxTQUFTTCxTQUFULENBQW1CaUIsTUFBbkIsRUFBMkJDLE1BQTNCLEVBQW1DQyxRQUFuQyxFQUE2QztBQUFFLFNBQU9qQixTQUFTa0IsSUFBVCxDQUFjSCxNQUFkLEVBQXNCQyxNQUF0QixFQUE4QkMsUUFBOUIsQ0FBUDtBQUFpRDtBQUNoRyxTQUFTbEIsZ0JBQVQsQ0FBMEJnQixNQUExQixFQUFrQ0MsTUFBbEMsRUFBMENDLFFBQTFDLEVBQW9EO0FBQ3pELE1BQUlQLFVBQVUsOEVBQWdCTyxRQUFoQixFQUEwQixFQUFDTCxrQkFBa0IsSUFBbkIsRUFBMUIsQ0FBZDtBQUNBLFNBQU9aLFNBQVNrQixJQUFULENBQWNILE1BQWQsRUFBc0JDLE1BQXRCLEVBQThCTixPQUE5QixDQUFQO0FBQ0QiLCJmaWxlIjoibGluZS5qcyIsInNvdXJjZXNDb250ZW50IjpbImltcG9ydCBEaWZmIGZyb20gJy4vYmFzZSc7XG5pbXBvcnQge2dlbmVyYXRlT3B0aW9uc30gZnJvbSAnLi4vdXRpbC9wYXJhbXMnO1xuXG5leHBvcnQgY29uc3QgbGluZURpZmYgPSBuZXcgRGlmZigpO1xubGluZURpZmYudG9rZW5pemUgPSBmdW5jdGlvbih2YWx1ZSkge1xuICBsZXQgcmV0TGluZXMgPSBbXSxcbiAgICAgIGxpbmVzQW5kTmV3bGluZXMgPSB2YWx1ZS5zcGxpdCgvKFxcbnxcXHJcXG4pLyk7XG5cbiAgLy8gSWdub3JlIHRoZSBmaW5hbCBlbXB0eSB0b2tlbiB0aGF0IG9jY3VycyBpZiB0aGUgc3RyaW5nIGVuZHMgd2l0aCBhIG5ldyBsaW5lXG4gIGlmICghbGluZXNBbmROZXdsaW5lc1tsaW5lc0FuZE5ld2xpbmVzLmxlbmd0aCAtIDFdKSB7XG4gICAgbGluZXNBbmROZXdsaW5lcy5wb3AoKTtcbiAgfVxuXG4gIC8vIE1lcmdlIHRoZSBjb250ZW50IGFuZCBsaW5lIHNlcGFyYXRvcnMgaW50byBzaW5nbGUgdG9rZW5zXG4gIGZvciAobGV0IGkgPSAwOyBpIDwgbGluZXNBbmROZXdsaW5lcy5sZW5ndGg7IGkrKykge1xuICAgIGxldCBsaW5lID0gbGluZXNBbmROZXdsaW5lc1tpXTtcblxuICAgIGlmIChpICUgMiAmJiAhdGhpcy5vcHRpb25zLm5ld2xpbmVJc1Rva2VuKSB7XG4gICAgICByZXRMaW5lc1tyZXRMaW5lcy5sZW5ndGggLSAxXSArPSBsaW5lO1xuICAgIH0gZWxzZSB7XG4gICAgICBpZiAodGhpcy5vcHRpb25zLmlnbm9yZVdoaXRlc3BhY2UpIHtcbiAgICAgICAgbGluZSA9IGxpbmUudHJpbSgpO1xuICAgICAgfVxuICAgICAgcmV0TGluZXMucHVzaChsaW5lKTtcbiAgICB9XG4gIH1cblxuICByZXR1cm4gcmV0TGluZXM7XG59O1xuXG5leHBvcnQgZnVuY3Rpb24gZGlmZkxpbmVzKG9sZFN0ciwgbmV3U3RyLCBjYWxsYmFjaykgeyByZXR1cm4gbGluZURpZmYuZGlmZihvbGRTdHIsIG5ld1N0ciwgY2FsbGJhY2spOyB9XG5leHBvcnQgZnVuY3Rpb24gZGlmZlRyaW1tZWRMaW5lcyhvbGRTdHIsIG5ld1N0ciwgY2FsbGJhY2spIHtcbiAgbGV0IG9wdGlvbnMgPSBnZW5lcmF0ZU9wdGlvbnMoY2FsbGJhY2ssIHtpZ25vcmVXaGl0ZXNwYWNlOiB0cnVlfSk7XG4gIHJldHVybiBsaW5lRGlmZi5kaWZmKG9sZFN0ciwgbmV3U3RyLCBvcHRpb25zKTtcbn1cbiJdfQ==
|
|
@ -0,0 +1,21 @@
|
|||
/*istanbul ignore start*/'use strict';
|
||||
|
||||
exports.__esModule = true;
|
||||
exports.sentenceDiff = undefined;
|
||||
exports. /*istanbul ignore end*/diffSentences = diffSentences;
|
||||
|
||||
var /*istanbul ignore start*/_base = require('./base') /*istanbul ignore end*/;
|
||||
|
||||
/*istanbul ignore start*/var _base2 = _interopRequireDefault(_base);
|
||||
|
||||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; }
|
||||
|
||||
/*istanbul ignore end*/var sentenceDiff = /*istanbul ignore start*/exports. /*istanbul ignore end*/sentenceDiff = new /*istanbul ignore start*/_base2['default'] /*istanbul ignore end*/();
|
||||
sentenceDiff.tokenize = function (value) {
|
||||
return value.split(/(\S.+?[.!?])(?=\s+|$)/);
|
||||
};
|
||||
|
||||
function diffSentences(oldStr, newStr, callback) {
|
||||
return sentenceDiff.diff(oldStr, newStr, callback);
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy9kaWZmL3NlbnRlbmNlLmpzIl0sIm5hbWVzIjpbImRpZmZTZW50ZW5jZXMiLCJzZW50ZW5jZURpZmYiLCJ0b2tlbml6ZSIsInZhbHVlIiwic3BsaXQiLCJvbGRTdHIiLCJuZXdTdHIiLCJjYWxsYmFjayIsImRpZmYiXSwibWFwcGluZ3MiOiI7Ozs7Z0NBUWdCQSxhLEdBQUFBLGE7O0FBUmhCOzs7Ozs7dUJBR08sSUFBTUMsdUZBQWUsd0VBQXJCO0FBQ1BBLGFBQWFDLFFBQWIsR0FBd0IsVUFBU0MsS0FBVCxFQUFnQjtBQUN0QyxTQUFPQSxNQUFNQyxLQUFOLENBQVksdUJBQVosQ0FBUDtBQUNELENBRkQ7O0FBSU8sU0FBU0osYUFBVCxDQUF1QkssTUFBdkIsRUFBK0JDLE1BQS9CLEVBQXVDQyxRQUF2QyxFQUFpRDtBQUFFLFNBQU9OLGFBQWFPLElBQWIsQ0FBa0JILE1BQWxCLEVBQTBCQyxNQUExQixFQUFrQ0MsUUFBbEMsQ0FBUDtBQUFxRCIsImZpbGUiOiJzZW50ZW5jZS5qcyIsInNvdXJjZXNDb250ZW50IjpbImltcG9ydCBEaWZmIGZyb20gJy4vYmFzZSc7XG5cblxuZXhwb3J0IGNvbnN0IHNlbnRlbmNlRGlmZiA9IG5ldyBEaWZmKCk7XG5zZW50ZW5jZURpZmYudG9rZW5pemUgPSBmdW5jdGlvbih2YWx1ZSkge1xuICByZXR1cm4gdmFsdWUuc3BsaXQoLyhcXFMuKz9bLiE/XSkoPz1cXHMrfCQpLyk7XG59O1xuXG5leHBvcnQgZnVuY3Rpb24gZGlmZlNlbnRlbmNlcyhvbGRTdHIsIG5ld1N0ciwgY2FsbGJhY2spIHsgcmV0dXJuIHNlbnRlbmNlRGlmZi5kaWZmKG9sZFN0ciwgbmV3U3RyLCBjYWxsYmFjayk7IH1cbiJdfQ==
|
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,74 @@
|
|||
/*istanbul ignore start*/'use strict';
|
||||
|
||||
exports.__esModule = true;
|
||||
exports.canonicalize = exports.convertChangesToXML = exports.convertChangesToDMP = exports.merge = exports.parsePatch = exports.applyPatches = exports.applyPatch = exports.createPatch = exports.createTwoFilesPatch = exports.structuredPatch = exports.diffArrays = exports.diffJson = exports.diffCss = exports.diffSentences = exports.diffTrimmedLines = exports.diffLines = exports.diffWordsWithSpace = exports.diffWords = exports.diffChars = exports.Diff = undefined;
|
||||
|
||||
/*istanbul ignore end*/var /*istanbul ignore start*/_base = require('./diff/base') /*istanbul ignore end*/;
|
||||
|
||||
/*istanbul ignore start*/var _base2 = _interopRequireDefault(_base);
|
||||
|
||||
/*istanbul ignore end*/var /*istanbul ignore start*/_character = require('./diff/character') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_word = require('./diff/word') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_line = require('./diff/line') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_sentence = require('./diff/sentence') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_css = require('./diff/css') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_json = require('./diff/json') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_array = require('./diff/array') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_apply = require('./patch/apply') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_parse = require('./patch/parse') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_merge = require('./patch/merge') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_create = require('./patch/create') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_dmp = require('./convert/dmp') /*istanbul ignore end*/;
|
||||
|
||||
var /*istanbul ignore start*/_xml = require('./convert/xml') /*istanbul ignore end*/;
|
||||
|
||||
/*istanbul ignore start*/function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; }
|
||||
|
||||
/* See LICENSE file for terms of use */
|
||||
|
||||
/*
|
||||
* Text diff implementation.
|
||||
*
|
||||
* This library supports the following APIS:
|
||||
* JsDiff.diffChars: Character by character diff
|
||||
* JsDiff.diffWords: Word (as defined by \b regex) diff which ignores whitespace
|
||||
* JsDiff.diffLines: Line based diff
|
||||
*
|
||||
* JsDiff.diffCss: Diff targeted at CSS content
|
||||
*
|
||||
* These methods are based on the implementation proposed in
|
||||
* "An O(ND) Difference Algorithm and its Variations" (Myers, 1986).
|
||||
* http://citeseerx.ist.psu.edu/viewdoc/summary?doi=10.1.1.4.6927
|
||||
*/
|
||||
exports. /*istanbul ignore end*/Diff = _base2['default'];
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffChars = _character.diffChars;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffWords = _word.diffWords;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffWordsWithSpace = _word.diffWordsWithSpace;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffLines = _line.diffLines;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffTrimmedLines = _line.diffTrimmedLines;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffSentences = _sentence.diffSentences;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffCss = _css.diffCss;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffJson = _json.diffJson;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/diffArrays = _array.diffArrays;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/structuredPatch = _create.structuredPatch;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/createTwoFilesPatch = _create.createTwoFilesPatch;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/createPatch = _create.createPatch;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/applyPatch = _apply.applyPatch;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/applyPatches = _apply.applyPatches;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/parsePatch = _parse.parsePatch;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/merge = _merge.merge;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/convertChangesToDMP = _dmp.convertChangesToDMP;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/convertChangesToXML = _xml.convertChangesToXML;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/canonicalize = _json.canonicalize;
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uL3NyYy9pbmRleC5qcyJdLCJuYW1lcyI6WyJEaWZmIiwiZGlmZkNoYXJzIiwiZGlmZldvcmRzIiwiZGlmZldvcmRzV2l0aFNwYWNlIiwiZGlmZkxpbmVzIiwiZGlmZlRyaW1tZWRMaW5lcyIsImRpZmZTZW50ZW5jZXMiLCJkaWZmQ3NzIiwiZGlmZkpzb24iLCJkaWZmQXJyYXlzIiwic3RydWN0dXJlZFBhdGNoIiwiY3JlYXRlVHdvRmlsZXNQYXRjaCIsImNyZWF0ZVBhdGNoIiwiYXBwbHlQYXRjaCIsImFwcGx5UGF0Y2hlcyIsInBhcnNlUGF0Y2giLCJtZXJnZSIsImNvbnZlcnRDaGFuZ2VzVG9ETVAiLCJjb252ZXJ0Q2hhbmdlc1RvWE1MIiwiY2Fub25pY2FsaXplIl0sIm1hcHBpbmdzIjoiOzs7Ozt1QkFnQkE7Ozs7dUJBQ0E7O0FBQ0E7O0FBQ0E7O0FBQ0E7O0FBRUE7O0FBQ0E7O0FBRUE7O0FBRUE7O0FBQ0E7O0FBQ0E7O0FBQ0E7O0FBRUE7O0FBQ0E7Ozs7QUFqQ0E7O0FBRUE7Ozs7Ozs7Ozs7Ozs7O2dDQWtDRUEsSTt5REFFQUMsUzt5REFDQUMsUzt5REFDQUMsa0I7eURBQ0FDLFM7eURBQ0FDLGdCO3lEQUNBQyxhO3lEQUVBQyxPO3lEQUNBQyxRO3lEQUVBQyxVO3lEQUVBQyxlO3lEQUNBQyxtQjt5REFDQUMsVzt5REFDQUMsVTt5REFDQUMsWTt5REFDQUMsVTt5REFDQUMsSzt5REFDQUMsbUI7eURBQ0FDLG1CO3lEQUNBQyxZIiwiZmlsZSI6ImluZGV4LmpzIiwic291cmNlc0NvbnRlbnQiOlsiLyogU2VlIExJQ0VOU0UgZmlsZSBmb3IgdGVybXMgb2YgdXNlICovXG5cbi8qXG4gKiBUZXh0IGRpZmYgaW1wbGVtZW50YXRpb24uXG4gKlxuICogVGhpcyBsaWJyYXJ5IHN1cHBvcnRzIHRoZSBmb2xsb3dpbmcgQVBJUzpcbiAqIEpzRGlmZi5kaWZmQ2hhcnM6IENoYXJhY3RlciBieSBjaGFyYWN0ZXIgZGlmZlxuICogSnNEaWZmLmRpZmZXb3JkczogV29yZCAoYXMgZGVmaW5lZCBieSBcXGIgcmVnZXgpIGRpZmYgd2hpY2ggaWdub3JlcyB3aGl0ZXNwYWNlXG4gKiBKc0RpZmYuZGlmZkxpbmVzOiBMaW5lIGJhc2VkIGRpZmZcbiAqXG4gKiBKc0RpZmYuZGlmZkNzczogRGlmZiB0YXJnZXRlZCBhdCBDU1MgY29udGVudFxuICpcbiAqIFRoZXNlIG1ldGhvZHMgYXJlIGJhc2VkIG9uIHRoZSBpbXBsZW1lbnRhdGlvbiBwcm9wb3NlZCBpblxuICogXCJBbiBPKE5EKSBEaWZmZXJlbmNlIEFsZ29yaXRobSBhbmQgaXRzIFZhcmlhdGlvbnNcIiAoTXllcnMsIDE5ODYpLlxuICogaHR0cDovL2NpdGVzZWVyeC5pc3QucHN1LmVkdS92aWV3ZG9jL3N1bW1hcnk/ZG9pPTEwLjEuMS40LjY5MjdcbiAqL1xuaW1wb3J0IERpZmYgZnJvbSAnLi9kaWZmL2Jhc2UnO1xuaW1wb3J0IHtkaWZmQ2hhcnN9IGZyb20gJy4vZGlmZi9jaGFyYWN0ZXInO1xuaW1wb3J0IHtkaWZmV29yZHMsIGRpZmZXb3Jkc1dpdGhTcGFjZX0gZnJvbSAnLi9kaWZmL3dvcmQnO1xuaW1wb3J0IHtkaWZmTGluZXMsIGRpZmZUcmltbWVkTGluZXN9IGZyb20gJy4vZGlmZi9saW5lJztcbmltcG9ydCB7ZGlmZlNlbnRlbmNlc30gZnJvbSAnLi9kaWZmL3NlbnRlbmNlJztcblxuaW1wb3J0IHtkaWZmQ3NzfSBmcm9tICcuL2RpZmYvY3NzJztcbmltcG9ydCB7ZGlmZkpzb24sIGNhbm9uaWNhbGl6ZX0gZnJvbSAnLi9kaWZmL2pzb24nO1xuXG5pbXBvcnQge2RpZmZBcnJheXN9IGZyb20gJy4vZGlmZi9hcnJheSc7XG5cbmltcG9ydCB7YXBwbHlQYXRjaCwgYXBwbHlQYXRjaGVzfSBmcm9tICcuL3BhdGNoL2FwcGx5JztcbmltcG9ydCB7cGFyc2VQYXRjaH0gZnJvbSAnLi9wYXRjaC9wYXJzZSc7XG5pbXBvcnQge21lcmdlfSBmcm9tICcuL3BhdGNoL21lcmdlJztcbmltcG9ydCB7c3RydWN0dXJlZFBhdGNoLCBjcmVhdGVUd29GaWxlc1BhdGNoLCBjcmVhdGVQYXRjaH0gZnJvbSAnLi9wYXRjaC9jcmVhdGUnO1xuXG5pbXBvcnQge2NvbnZlcnRDaGFuZ2VzVG9ETVB9IGZyb20gJy4vY29udmVydC9kbXAnO1xuaW1wb3J0IHtjb252ZXJ0Q2hhbmdlc1RvWE1MfSBmcm9tICcuL2NvbnZlcnQveG1sJztcblxuZXhwb3J0IHtcbiAgRGlmZixcblxuICBkaWZmQ2hhcnMsXG4gIGRpZmZXb3JkcyxcbiAgZGlmZldvcmRzV2l0aFNwYWNlLFxuICBkaWZmTGluZXMsXG4gIGRpZmZUcmltbWVkTGluZXMsXG4gIGRpZmZTZW50ZW5jZXMsXG5cbiAgZGlmZkNzcyxcbiAgZGlmZkpzb24sXG5cbiAgZGlmZkFycmF5cyxcblxuICBzdHJ1Y3R1cmVkUGF0Y2gsXG4gIGNyZWF0ZVR3b0ZpbGVzUGF0Y2gsXG4gIGNyZWF0ZVBhdGNoLFxuICBhcHBseVBhdGNoLFxuICBhcHBseVBhdGNoZXMsXG4gIHBhcnNlUGF0Y2gsXG4gIG1lcmdlLFxuICBjb252ZXJ0Q2hhbmdlc1RvRE1QLFxuICBjb252ZXJ0Q2hhbmdlc1RvWE1MLFxuICBjYW5vbmljYWxpemVcbn07XG4iXX0=
|
File diff suppressed because one or more lines are too long
File diff suppressed because one or more lines are too long
File diff suppressed because one or more lines are too long
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,27 @@
|
|||
/*istanbul ignore start*/"use strict";
|
||||
|
||||
exports.__esModule = true;
|
||||
exports. /*istanbul ignore end*/arrayEqual = arrayEqual;
|
||||
/*istanbul ignore start*/exports. /*istanbul ignore end*/arrayStartsWith = arrayStartsWith;
|
||||
function arrayEqual(a, b) {
|
||||
if (a.length !== b.length) {
|
||||
return false;
|
||||
}
|
||||
|
||||
return arrayStartsWith(a, b);
|
||||
}
|
||||
|
||||
function arrayStartsWith(array, start) {
|
||||
if (start.length > array.length) {
|
||||
return false;
|
||||
}
|
||||
|
||||
for (var i = 0; i < start.length; i++) {
|
||||
if (start[i] !== array[i]) {
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
return true;
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy91dGlsL2FycmF5LmpzIl0sIm5hbWVzIjpbImFycmF5RXF1YWwiLCJhcnJheVN0YXJ0c1dpdGgiLCJhIiwiYiIsImxlbmd0aCIsImFycmF5Iiwic3RhcnQiLCJpIl0sIm1hcHBpbmdzIjoiOzs7Z0NBQWdCQSxVLEdBQUFBLFU7eURBUUFDLGUsR0FBQUEsZTtBQVJULFNBQVNELFVBQVQsQ0FBb0JFLENBQXBCLEVBQXVCQyxDQUF2QixFQUEwQjtBQUMvQixNQUFJRCxFQUFFRSxNQUFGLEtBQWFELEVBQUVDLE1BQW5CLEVBQTJCO0FBQ3pCLFdBQU8sS0FBUDtBQUNEOztBQUVELFNBQU9ILGdCQUFnQkMsQ0FBaEIsRUFBbUJDLENBQW5CLENBQVA7QUFDRDs7QUFFTSxTQUFTRixlQUFULENBQXlCSSxLQUF6QixFQUFnQ0MsS0FBaEMsRUFBdUM7QUFDNUMsTUFBSUEsTUFBTUYsTUFBTixHQUFlQyxNQUFNRCxNQUF6QixFQUFpQztBQUMvQixXQUFPLEtBQVA7QUFDRDs7QUFFRCxPQUFLLElBQUlHLElBQUksQ0FBYixFQUFnQkEsSUFBSUQsTUFBTUYsTUFBMUIsRUFBa0NHLEdBQWxDLEVBQXVDO0FBQ3JDLFFBQUlELE1BQU1DLENBQU4sTUFBYUYsTUFBTUUsQ0FBTixDQUFqQixFQUEyQjtBQUN6QixhQUFPLEtBQVA7QUFDRDtBQUNGOztBQUVELFNBQU8sSUFBUDtBQUNEIiwiZmlsZSI6ImFycmF5LmpzIiwic291cmNlc0NvbnRlbnQiOlsiZXhwb3J0IGZ1bmN0aW9uIGFycmF5RXF1YWwoYSwgYikge1xuICBpZiAoYS5sZW5ndGggIT09IGIubGVuZ3RoKSB7XG4gICAgcmV0dXJuIGZhbHNlO1xuICB9XG5cbiAgcmV0dXJuIGFycmF5U3RhcnRzV2l0aChhLCBiKTtcbn1cblxuZXhwb3J0IGZ1bmN0aW9uIGFycmF5U3RhcnRzV2l0aChhcnJheSwgc3RhcnQpIHtcbiAgaWYgKHN0YXJ0Lmxlbmd0aCA+IGFycmF5Lmxlbmd0aCkge1xuICAgIHJldHVybiBmYWxzZTtcbiAgfVxuXG4gIGZvciAobGV0IGkgPSAwOyBpIDwgc3RhcnQubGVuZ3RoOyBpKyspIHtcbiAgICBpZiAoc3RhcnRbaV0gIT09IGFycmF5W2ldKSB7XG4gICAgICByZXR1cm4gZmFsc2U7XG4gICAgfVxuICB9XG5cbiAgcmV0dXJuIHRydWU7XG59XG4iXX0=
|
|
@ -0,0 +1,47 @@
|
|||
/*istanbul ignore start*/"use strict";
|
||||
|
||||
exports.__esModule = true;
|
||||
|
||||
exports["default"] = /*istanbul ignore end*/function (start, minLine, maxLine) {
|
||||
var wantForward = true,
|
||||
backwardExhausted = false,
|
||||
forwardExhausted = false,
|
||||
localOffset = 1;
|
||||
|
||||
return function iterator() {
|
||||
if (wantForward && !forwardExhausted) {
|
||||
if (backwardExhausted) {
|
||||
localOffset++;
|
||||
} else {
|
||||
wantForward = false;
|
||||
}
|
||||
|
||||
// Check if trying to fit beyond text length, and if not, check it fits
|
||||
// after offset location (or desired location on first iteration)
|
||||
if (start + localOffset <= maxLine) {
|
||||
return localOffset;
|
||||
}
|
||||
|
||||
forwardExhausted = true;
|
||||
}
|
||||
|
||||
if (!backwardExhausted) {
|
||||
if (!forwardExhausted) {
|
||||
wantForward = true;
|
||||
}
|
||||
|
||||
// Check if trying to fit before text beginning, and if not, check it fits
|
||||
// before offset location
|
||||
if (minLine <= start - localOffset) {
|
||||
return -localOffset++;
|
||||
}
|
||||
|
||||
backwardExhausted = true;
|
||||
return iterator();
|
||||
}
|
||||
|
||||
// We tried to fit hunk before text beginning and beyond text length, then
|
||||
// hunk can't fit on the text. Return undefined
|
||||
};
|
||||
};
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy91dGlsL2Rpc3RhbmNlLWl0ZXJhdG9yLmpzIl0sIm5hbWVzIjpbInN0YXJ0IiwibWluTGluZSIsIm1heExpbmUiLCJ3YW50Rm9yd2FyZCIsImJhY2t3YXJkRXhoYXVzdGVkIiwiZm9yd2FyZEV4aGF1c3RlZCIsImxvY2FsT2Zmc2V0IiwiaXRlcmF0b3IiXSwibWFwcGluZ3MiOiI7Ozs7NENBR2UsVUFBU0EsS0FBVCxFQUFnQkMsT0FBaEIsRUFBeUJDLE9BQXpCLEVBQWtDO0FBQy9DLE1BQUlDLGNBQWMsSUFBbEI7QUFBQSxNQUNJQyxvQkFBb0IsS0FEeEI7QUFBQSxNQUVJQyxtQkFBbUIsS0FGdkI7QUFBQSxNQUdJQyxjQUFjLENBSGxCOztBQUtBLFNBQU8sU0FBU0MsUUFBVCxHQUFvQjtBQUN6QixRQUFJSixlQUFlLENBQUNFLGdCQUFwQixFQUFzQztBQUNwQyxVQUFJRCxpQkFBSixFQUF1QjtBQUNyQkU7QUFDRCxPQUZELE1BRU87QUFDTEgsc0JBQWMsS0FBZDtBQUNEOztBQUVEO0FBQ0E7QUFDQSxVQUFJSCxRQUFRTSxXQUFSLElBQXVCSixPQUEzQixFQUFvQztBQUNsQyxlQUFPSSxXQUFQO0FBQ0Q7O0FBRURELHlCQUFtQixJQUFuQjtBQUNEOztBQUVELFFBQUksQ0FBQ0QsaUJBQUwsRUFBd0I7QUFDdEIsVUFBSSxDQUFDQyxnQkFBTCxFQUF1QjtBQUNyQkYsc0JBQWMsSUFBZDtBQUNEOztBQUVEO0FBQ0E7QUFDQSxVQUFJRixXQUFXRCxRQUFRTSxXQUF2QixFQUFvQztBQUNsQyxlQUFPLENBQUNBLGFBQVI7QUFDRDs7QUFFREYsMEJBQW9CLElBQXBCO0FBQ0EsYUFBT0csVUFBUDtBQUNEOztBQUVEO0FBQ0E7QUFDRCxHQWxDRDtBQW1DRCxDIiwiZmlsZSI6ImRpc3RhbmNlLWl0ZXJhdG9yLmpzIiwic291cmNlc0NvbnRlbnQiOlsiLy8gSXRlcmF0b3IgdGhhdCB0cmF2ZXJzZXMgaW4gdGhlIHJhbmdlIG9mIFttaW4sIG1heF0sIHN0ZXBwaW5nXG4vLyBieSBkaXN0YW5jZSBmcm9tIGEgZ2l2ZW4gc3RhcnQgcG9zaXRpb24uIEkuZS4gZm9yIFswLCA0XSwgd2l0aFxuLy8gc3RhcnQgb2YgMiwgdGhpcyB3aWxsIGl0ZXJhdGUgMiwgMywgMSwgNCwgMC5cbmV4cG9ydCBkZWZhdWx0IGZ1bmN0aW9uKHN0YXJ0LCBtaW5MaW5lLCBtYXhMaW5lKSB7XG4gIGxldCB3YW50Rm9yd2FyZCA9IHRydWUsXG4gICAgICBiYWNrd2FyZEV4aGF1c3RlZCA9IGZhbHNlLFxuICAgICAgZm9yd2FyZEV4aGF1c3RlZCA9IGZhbHNlLFxuICAgICAgbG9jYWxPZmZzZXQgPSAxO1xuXG4gIHJldHVybiBmdW5jdGlvbiBpdGVyYXRvcigpIHtcbiAgICBpZiAod2FudEZvcndhcmQgJiYgIWZvcndhcmRFeGhhdXN0ZWQpIHtcbiAgICAgIGlmIChiYWNrd2FyZEV4aGF1c3RlZCkge1xuICAgICAgICBsb2NhbE9mZnNldCsrO1xuICAgICAgfSBlbHNlIHtcbiAgICAgICAgd2FudEZvcndhcmQgPSBmYWxzZTtcbiAgICAgIH1cblxuICAgICAgLy8gQ2hlY2sgaWYgdHJ5aW5nIHRvIGZpdCBiZXlvbmQgdGV4dCBsZW5ndGgsIGFuZCBpZiBub3QsIGNoZWNrIGl0IGZpdHNcbiAgICAgIC8vIGFmdGVyIG9mZnNldCBsb2NhdGlvbiAob3IgZGVzaXJlZCBsb2NhdGlvbiBvbiBmaXJzdCBpdGVyYXRpb24pXG4gICAgICBpZiAoc3RhcnQgKyBsb2NhbE9mZnNldCA8PSBtYXhMaW5lKSB7XG4gICAgICAgIHJldHVybiBsb2NhbE9mZnNldDtcbiAgICAgIH1cblxuICAgICAgZm9yd2FyZEV4aGF1c3RlZCA9IHRydWU7XG4gICAgfVxuXG4gICAgaWYgKCFiYWNrd2FyZEV4aGF1c3RlZCkge1xuICAgICAgaWYgKCFmb3J3YXJkRXhoYXVzdGVkKSB7XG4gICAgICAgIHdhbnRGb3J3YXJkID0gdHJ1ZTtcbiAgICAgIH1cblxuICAgICAgLy8gQ2hlY2sgaWYgdHJ5aW5nIHRvIGZpdCBiZWZvcmUgdGV4dCBiZWdpbm5pbmcsIGFuZCBpZiBub3QsIGNoZWNrIGl0IGZpdHNcbiAgICAgIC8vIGJlZm9yZSBvZmZzZXQgbG9jYXRpb25cbiAgICAgIGlmIChtaW5MaW5lIDw9IHN0YXJ0IC0gbG9jYWxPZmZzZXQpIHtcbiAgICAgICAgcmV0dXJuIC1sb2NhbE9mZnNldCsrO1xuICAgICAgfVxuXG4gICAgICBiYWNrd2FyZEV4aGF1c3RlZCA9IHRydWU7XG4gICAgICByZXR1cm4gaXRlcmF0b3IoKTtcbiAgICB9XG5cbiAgICAvLyBXZSB0cmllZCB0byBmaXQgaHVuayBiZWZvcmUgdGV4dCBiZWdpbm5pbmcgYW5kIGJleW9uZCB0ZXh0IGxlbmd0aCwgdGhlblxuICAgIC8vIGh1bmsgY2FuJ3QgZml0IG9uIHRoZSB0ZXh0LiBSZXR1cm4gdW5kZWZpbmVkXG4gIH07XG59XG4iXX0=
|
|
@ -0,0 +1,18 @@
|
|||
/*istanbul ignore start*/'use strict';
|
||||
|
||||
exports.__esModule = true;
|
||||
exports. /*istanbul ignore end*/generateOptions = generateOptions;
|
||||
function generateOptions(options, defaults) {
|
||||
if (typeof options === 'function') {
|
||||
defaults.callback = options;
|
||||
} else if (options) {
|
||||
for (var name in options) {
|
||||
/* istanbul ignore else */
|
||||
if (options.hasOwnProperty(name)) {
|
||||
defaults[name] = options[name];
|
||||
}
|
||||
}
|
||||
}
|
||||
return defaults;
|
||||
}
|
||||
//# sourceMappingURL=data:application/json;charset=utf-8;base64,eyJ2ZXJzaW9uIjozLCJzb3VyY2VzIjpbIi4uLy4uL3NyYy91dGlsL3BhcmFtcy5qcyJdLCJuYW1lcyI6WyJnZW5lcmF0ZU9wdGlvbnMiLCJvcHRpb25zIiwiZGVmYXVsdHMiLCJjYWxsYmFjayIsIm5hbWUiLCJoYXNPd25Qcm9wZXJ0eSJdLCJtYXBwaW5ncyI6Ijs7O2dDQUFnQkEsZSxHQUFBQSxlO0FBQVQsU0FBU0EsZUFBVCxDQUF5QkMsT0FBekIsRUFBa0NDLFFBQWxDLEVBQTRDO0FBQ2pELE1BQUksT0FBT0QsT0FBUCxLQUFtQixVQUF2QixFQUFtQztBQUNqQ0MsYUFBU0MsUUFBVCxHQUFvQkYsT0FBcEI7QUFDRCxHQUZELE1BRU8sSUFBSUEsT0FBSixFQUFhO0FBQ2xCLFNBQUssSUFBSUcsSUFBVCxJQUFpQkgsT0FBakIsRUFBMEI7QUFDeEI7QUFDQSxVQUFJQSxRQUFRSSxjQUFSLENBQXVCRCxJQUF2QixDQUFKLEVBQWtDO0FBQ2hDRixpQkFBU0UsSUFBVCxJQUFpQkgsUUFBUUcsSUFBUixDQUFqQjtBQUNEO0FBQ0Y7QUFDRjtBQUNELFNBQU9GLFFBQVA7QUFDRCIsImZpbGUiOiJwYXJhbXMuanMiLCJzb3VyY2VzQ29udGVudCI6WyJleHBvcnQgZnVuY3Rpb24gZ2VuZXJhdGVPcHRpb25zKG9wdGlvbnMsIGRlZmF1bHRzKSB7XG4gIGlmICh0eXBlb2Ygb3B0aW9ucyA9PT0gJ2Z1bmN0aW9uJykge1xuICAgIGRlZmF1bHRzLmNhbGxiYWNrID0gb3B0aW9ucztcbiAgfSBlbHNlIGlmIChvcHRpb25zKSB7XG4gICAgZm9yIChsZXQgbmFtZSBpbiBvcHRpb25zKSB7XG4gICAgICAvKiBpc3RhbmJ1bCBpZ25vcmUgZWxzZSAqL1xuICAgICAgaWYgKG9wdGlvbnMuaGFzT3duUHJvcGVydHkobmFtZSkpIHtcbiAgICAgICAgZGVmYXVsdHNbbmFtZV0gPSBvcHRpb25zW25hbWVdO1xuICAgICAgfVxuICAgIH1cbiAgfVxuICByZXR1cm4gZGVmYXVsdHM7XG59XG4iXX0=
|
|
@ -0,0 +1,67 @@
|
|||
{
|
||||
"name": "diff",
|
||||
"version": "3.5.0",
|
||||
"description": "A javascript text diff implementation.",
|
||||
"keywords": [
|
||||
"diff",
|
||||
"javascript"
|
||||
],
|
||||
"maintainers": [
|
||||
"Kevin Decker <kpdecker@gmail.com> (http://incaseofstairs.com)"
|
||||
],
|
||||
"bugs": {
|
||||
"email": "kpdecker@gmail.com",
|
||||
"url": "http://github.com/kpdecker/jsdiff/issues"
|
||||
},
|
||||
"license": "BSD-3-Clause",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/kpdecker/jsdiff.git"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.3.1"
|
||||
},
|
||||
"main": "./lib",
|
||||
"browser": "./dist/diff.js",
|
||||
"scripts": {
|
||||
"test": "grunt"
|
||||
},
|
||||
"dependencies": {},
|
||||
"devDependencies": {
|
||||
"async": "^1.4.2",
|
||||
"babel-core": "^6.0.0",
|
||||
"babel-loader": "^6.0.0",
|
||||
"babel-preset-es2015-mod": "^6.3.13",
|
||||
"babel-preset-es3": "^1.0.1",
|
||||
"chai": "^3.3.0",
|
||||
"colors": "^1.1.2",
|
||||
"eslint": "^1.6.0",
|
||||
"grunt": "^0.4.5",
|
||||
"grunt-babel": "^6.0.0",
|
||||
"grunt-clean": "^0.4.0",
|
||||
"grunt-cli": "^0.1.13",
|
||||
"grunt-contrib-clean": "^1.0.0",
|
||||
"grunt-contrib-copy": "^1.0.0",
|
||||
"grunt-contrib-uglify": "^1.0.0",
|
||||
"grunt-contrib-watch": "^1.0.0",
|
||||
"grunt-eslint": "^17.3.1",
|
||||
"grunt-karma": "^0.12.1",
|
||||
"grunt-mocha-istanbul": "^3.0.1",
|
||||
"grunt-mocha-test": "^0.12.7",
|
||||
"grunt-webpack": "^1.0.11",
|
||||
"istanbul": "github:kpdecker/istanbul",
|
||||
"karma": "^0.13.11",
|
||||
"karma-mocha": "^0.2.0",
|
||||
"karma-mocha-reporter": "^2.0.0",
|
||||
"karma-phantomjs-launcher": "^1.0.0",
|
||||
"karma-sauce-launcher": "^0.3.0",
|
||||
"karma-sourcemap-loader": "^0.3.6",
|
||||
"karma-webpack": "^1.7.0",
|
||||
"mocha": "^2.3.3",
|
||||
"phantomjs-prebuilt": "^2.1.5",
|
||||
"semver": "^5.0.3",
|
||||
"webpack": "^1.12.2",
|
||||
"webpack-dev-server": "^1.12.0"
|
||||
},
|
||||
"optionalDependencies": {}
|
||||
}
|
|
@ -0,0 +1,247 @@
|
|||
# Release Notes
|
||||
|
||||
## Development
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.5.0...master)
|
||||
|
||||
## v3.5.0 - March 4th, 2018
|
||||
- Omit redundant slice in join method of diffArrays - 1023590
|
||||
- Support patches with empty lines - fb0f208
|
||||
- Accept a custom JSON replacer function for JSON diffing - 69c7f0a
|
||||
- Optimize parch header parser - 2aec429
|
||||
- Fix typos - e89c832
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.5.0...v3.5.0)
|
||||
|
||||
## v3.5.0 - March 4th, 2018
|
||||
- Omit redundant slice in join method of diffArrays - 1023590
|
||||
- Support patches with empty lines - fb0f208
|
||||
- Accept a custom JSON replacer function for JSON diffing - 69c7f0a
|
||||
- Optimize parch header parser - 2aec429
|
||||
- Fix typos - e89c832
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.4.0...v3.5.0)
|
||||
|
||||
## v3.4.0 - October 7th, 2017
|
||||
- [#183](https://github.com/kpdecker/jsdiff/issues/183) - Feature request: ability to specify a custom equality checker for `diffArrays`
|
||||
- [#173](https://github.com/kpdecker/jsdiff/issues/173) - Bug: diffArrays gives wrong result on array of booleans
|
||||
- [#158](https://github.com/kpdecker/jsdiff/issues/158) - diffArrays will not compare the empty string in array?
|
||||
- comparator for custom equality checks - 30e141e
|
||||
- count oldLines and newLines when there are conflicts - 53bf384
|
||||
- Fix: diffArrays can compare falsey items - 9e24284
|
||||
- Docs: Replace grunt with npm test - 00e2f94
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.3.1...v3.4.0)
|
||||
|
||||
## v3.3.1 - September 3rd, 2017
|
||||
- [#141](https://github.com/kpdecker/jsdiff/issues/141) - Cannot apply patch because my file delimiter is "/r/n" instead of "/n"
|
||||
- [#192](https://github.com/kpdecker/jsdiff/pull/192) - Fix: Bad merge when adding new files (#189)
|
||||
- correct spelling mistake - 21fa478
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.3.0...v3.3.1)
|
||||
|
||||
## v3.3.0 - July 5th, 2017
|
||||
- [#114](https://github.com/kpdecker/jsdiff/issues/114) - /patch/merge not exported
|
||||
- Gracefully accept invalid newStart in hunks, same as patch(1) does. - d8a3635
|
||||
- Use regex rather than starts/ends with for parsePatch - 6cab62c
|
||||
- Add browser flag - e64f674
|
||||
- refactor: simplified code a bit more - 8f8e0f2
|
||||
- refactor: simplified code a bit - b094a6f
|
||||
- fix: some corrections re ignoreCase option - 3c78fd0
|
||||
- ignoreCase option - 3cbfbb5
|
||||
- Sanitize filename while parsing patches - 2fe8129
|
||||
- Added better installation methods - aced50b
|
||||
- Simple export of functionality - 8690f31
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.2.0...v3.3.0)
|
||||
|
||||
## v3.2.0 - December 26th, 2016
|
||||
- [#156](https://github.com/kpdecker/jsdiff/pull/156) - Add `undefinedReplacement` option to `diffJson` ([@ewnd9](https://api.github.com/users/ewnd9))
|
||||
- [#154](https://github.com/kpdecker/jsdiff/pull/154) - Add `examples` and `images` to `.npmignore`. ([@wtgtybhertgeghgtwtg](https://api.github.com/users/wtgtybhertgeghgtwtg))
|
||||
- [#153](https://github.com/kpdecker/jsdiff/pull/153) - feat(structuredPatch): Pass options to diffLines ([@Kiougar](https://api.github.com/users/Kiougar))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.1.0...v3.2.0)
|
||||
|
||||
## v3.1.0 - November 27th, 2016
|
||||
- [#146](https://github.com/kpdecker/jsdiff/pull/146) - JsDiff.diffArrays to compare arrays ([@wvanderdeijl](https://api.github.com/users/wvanderdeijl))
|
||||
- [#144](https://github.com/kpdecker/jsdiff/pull/144) - Split file using all possible line delimiter instead of hard-coded "/n" and join lines back using the original delimiters ([@soulbeing](https://api.github.com/users/soulbeing))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.0.1...v3.1.0)
|
||||
|
||||
## v3.0.1 - October 9th, 2016
|
||||
- [#139](https://github.com/kpdecker/jsdiff/pull/139) - Make README.md look nicer in npmjs.com ([@takenspc](https://api.github.com/users/takenspc))
|
||||
- [#135](https://github.com/kpdecker/jsdiff/issues/135) - parsePatch combines patches from multiple files into a single IUniDiff when there is no "Index" line ([@ramya-rao-a](https://api.github.com/users/ramya-rao-a))
|
||||
- [#124](https://github.com/kpdecker/jsdiff/issues/124) - IE7/IE8 failure since 2.0.0 ([@boneskull](https://api.github.com/users/boneskull))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v3.0.0...v3.0.1)
|
||||
|
||||
## v3.0.0 - August 23rd, 2016
|
||||
- [#130](https://github.com/kpdecker/jsdiff/pull/130) - Add callback argument to applyPatches `patched` option ([@piranna](https://api.github.com/users/piranna))
|
||||
- [#120](https://github.com/kpdecker/jsdiff/pull/120) - Correctly handle file names containing spaces ([@adius](https://api.github.com/users/adius))
|
||||
- [#119](https://github.com/kpdecker/jsdiff/pull/119) - Do single reflow ([@wifiextender](https://api.github.com/users/wifiextender))
|
||||
- [#117](https://github.com/kpdecker/jsdiff/pull/117) - Make more usable with long strings. ([@abnbgist](https://api.github.com/users/abnbgist))
|
||||
|
||||
Compatibility notes:
|
||||
- applyPatches patch callback now is async and requires the callback be called to continue operation
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.2.3...v3.0.0)
|
||||
|
||||
## v2.2.3 - May 31st, 2016
|
||||
- [#118](https://github.com/kpdecker/jsdiff/pull/118) - Add a fix for applying 0-length destination patches ([@chaaz](https://api.github.com/users/chaaz))
|
||||
- [#115](https://github.com/kpdecker/jsdiff/pull/115) - Fixed grammar in README ([@krizalys](https://api.github.com/users/krizalys))
|
||||
- [#113](https://github.com/kpdecker/jsdiff/pull/113) - fix typo ([@vmazare](https://api.github.com/users/vmazare))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.2.2...v2.2.3)
|
||||
|
||||
## v2.2.2 - March 13th, 2016
|
||||
- [#102](https://github.com/kpdecker/jsdiff/issues/102) - diffJson with dates, returns empty curly braces ([@dr-dimitru](https://api.github.com/users/dr-dimitru))
|
||||
- [#97](https://github.com/kpdecker/jsdiff/issues/97) - Whitespaces & diffWords ([@faiwer](https://api.github.com/users/faiwer))
|
||||
- [#92](https://github.com/kpdecker/jsdiff/pull/92) - Fixes typo in the readme ([@bg451](https://api.github.com/users/bg451))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.2.1...v2.2.2)
|
||||
|
||||
## v2.2.1 - November 12th, 2015
|
||||
- [#89](https://github.com/kpdecker/jsdiff/pull/89) - add in display selector to readme ([@FranDias](https://api.github.com/users/FranDias))
|
||||
- [#88](https://github.com/kpdecker/jsdiff/pull/88) - Split diffs based on file headers instead of 'Index:' metadata ([@piranna](https://api.github.com/users/piranna))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.2.0...v2.2.1)
|
||||
|
||||
## v2.2.0 - October 29th, 2015
|
||||
- [#80](https://github.com/kpdecker/jsdiff/pull/80) - Fix a typo: applyPath -> applyPatch ([@fluxxu](https://api.github.com/users/fluxxu))
|
||||
- [#83](https://github.com/kpdecker/jsdiff/pull/83) - Add basic fuzzy matching to applyPatch ([@piranna](https://github.com/piranna))
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.2.0...v2.2.0)
|
||||
|
||||
## v2.2.0 - October 29th, 2015
|
||||
- [#80](https://github.com/kpdecker/jsdiff/pull/80) - Fix a typo: applyPath -> applyPatch ([@fluxxu](https://api.github.com/users/fluxxu))
|
||||
- [#83](https://github.com/kpdecker/jsdiff/pull/83) - Add basic fuzzy matching to applyPatch ([@piranna](https://github.com/piranna))
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.1.3...v2.2.0)
|
||||
|
||||
## v2.1.3 - September 30th, 2015
|
||||
- [#78](https://github.com/kpdecker/jsdiff/pull/78) - fix: error throwing when apply patch to empty string ([@21paradox](https://api.github.com/users/21paradox))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.1.2...v2.1.3)
|
||||
|
||||
## v2.1.2 - September 23rd, 2015
|
||||
- [#76](https://github.com/kpdecker/jsdiff/issues/76) - diff headers give error ([@piranna](https://api.github.com/users/piranna))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.1.1...v2.1.2)
|
||||
|
||||
## v2.1.1 - September 9th, 2015
|
||||
- [#73](https://github.com/kpdecker/jsdiff/issues/73) - Is applyPatches() exposed in the API? ([@davidparsson](https://api.github.com/users/davidparsson))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.1.0...v2.1.1)
|
||||
|
||||
## v2.1.0 - August 27th, 2015
|
||||
- [#72](https://github.com/kpdecker/jsdiff/issues/72) - Consider using options object API for flag permutations ([@kpdecker](https://api.github.com/users/kpdecker))
|
||||
- [#70](https://github.com/kpdecker/jsdiff/issues/70) - diffWords treats \n at the end as significant whitespace ([@nesQuick](https://api.github.com/users/nesQuick))
|
||||
- [#69](https://github.com/kpdecker/jsdiff/issues/69) - Missing count ([@wfalkwallace](https://api.github.com/users/wfalkwallace))
|
||||
- [#68](https://github.com/kpdecker/jsdiff/issues/68) - diffLines seems broken ([@wfalkwallace](https://api.github.com/users/wfalkwallace))
|
||||
- [#60](https://github.com/kpdecker/jsdiff/issues/60) - Support multiple diff hunks ([@piranna](https://api.github.com/users/piranna))
|
||||
- [#54](https://github.com/kpdecker/jsdiff/issues/54) - Feature Request: 3-way merge ([@mog422](https://api.github.com/users/mog422))
|
||||
- [#42](https://github.com/kpdecker/jsdiff/issues/42) - Fuzz factor for applyPatch ([@stuartpb](https://api.github.com/users/stuartpb))
|
||||
- Move whitespace ignore out of equals method - 542063c
|
||||
- Include source maps in babel output - 7f7ab21
|
||||
- Merge diff/line and diff/patch implementations - 1597705
|
||||
- Drop map utility method - 1ddc939
|
||||
- Documentation for parsePatch and applyPatches - 27c4b77
|
||||
|
||||
Compatibility notes:
|
||||
- The undocumented ignoreWhitespace flag has been removed from the Diff equality check directly. This implementation may be copied to diff utilities if dependencies existed on this functionality.
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.0.2...v2.1.0)
|
||||
|
||||
## v2.0.2 - August 8th, 2015
|
||||
- [#67](https://github.com/kpdecker/jsdiff/issues/67) - cannot require from npm module in node ([@commenthol](https://api.github.com/users/commenthol))
|
||||
- Convert to chai since we don’t support IE8 - a96bbad
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.0.1...v2.0.2)
|
||||
|
||||
## v2.0.1 - August 7th, 2015
|
||||
- Add release build at proper step - 57542fd
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v2.0.0...v2.0.1)
|
||||
|
||||
## v2.0.0 - August 7th, 2015
|
||||
- [#66](https://github.com/kpdecker/jsdiff/issues/66) - Add karma and sauce tests ([@kpdecker](https://api.github.com/users/kpdecker))
|
||||
- [#65](https://github.com/kpdecker/jsdiff/issues/65) - Create component repository for bower ([@kpdecker](https://api.github.com/users/kpdecker))
|
||||
- [#64](https://github.com/kpdecker/jsdiff/issues/64) - Automatically call removeEmpty for all tokenizer calls ([@kpdecker](https://api.github.com/users/kpdecker))
|
||||
- [#62](https://github.com/kpdecker/jsdiff/pull/62) - Allow access to structured object representation of patch data ([@bittrance](https://api.github.com/users/bittrance))
|
||||
- [#61](https://github.com/kpdecker/jsdiff/pull/61) - Use svg instead of png to get better image quality ([@PeterDaveHello](https://api.github.com/users/PeterDaveHello))
|
||||
- [#29](https://github.com/kpdecker/jsdiff/issues/29) - word tokenizer works only for 7 bit ascii ([@plasmagunman](https://api.github.com/users/plasmagunman))
|
||||
|
||||
Compatibility notes:
|
||||
- `this.removeEmpty` is now called automatically for all instances. If this is not desired, this may be overridden on a per instance basis.
|
||||
- The library has been refactored to use some ES6 features. The external APIs should remain the same, but bower projects that directly referenced the repository will now have to point to the [components/jsdiff](https://github.com/components/jsdiff) repository.
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.4.0...v2.0.0)
|
||||
|
||||
## v1.4.0 - May 6th, 2015
|
||||
- [#57](https://github.com/kpdecker/jsdiff/issues/57) - createPatch -> applyPatch failed. ([@mog422](https://api.github.com/users/mog422))
|
||||
- [#56](https://github.com/kpdecker/jsdiff/pull/56) - Two files patch ([@rgeissert](https://api.github.com/users/rgeissert))
|
||||
- [#14](https://github.com/kpdecker/jsdiff/issues/14) - Flip added and removed order? ([@jakesandlund](https://api.github.com/users/jakesandlund))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.3.2...v1.4.0)
|
||||
|
||||
## v1.3.2 - March 30th, 2015
|
||||
- [#53](https://github.com/kpdecker/jsdiff/pull/53) - Updated README.MD with Bower installation instructions ([@ofbriggs](https://api.github.com/users/ofbriggs))
|
||||
- [#49](https://github.com/kpdecker/jsdiff/issues/49) - Cannot read property 'oldlines' of undefined ([@nwtn](https://api.github.com/users/nwtn))
|
||||
- [#44](https://github.com/kpdecker/jsdiff/issues/44) - invalid-meta jsdiff is missing "main" entry in bower.json
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.3.1...v1.3.2)
|
||||
|
||||
## v1.3.1 - March 13th, 2015
|
||||
- [#52](https://github.com/kpdecker/jsdiff/pull/52) - Fix for #51 Wrong result of JsDiff.diffLines ([@felicienfrancois](https://api.github.com/users/felicienfrancois))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.3.0...v1.3.1)
|
||||
|
||||
## v1.3.0 - March 2nd, 2015
|
||||
- [#47](https://github.com/kpdecker/jsdiff/pull/47) - Adding Diff Trimmed Lines ([@JamesGould123](https://api.github.com/users/JamesGould123))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.2.2...v1.3.0)
|
||||
|
||||
## v1.2.2 - January 26th, 2015
|
||||
- [#45](https://github.com/kpdecker/jsdiff/pull/45) - Fix AMD module loading ([@pedrocarrico](https://api.github.com/users/pedrocarrico))
|
||||
- [#43](https://github.com/kpdecker/jsdiff/pull/43) - added a bower file ([@nbrustein](https://api.github.com/users/nbrustein))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.2.1...v1.2.2)
|
||||
|
||||
## v1.2.1 - December 26th, 2014
|
||||
- [#41](https://github.com/kpdecker/jsdiff/pull/41) - change condition of using node export system. ([@ironhee](https://api.github.com/users/ironhee))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.2.0...v1.2.1)
|
||||
|
||||
## v1.2.0 - November 29th, 2014
|
||||
- [#37](https://github.com/kpdecker/jsdiff/pull/37) - Add support for sentences. ([@vmariano](https://api.github.com/users/vmariano))
|
||||
- [#28](https://github.com/kpdecker/jsdiff/pull/28) - Implemented diffJson ([@papandreou](https://api.github.com/users/papandreou))
|
||||
- [#27](https://github.com/kpdecker/jsdiff/issues/27) - Slow to execute over diffs with a large number of changes ([@termi](https://api.github.com/users/termi))
|
||||
- Allow for optional async diffing - 19385b9
|
||||
- Fix diffChars implementation - eaa44ed
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.1.0...v1.2.0)
|
||||
|
||||
## v1.1.0 - November 25th, 2014
|
||||
- [#33](https://github.com/kpdecker/jsdiff/pull/33) - AMD and global exports ([@ovcharik](https://api.github.com/users/ovcharik))
|
||||
- [#32](https://github.com/kpdecker/jsdiff/pull/32) - Add support for component ([@vmariano](https://api.github.com/users/vmariano))
|
||||
- [#31](https://github.com/kpdecker/jsdiff/pull/31) - Don't rely on Array.prototype.map ([@papandreou](https://api.github.com/users/papandreou))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.0.8...v1.1.0)
|
||||
|
||||
## v1.0.8 - December 22nd, 2013
|
||||
- [#24](https://github.com/kpdecker/jsdiff/pull/24) - Handle windows newlines on non windows machines. ([@benogle](https://api.github.com/users/benogle))
|
||||
- [#23](https://github.com/kpdecker/jsdiff/pull/23) - Prettied up the API formatting a little, and added basic node and web examples ([@airportyh](https://api.github.com/users/airportyh))
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.0.7...v1.0.8)
|
||||
|
||||
## v1.0.7 - September 11th, 2013
|
||||
|
||||
- [#22](https://github.com/kpdecker/jsdiff/pull/22) - Added variant of WordDiff that doesn't ignore whitespace differences ([@papandreou](https://api.github.com/users/papandreou)
|
||||
|
||||
- Add 0.10 to travis tests - 243a526
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.0.6...v1.0.7)
|
||||
|
||||
## v1.0.6 - August 30th, 2013
|
||||
|
||||
- [#19](https://github.com/kpdecker/jsdiff/pull/19) - Explicitly define contents of npm package ([@sindresorhus](https://api.github.com/users/sindresorhus)
|
||||
|
||||
[Commits](https://github.com/kpdecker/jsdiff/compare/v1.0.5...v1.0.6)
|
|
@ -0,0 +1,3 @@
|
|||
require('babel-core/register')({
|
||||
ignore: /\/lib\/|\/node_modules\//
|
||||
});
|
File diff suppressed because it is too large
Load Diff
|
@ -0,0 +1,155 @@
|
|||
# Master
|
||||
|
||||
# 4.2.5
|
||||
|
||||
* remove old try/catch performance hacks, modern runtimes do not require these tricks
|
||||
|
||||
# 4.2.4
|
||||
|
||||
* [Fixes #305] Confuse webpack
|
||||
|
||||
# 4.2.3
|
||||
|
||||
* Cleanup testem related build configuration
|
||||
* Use `prepublishOnly` instead of `prepublish` (thanks @rhysd)
|
||||
* Add Node.js 9, 8 to testing matrix
|
||||
* drop now unused s3 deployment files
|
||||
* internal cleanup (thanks to @bekzod, @mariusschulz)
|
||||
* Fixup Changelog
|
||||
|
||||
# 4.2.2
|
||||
|
||||
* Ensure PROMISE_ID works correctly
|
||||
* internal cleanup (thanks yo @mariusschulz)
|
||||
|
||||
# 4.2.1
|
||||
|
||||
* drop bower support
|
||||
|
||||
# 4.2.0
|
||||
|
||||
* drop `dist` from git repo
|
||||
* add `Promise.prototype.finally`
|
||||
* update various build related dependencies
|
||||
* add CDN links
|
||||
|
||||
# 4.1.0
|
||||
|
||||
* [BUGFIX] Fix memory leak [#269]
|
||||
* [BUGFIX] Auto Bundles within an AMD Environment [#263]
|
||||
|
||||
# 4.0.5
|
||||
|
||||
* fix require('es6-promise/auto') for Node < 4
|
||||
|
||||
# 4.0.4
|
||||
|
||||
* fix asap when using https://github.com/Kinvey/titanium-sdk
|
||||
|
||||
# 4.0.3
|
||||
|
||||
* fix Readme links
|
||||
|
||||
# 4.0.2
|
||||
|
||||
* fix require('es6-promise/auto');
|
||||
|
||||
# 4.0.0
|
||||
|
||||
* no longer polyfill automatically, if needed one can still invoke
|
||||
`require('es6-promise/auto')` directly.
|
||||
|
||||
# 3.3.1
|
||||
|
||||
* fix links in readme
|
||||
|
||||
# 3.3.0
|
||||
|
||||
* support polyfil on WebMAF (playstation env)
|
||||
* fix tampering related bug global `constructor` was referenced by mistake.
|
||||
* provide TS Typings
|
||||
* increase compatibliity with sinon.useFakeTimers();
|
||||
* update build tools (use rollup)
|
||||
* directly export promise;
|
||||
|
||||
# 3.2.2
|
||||
|
||||
* IE8: use isArray
|
||||
* update build dependencies
|
||||
|
||||
# 3.2.1
|
||||
|
||||
* fix race tampering issue
|
||||
* use eslint
|
||||
* fix Promise.all tampering
|
||||
* remove unused code
|
||||
* fix issues with NWJS/electron
|
||||
|
||||
# 3.2.0
|
||||
|
||||
* improve tamper resistence of Promise.all Promise.race and
|
||||
Promise.prototype.then (note, this isn't complete, but addresses an exception
|
||||
when used \w core-js, follow up work will address entirely)
|
||||
* remove spec incompatible then chaining fast-path
|
||||
* add eslint
|
||||
* update build deps
|
||||
|
||||
# 3.1.2
|
||||
|
||||
* fix node detection issues with NWJS/electron
|
||||
|
||||
# 3.1.0
|
||||
|
||||
* improve performance of Promise.all when it encounters a non-promise input object input
|
||||
* then/resolve tamper protection
|
||||
* reduce AST size of promise constructor, to facilitate more inlining
|
||||
* Update README.md with details about PhantomJS requirement for running tests
|
||||
* Mangle and compress the minified version
|
||||
|
||||
# 3.0.2
|
||||
|
||||
* correctly bump both bower and package.json versions
|
||||
|
||||
# 3.0.1
|
||||
|
||||
* no longer include dist/test in npm releases
|
||||
|
||||
# 3.0.0
|
||||
|
||||
* use nextTick() instead of setImmediate() to schedule microtasks with node 0.10. Later versions of
|
||||
nodes are not affected as they were already using nextTick(). Note that using nextTick() might
|
||||
trigger a depreciation warning on 0.10 as described at https://github.com/cujojs/when/issues/410.
|
||||
The reason why nextTick() is preferred is that is setImmediate() would schedule a macrotask
|
||||
instead of a microtask and might result in a different scheduling.
|
||||
If needed you can revert to the former behavior as follow:
|
||||
|
||||
var Promise = require('es6-promise').Promise;
|
||||
Promise._setScheduler(setImmediate);
|
||||
|
||||
# 2.3.0
|
||||
|
||||
* #121: Ability to override the internal asap implementation
|
||||
* #120: Use an ascii character for an apostrophe, for source maps
|
||||
|
||||
# 2.2.0
|
||||
|
||||
* #116: Expose asap() and a way to override the scheduling mechanism on Promise
|
||||
* Lock to v0.2.3 of ember-cli
|
||||
|
||||
# 2.1.1
|
||||
|
||||
* Fix #100 via #105: tell browserify to ignore vertx require
|
||||
* Fix #101 via #102: "follow thenable state, not own state"
|
||||
|
||||
# 2.1.0
|
||||
|
||||
* #59: Automatic polyfill. No need to invoke `ES6Promise.polyfill()` anymore.
|
||||
* ... (see the commit log)
|
||||
|
||||
# 2.0.0
|
||||
|
||||
* re-sync with RSVP. Many large performance improvements and bugfixes.
|
||||
|
||||
# 1.0.0
|
||||
|
||||
* first subset of RSVP
|
|
@ -0,0 +1,19 @@
|
|||
Copyright (c) 2014 Yehuda Katz, Tom Dale, Stefan Penner and contributors
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
this software and associated documentation files (the "Software"), to deal in
|
||||
the Software without restriction, including without limitation the rights to
|
||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
|
||||
of the Software, and to permit persons to whom the Software is furnished to do
|
||||
so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,97 @@
|
|||
# ES6-Promise (subset of [rsvp.js](https://github.com/tildeio/rsvp.js)) [](https://travis-ci.org/stefanpenner/es6-promise)
|
||||
|
||||
This is a polyfill of the [ES6 Promise](http://www.ecma-international.org/ecma-262/6.0/#sec-promise-constructor). The implementation is a subset of [rsvp.js](https://github.com/tildeio/rsvp.js) extracted by @jakearchibald, if you're wanting extra features and more debugging options, check out the [full library](https://github.com/tildeio/rsvp.js).
|
||||
|
||||
For API details and how to use promises, see the <a href="http://www.html5rocks.com/en/tutorials/es6/promises/">JavaScript Promises HTML5Rocks article</a>.
|
||||
|
||||
## Downloads
|
||||
|
||||
* [es6-promise 27.86 KB (7.33 KB gzipped)](https://cdn.jsdelivr.net/npm/es6-promise/dist/es6-promise.js)
|
||||
* [es6-promise-auto 27.78 KB (7.3 KB gzipped)](https://cdn.jsdelivr.net/npm/es6-promise/dist/es6-promise.auto.js) - Automatically provides/replaces `Promise` if missing or broken.
|
||||
* [es6-promise-min 6.17 KB (2.4 KB gzipped)](https://cdn.jsdelivr.net/npm/es6-promise/dist/es6-promise.min.js)
|
||||
* [es6-promise-auto-min 6.19 KB (2.4 KB gzipped)](https://cdn.jsdelivr.net/npm/es6-promise/dist/es6-promise.auto.min.js) - Minified version of `es6-promise-auto` above.
|
||||
|
||||
## CDN
|
||||
|
||||
To use via a CDN include this in your html:
|
||||
|
||||
```html
|
||||
<!-- Automatically provides/replaces `Promise` if missing or broken. -->
|
||||
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.js"></script>
|
||||
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.js"></script>
|
||||
|
||||
<!-- Minified version of `es6-promise-auto` below. -->
|
||||
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.min.js"></script>
|
||||
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.min.js"></script>
|
||||
|
||||
```
|
||||
|
||||
## Node.js
|
||||
|
||||
To install:
|
||||
|
||||
```sh
|
||||
yarn add es6-promise
|
||||
```
|
||||
|
||||
or
|
||||
|
||||
```sh
|
||||
npm install es6-promise
|
||||
```
|
||||
|
||||
To use:
|
||||
|
||||
```js
|
||||
var Promise = require('es6-promise').Promise;
|
||||
```
|
||||
|
||||
|
||||
## Usage in IE<9
|
||||
|
||||
`catch` and `finally` are reserved keywords in IE<9, meaning
|
||||
`promise.catch(func)` or `promise.finally(func)` throw a syntax error. To work
|
||||
around this, you can use a string to access the property as shown in the
|
||||
following example.
|
||||
|
||||
However most minifiers will automatically fix this for you, making the
|
||||
resulting code safe for old browsers and production:
|
||||
|
||||
```js
|
||||
promise['catch'](function(err) {
|
||||
// ...
|
||||
});
|
||||
```
|
||||
|
||||
```js
|
||||
promise['finally'](function() {
|
||||
// ...
|
||||
});
|
||||
```
|
||||
|
||||
## Auto-polyfill
|
||||
|
||||
To polyfill the global environment (either in Node or in the browser via CommonJS) use the following code snippet:
|
||||
|
||||
```js
|
||||
require('es6-promise').polyfill();
|
||||
```
|
||||
|
||||
Alternatively
|
||||
|
||||
```js
|
||||
require('es6-promise/auto');
|
||||
```
|
||||
|
||||
Notice that we don't assign the result of `polyfill()` to any variable. The `polyfill()` method will patch the global environment (in this case to the `Promise` name) when called.
|
||||
|
||||
## Building & Testing
|
||||
|
||||
You will need to have PhantomJS installed globally in order to run the tests.
|
||||
|
||||
`npm install -g phantomjs`
|
||||
|
||||
* `npm run build` to build
|
||||
* `npm test` to run tests
|
||||
* `npm start` to run a build watcher, and webserver to test
|
||||
* `npm run test:server` for a testem test runner and watching builder
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in New Issue